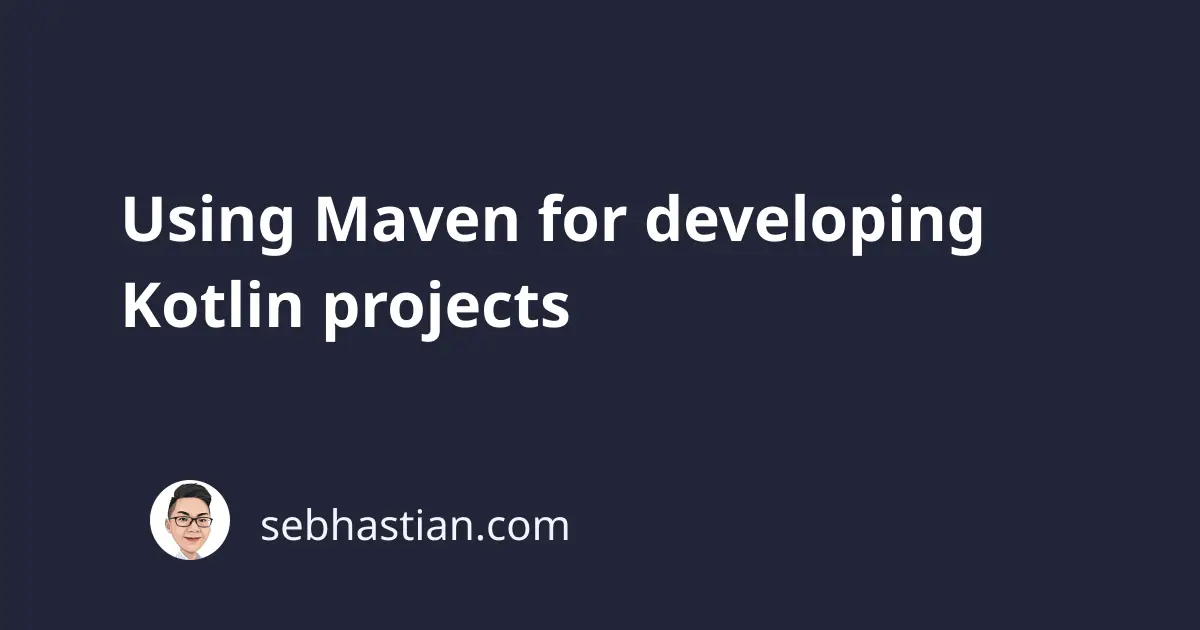
When developing a Kotlin project, you can use Maven as a tool to build your application. Currently, only Maven v3 supports compiling Kotlin code.
The setup of the pom.xml
file in your Kotlin project needs to include the kotlin-maven-plugin
Maven plugin as the dependencies.
This tutorial will help you learn how to compile a Kotlin project using Maven from the command line.
You can see a GitHub repository containing a Kotlin-Maven boilerplate here.
First, make sure that Maven is installed and accessible from the command line by running the mvn -v
command.
Here’s an example output from my computer:
$ mvn -v
Apache Maven 3.8.4 (9b656c72d54e5bacbed989b64718c159fe39b537)
Maven home: /opt/homebrew/Cellar/maven/3.8.4/libexec
Java version: 17.0.1, vendor: Homebrew
OS name: "mac os x", version: "11.5.2", arch: "aarch64", family: "mac"
Once you have Maven installed, create a pom.xml
file and add the necessary Maven project detail like <artifactId>
and <packaging>
.
Also, you need to add the <properties>
tag specifying your Kotlin and Java language version:
<artifactId>KotlinMaven</artifactId>
<groupId>com.kotlin.maven</groupId>
<version>1.0</version>
<packaging>jar</packaging>
<name>KotlinMaven</name>
<properties>
<java.version>1.8</java.version>
<kotlin.version>1.2.51</kotlin.version>
</properties>
Next, you need to add the Kotlin Standard Library as a dependency to your project.
<dependencies>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-stdlib-jdk8</artifactId>
<version>1.5.10</version>
</dependency>
</dependencies>
Once you have the dependency, add the kotlin-maven-plugin
to the <build>
tag inside your pom.xml
file.
