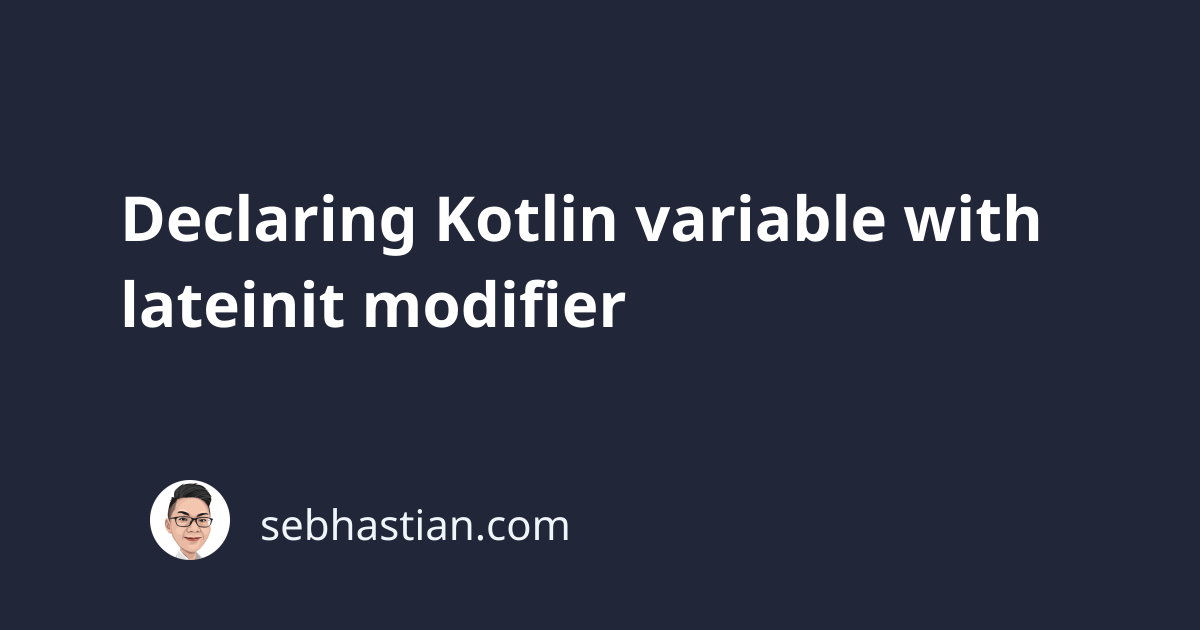
The Kotlin lateinit
modifier is used when you want to declare a non-nullable variable without initializing the value.
To use the modifier, you need to add it before the variable name as follows:
lateinit var message: String
A variable declared with lateinit
must use the var
keyword instead of val
. This is because you need to mutate the value of the variable later.
When you declare a non-nullable variable with Kotlin, you need to immediately set the value on initialization.
For example, if you are defining a String
variable, then the value needs to be assigned during the declaration as follows:
var myString: String = "Hello"
But in developing applications, there will be cases when you want to declare variables without initial values.
It could be that the variable is a class member that gets initialized by one function and called by the other.
Consider the following Message
class:
class Message {
lateinit var message: String
fun setMessage(message: String) {
this.message = message
}
fun getLength(): Int {
return message.length
}
}
As you can see, the message
property of the Message
class is initialized without any value.
The value is set when the setMessage()
function is called.
When you call the getLength()
function first, Kotlin will throw the UninitializedPropertyAccessException
error:
val msg = Message()
msg.getLength() // UninitializedPropertyAccessException
msg.newMessage("Hello!")
To prevent any error with lateinit
variables, you need to check if the variable has been initialized before accessing it.
The getLength()
method needs to check the message
variable initialization using .isInitialized
property:
fun getLength(): Int {
return if(this::message.isInitialized) {
message.length
} else {
0
}
}
Using lateinit modifier in Android apps
The modifier is commonly used when you create an Android application with multiple Activity
classes.
The class variable values might come from the Intent
of another class, so you need to use lateinit
to declare the variables before the onCreate()
method call.
Consider the following example:
class PreviewActivity : AppCompatActivity() {
private lateinit var message: Message
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_preview)
message = intent.getSerializableExtra("Message") as Message
}
}
In the above example, the variable message
is an instance of Message
class.
The variable value was initialized from the extra data put on the intent
by the previous Activity
class.
Without defining the variable as a class member, you won’t be able to use the message
variable outside of the onCreate()
method.
You can also define the variable as a nullable type, but that means you need to set the value as null
on initialization:
private var message: Message? = null
But then each time you need to call the message
property or method, you need to add the double bang operator !!
for non-null asserted call:
message!!.contactName
message!!.getReplyAddress
With the lateinit
modifier, Kotlin won’t complain about the value of your variable and you don’t need to add double bang operator on property and method calls.
Now you’ve learned how the lateinit
modifier works in Kotlin. Nice work! 👍