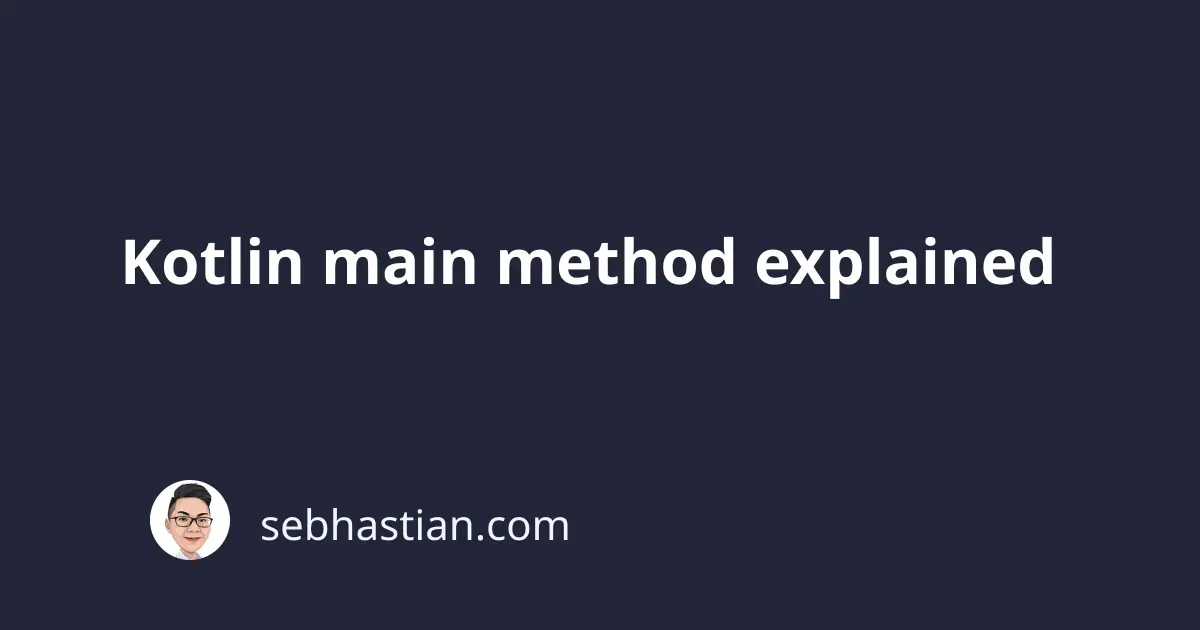
The main()
method is a function that must be included in a Kotlin application.
Like in Java, the main()
method is the entry point of an application written in Kotlin.
A Java application requires you to have a main()
method inside a class
as follows:
class Scratch {
public static void main(String[] args) {
// Your app code here
System.out.println("Hello World");
}
}
But in Kotlin, you don’t need to create a class
for the main()
method.
The main()
method in Kotlin has two constructors: one with the args
argument and one without.
You can write the Kotlin main()
method as follows:
fun main() {
println("Hello World!")
}
// or
fun main(args: Array<String>) {
println("Hello World!")
println(args.contentToString())
}
Without a main()
method, an error will be thrown during runtime, saying Function 'main' not found
.
In Android, the main()
method is replaced with the MainActivity
class that’s generated by Android Studio when you create a new Android project.
An example MainActivity
class might look as follows:
package com.example.kotlinbasic
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Then, the MainActivity
class should be registered in the AndroidManifest.xml
file as the activity to run for MAIN
intent as shown below:
<activity
android:name=".MainActivity"
android:exported="true" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
This is why when developing Android applications, you don’t see the main()
method inside the Kotlin file.
Instead, you have a MainActivity
class with the onCreate()
method.
The MainActivity
class would then be registered in the AndroidManifest.xml
file as the entry point with the <intent-filter>
tag.