Take a look at the following XML section:
<build>
<plugins>
<plugin>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-maven-plugin</artifactId>
<version>1.5.10</version>
<executions>
<execution>
<id>compile</id>
<phase>compile</phase>
<goals>
<goal>compile</goal>
</goals>
<configuration>
<sourceDirs>
<sourceDir>${project.basedir}/src/main/java</sourceDir>
<sourceDir>${project.basedir}/src/main/kotlin</sourceDir>
</sourceDirs>
</configuration>
</execution>
<execution>
<id>test-compile</id>
<phase>test-compile</phase>
<goals>
<goal>test-compile</goal>
</goals>
<configuration>
<sourceDirs>
<sourceDir>${project.basedir}/src/test/java</sourceDir>
<sourceDir>${project.basedir}/src/test/kotlin</sourceDir>
</sourceDirs>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
The kotlin-maven-plugin
specification above allows you to compile both Java and Kotlin source code from the designated <sourceDirs>
section.
With the pom.xml
ready, you need to write a few lines of code to test the build.
First, create new folders and files in your project and make them look as follows:
├── pom.xml
├── src
├── main
├── Main.java
├── java
│ └── JavaClass.java
└── kotlin
└── KotlinClass.kt
Inside the Main.java
file, write a Main
class that generates a different output depending on the argument provided to the main()
function.
The code for the file is as shown below:
public class Main {
static final String JAVA = "java";
static final String KOTLIN = "kotlin";
public static void main(String[] args) {
String language = args[0];
switch (language) {
case JAVA:
new JavaClass().callOutput();
break;
case KOTLIN:
new KotlinClass().callOutput();
break;
default:
System.out.println("Hello World");
break;
}
}
}
The main()
function will call the callOutput()
method from either JavaClass
or KotlinClass
instance depending on the argument you provide when you execute the program.
Next, create a callOutput()
method in the JavaClass.java
file as follows:
public class JavaClass {
public void callOutput() {
System.out.println("This output is from JavaClass");
}
}
Then, write another one inside the KotlinClass.kt
file:
class KotlinClass {
fun callOutput() {
println("This output is from KotlinClass")
}
}
Now that you have the classes available for access, your Kotlin Maven project is now ready to compile.
You need to create a .jar
file from your source code. To do so, run the mvn package
command from your root directory:
$ mvn package
...
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 2.953 s
[INFO] Finished at: 2019-11-24T13:05:10+01:00
[INFO] ------------------------------------------------------------------------
It will take a moment to download the dependencies and compile the jar
file.
Once you see the build success output, a target/
folder should be generated with a KotlinMaven-1.0.jar
file inside.
Maven build failed option no longer supported.
The latest Maven version might trigger an option no longer supported
error when you run the mvn package
command.
Here’s an output that I got when running the command:
[INFO] ------------------------------------------------------------------------
[INFO] BUILD FAILURE
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 0.414 s
[INFO] Finished at: 2022-01-02T15:38:06+07:00
[INFO] ------------------------------------------------------------------------
[ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:3.1:compile
(default-compile) on project KotlinMaven:
Compilation failure: Compilation failure:
[ERROR] Source option 5 is no longer supported. Use 7 or later.
[ERROR] Target option 5 is no longer supported. Use 7 or later.
If you see errors similar to the one highlighted above, that means the JDK version connected to your Maven installation no longer supports Java version 1.5 (or Java 5)
You need to specify the maven.compiler
version in the <properties>
tag of your pom.xml
file. Since Maven says Use 7 or later
, this means you need to target JDK version 1.7 (Java 7) or later.
The <properties>
tag in your pom.xml
file should look as follows:
<properties>
<java.version>1.8</java.version>
<kotlin.version>1.2.51</kotlin.version>
<maven.compiler.source>1.7</maven.compiler.source>
<maven.compiler.target>1.7</maven.compiler.target>
</properties>
Now you should be able to build the project successfully.
Running Maven-built jar using Java
It’s time to run the jar
file using Java. From your terminal, run the following command:
java -cp target/KotlinMaven-1.0.jar src/main/Main.java "kotlin"
The output would be as follows:
This output is from KotlinClass
The above output means that your Main.java
file can access the KotlinClass.kt
file successfully.
You can replace the argument kotlin
with java
to see the output from JavaClass
as shown below:
$ java -cp target/KotlinMaven-1.0.jar src/main/Main.java "java"
This output is from JavaClass
You can download the complete project boilerplate in this GitHub repository.
And that’s how you can build a Kotlin project using Maven.
Although you can build a Kotlin project with Maven just fine, I’d recommend you use Gradle as your build tool.
Both Maven and Gradle are supported by popular Java and Kotlin IDEs like IntelliJ IDEA and Android Studio, but Google chose Gradle as the official build tool for Android.
Furthermore, IntelliJ IDEA can only generate a Gradle-based project for Kotlin Native and Web application projects.
In the screenshot below, you can see that Maven is disabled from the Build System options:
But of course, you are free to use Maven if you want to. 😉