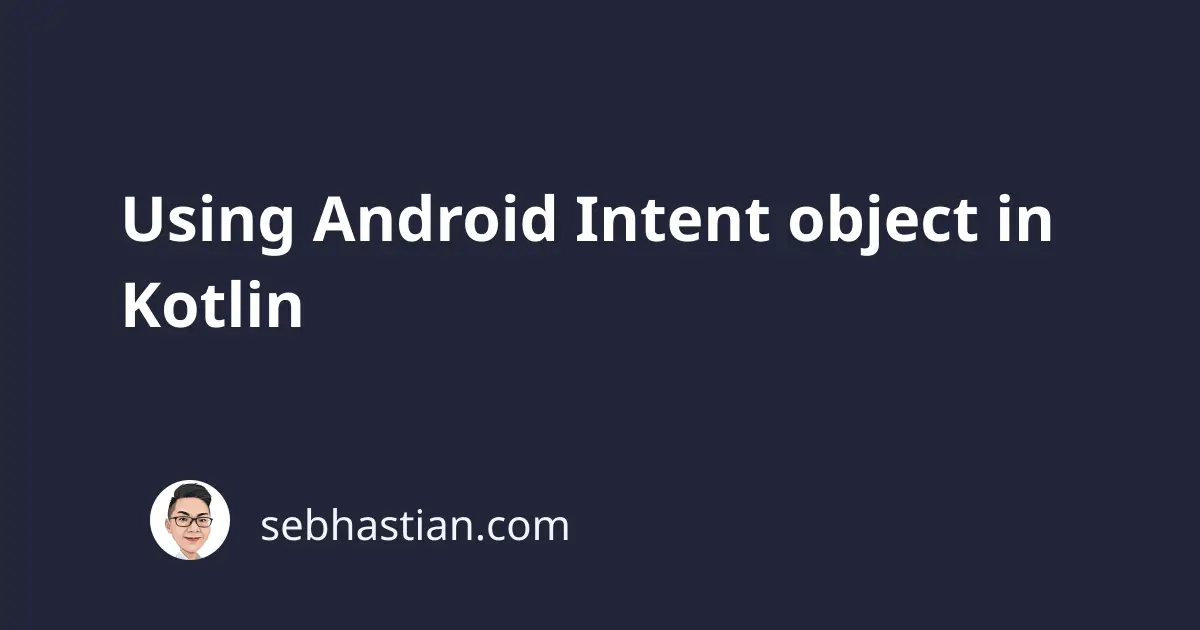
An Intent
is an object of Android application development that’s used for representing an Android application intent to perform an action.
You can use intents for many performing many kinds of actions, such as opening a website or moving from one Android Activity
to the next.
There are two kinds of Intent
in Android:
- Explicit
Intent
is an intent that is fulfilled within the application that runs it. - Implicit
Intent
sends a request to the Android system to fulfill the intended action. It’s up to Android how to respond to the request
This tutorial will help you run both types of Intent
when developing an Android application with Kotlin.
There’s also a companion Android project that demonstrates how to create both types of Intent
in this GitHub repo. You can import the project to Android Studio.
Creating explicit Kotlin Intent
To create an explicit Intent
, you need to pass a Context
and a Class
to the constructor.
For example, the following Kotlin code creates an intent
that takes the current activity this
as the Context
argument and the SecondActivity
class as the Class
argument:
intent = Intent(this, SecondActivity::class.java)
startActivity(intent)
In Kotlin, the SecondActivity
class is directly initialized using the ::class.java
syntax.
Then, the intent
is passed to the startActivity()
function so that a new activity will be launched by Android.
In the example application, you can press the Go to second activity
button to see an explicit Intent
in action:
The code for the explicit intent can be found at the MainActivity.kt
file at lines 14 to 20.
Now that you’ve seen an example of an explicit Intent
, let’s see an example of an implicit Intent
next.
Creating implicit Kotlin Intent
An implicit Intent
can be created by specifying two arguments in the Intent
constructor:
- The
Intent
action that you want Android to perform. There are a limited set of actions provided by Android that you can use - The data to operate on, expressed as a
Uri
value
For example, suppose you want your Android app to open a web browser and navigate to a certain URL.
This is how you can create the Intent
object:
intent = Intent(Intent.ACTION_VIEW, Uri.parse("https://metapx.org"))
startActivity(intent)
The above code states that you want to perform an intended action called ACTION_VIEW
and use the parsed URL address https://metapx.org
as the Uri
data.
The Android system will try to fulfill this request as best as it can.
In the example Android app, you can press the Launch Browser
button to see an implicit Intent
in action:
The code for the implicit intent can be found at the MainActivity.kt
file at lines 22 to 28.
By providing a web address as the Uri
data value, Android will open your default browser and switch the active application to that browser.
The browser will also navigate to the provided URL automatically.
Now you’ve learned how to create an Android Intent
object in Kotlin. Good work! 👍