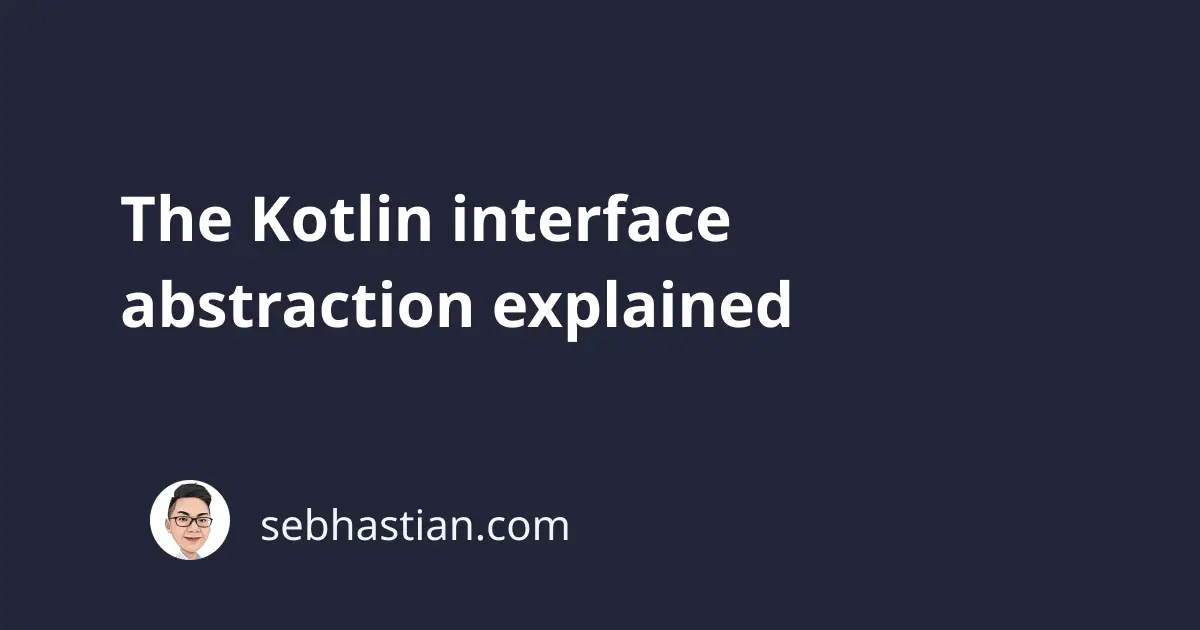
In Kotlin, an interface
is an abstraction type from which you can list specific properties and methods that must be implemented by other classes or interfaces that implement the said interface
.
The goal of using an interface
is to ensure that any class implementing the interface
will have the properties and methods readily available for you to call and use in your program.
An interface
in Kotlin may have the following definitions:
- Abstract methods to be implemented by others
- Implementations of certain methods
- Abstract properties (variables)
- Abstract properties with accessors
Let’s see how you can add these definitions to an interface
next, starting with adding abstract methods.
Kotlin interface abstract methods
First, let’s create an interface
named Animal
in Kotlin as follows:
interface Animal {
fun sleep()
}
In the code above, the function sleep()
is an abstract function that needs to be implemented by any class
that implements the Animal
interface.
Next, let’s create a Dog
class that implements the Animal
interface:
class Dog: Animal
The Dog
class above will cause a static error saying that the class is not abstract and it doesn’t implement the abstract member sleep()
.
In other words, you need to define what the sleep()
method does inside the Dog
class.
The following example should resolve the error:
class Dog: Animal {
override fun sleep() {
println("The dog is sleeping")
}
}
The override
keyword means you are overriding the function that’s inherited from another class or interface.
Kotlin interface method with implementation
Alternatively, an interface
can also define the implementation of specific methods that can be accessed by the implementing class
member.
In the following example, the Animal
interface has implementation of the sleep()
method:
interface Animal {
fun sleep() {
println("The animal is sleeping")
}
}
When an interface
function has its own implementation, you don’t need to override the function inside the implementing class
.
The class
instance can directly call the method as shown below:
class Dog : Animal
val myDog = Dog()
myDog.sleep() // The animal is sleeping
Now you’ve learned how to create abstract and non-abstract methods in an interface
.
Let’s see how to create abstract properties next.
Kotlin interface abstract properties
A Kotlin interface
allows you to create abstract properties that need to be implemented by the class
.
For example, the Animal
interface may have a scientificName
variable that’s abstract as follows:
interface Animal {
val scientificName: String
}
When you implement the Animal
interface to the Dog
class, you need to provide the variable’s value as shown below:
class Dog : Animal {
override val scientificName = "Canidae"
}
This ensures that any class instance you create later will be able to access the scientificName
variable:
val myDog = Dog()
println(myDog.scientificName) // Canidae
You can add as many abstract properties as you need in an interface
type as shown below:
interface Animal {
val scientificName: String
val feet: Int
val canFly: Boolean
}
Kotlin interface with accessors
Alternatively, you can create a property with accessors (set
and get
methods) to modify the behavior of your variable when accessed.
In an interface
, you cannot initialize a property as follows:
interface Animal {
val scientificName: String = "unidentified"
}
To initialize a property in an interface
, you need to use the get()
method as follows:
interface Animal {
val scientificName: String
get() = "unidentified"
}
With the accessors get()
defined in the scientificName
property, you can skip overriding the property in your class
definition.
Take a look at the following example:
interface Animal {
val scientificName: String
get() = "undefined"
}
class Dog : Animal
val myDog = Dog()
println(myDog.scientificName) // undefined
An abstract property in an interface
is commonly defined as a final variable (using the val
keyword)
Because of that, you don’t need to implement a set()
method into the property. The value can’t be changed once initialized.
Implement multiple interfaces with the same properties or methods
Because you can implement multiple interfaces to a single class
, it’s possible that your class may have implemented identical properties or methods from the interfaces.
When your interfaces have the same property name with the same type, then you can override the property just fine as follows:
interface Animal {
val scientificName: String
}
interface Species {
val scientificName: String
}
class Dog : Animal, Species {
override val scientificName: String = "Canidae"
}
But when you have property names with different types from the interfaces, then a conflict will occur:
interface Animal {
val scientificName: String
}
interface Species {
val scientificName: Int
// ^ The same property name with a different type
}
class Dog : Animal, Species {
override val scientificName: String = "Canidae"
}
The imaginary case above shouldn’t happen when you’re developing a Kotlin application.
But when it does happen, then you need to make sure that you’re using the same variable type for the scientificName
properties.
Finally, when you have multiple interfaces with the same method name, then the class
must have its own implementation of the same method.
Consider the following example:
interface Animal {
fun sleep() {
println("ZzZ")
}
}
interface Species {
fun sleep() {
println("Sleeping")
}
}
class Dog : Animal, Species {
override fun sleep() {
println("Good night!")
}
}
val myDog = Dog()
myDog.sleep() // Good night!
The class
implementation of the method tells Kotlin which implementation to execute when the class
instance calls on the method.
And that’s how the interface
type works in Kotlin. It allows you to define properties and methods that a class
implementing the interface
should have.
While you may have multiple interfaces that have the same properties or method names, you need to think through your source code and try to avoid having properties or methods with the same name as best as you can.
Great work on learning about Kotlin interfaces! 😉