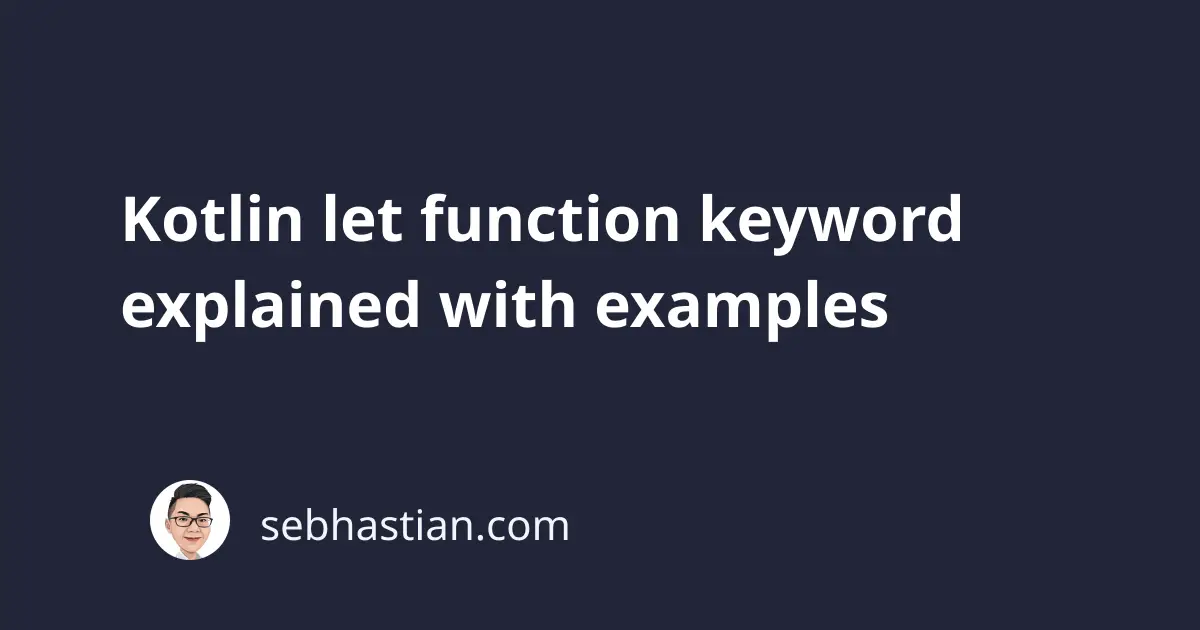
The Kotlin let
keyword is one of several Kotlin scope function keywords.
The scope functions keyword in Kotlin allows you to execute a block of code in the context of the object.
Using scope functions make your code more concise and readable.
For example, suppose you have a User
class with the following definitions:
class User(var username: String, var isActive: Boolean) {
fun hello() {
println("Hello! I'm $username")
}
}
The usual way of instantiating a User
object would be to declare a new variable as shown below:
val user = User("Nathan", true)
user.hello() // Hello! I'm Nathan
user.username = "Jack"
user.hello() // Hello! I'm Jack
By using the let
keyword, you can omit assigning the returned User
object to the variable.
The code example below has the same output as the one above:
User("Nathan", true).let {
it.hello() // Hello! I'm Nathan
it.username = "Jack"
it.hello() // Hello! I'm Jack
}
Instead of having to initialize a new variable, you can call the methods and properties of the generated User
object directly within the let
keyword using a lambda expression.
The object where you call the let
function can be accessed inside let
by using the it
keyword:
User("Nathan", true).let {
println(it.username) // Nathan
println(it.isActive) // true
}
The let
keyword returns the result of the lambda expression you defined inside the code block.
In the above example, the lambda expression returns nothing, so it returns a Unit
type:
val unit = User("Nathan", true).let {
it.hello() // Hello! I'm Nathan
it.username = "Jack"
it.hello() // Hello! I'm Jack
}
println(unit is Unit) // true
To return something other than Unit
, the last code line inside your let
code block must return a value.
For example, you can return the length
value of the username
property:
val usernameLength = User("Nathan", true).let {
it.hello()
it.username = "Jack"
it.username.length
}
println(usernameLength) // 4
if it.username.length
above is not on the last line of the code block, let
would still return a Unit
instead of an Int
value.
Finally, the let
keyword can also be called on any Kotlin built-in objects like a String
or a Boolean
.
This is particularly useful when you have a nullable type variable. You need to perform a safe call with ?.
symbol only once:
val name: String? = "Nathan"
name?.let {
println(it.length) // 6
println(it.lastIndex) // 5
}
Without using let
, you need to add the safe call symbol ?.
before the call to length
and lastIndex
properties as follows:
val name: String? = "Nathan"
println(name?.length)
println(name?.lastIndex)
Now you’ve learned how the let
keyword works in Kotlin. Very nice! 👍