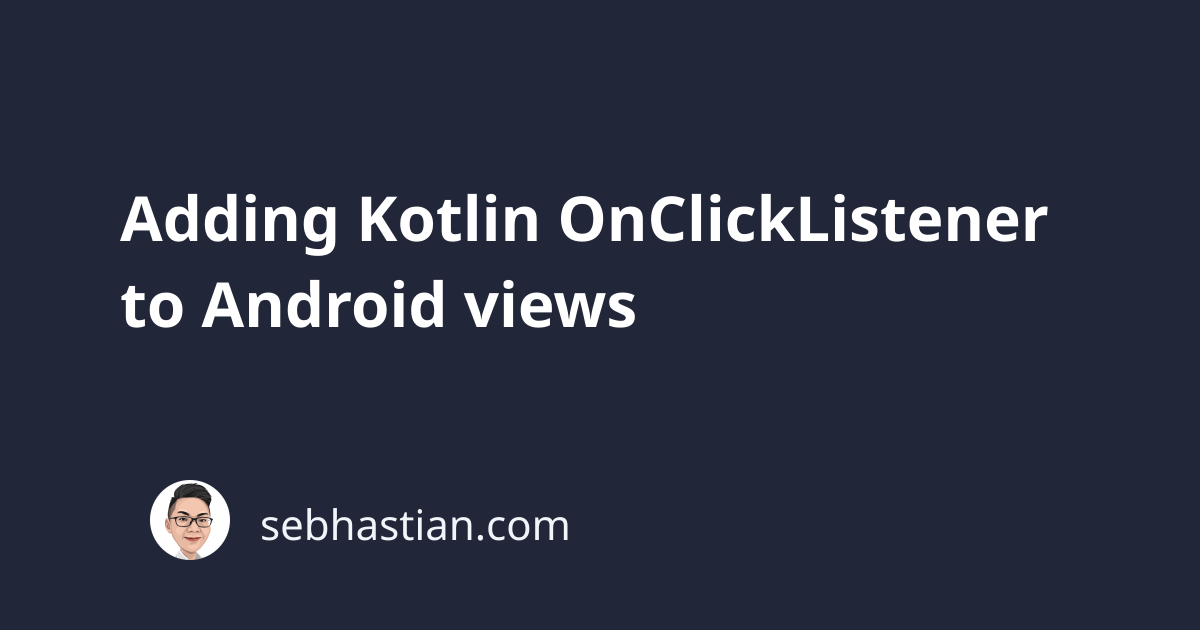
Just like with Java, you can add a listener to your Android View
objects with Kotlin.
To do so, you need to call the setOnClickListener()
function from one of your existing View
instances.
The View
class is the base class used to create all Android UI components like Button
and TextView
, so you can always call the setOnClickListener()
from the UI components added to your XML layout.
For example, here’s how you set the onClickListener
to a Button
with the id
value of button_id
:
val button = findViewById<Button>(R.id.button_id)
button.setOnClickListener {
// do something when the button is clicked
}
In the code block below the setOnClickListener()
function call above, you can write any code you want to execute when the button is clicked.
In Java, you might see the setOnClickListener()
function call where a new View.OnClickListener
instance is created as shown below:
button.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// Code here executes on main thread after user presses button
}
});
In Kotlin, the creation of View.OnClickListener
and the implementation of the onClick
method is done behind the scenes because of Kotlin Java interoperability.
You only need to provide the code to run inside the setOnClickListener
call when using Kotlin.
When you have multiple views with click listeners in your view, you might want to separate the implementation of View.OnClickListener
from the onCreate()
body as follows:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button_id)
val textView = findViewById<TextView>(R.id.textview_id)
button.setOnClickListener(btnListener)
textView.setOnClickListener(textViewListener)
}
val btnListener = View.OnClickListener {
// Button is clicked. Do something
}
val textViewListener = View.OnClickListener {
// TextView is clicked. Do something
}
}
Alternatively, you can also use a single View.OnClickListener
implementation for both views above by checking for the View.id
value before executing your code.
Take a look at the example below:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button_id)
val textView = findViewById<TextView>(R.id.textview_id)
button.setOnClickListener(viewListener)
textView.setOnClickListener(viewListener)
}
private val viewListener = View.OnClickListener {
when (it.id){
R.id.button_id -> Log.i(null, "Button clicked")
R.id.textview_id -> Log.i(null, "TextView clicked")
}
}
}
Inside the View.OnClickListener
block, you can refer to the View
object that’s calling the function using the it
keyword.
With the when
statement, you can create conditional branches that will run only when the id
value of the calling View
matches one of the defined values above.
And those are several ways you can implement the OnClickListener
interface of the View
class using Kotlin.
Unlike in java where everything needs to be explicitly typed, Kotlin has the ability to infer the type of your variables and use a lambda expression as the body of a function call.
You can also separate the implementation of the View.OnClickListener
outside of the setOnClickListener()
method call.
This allows you to listen to multiple View
instances in a concise way.