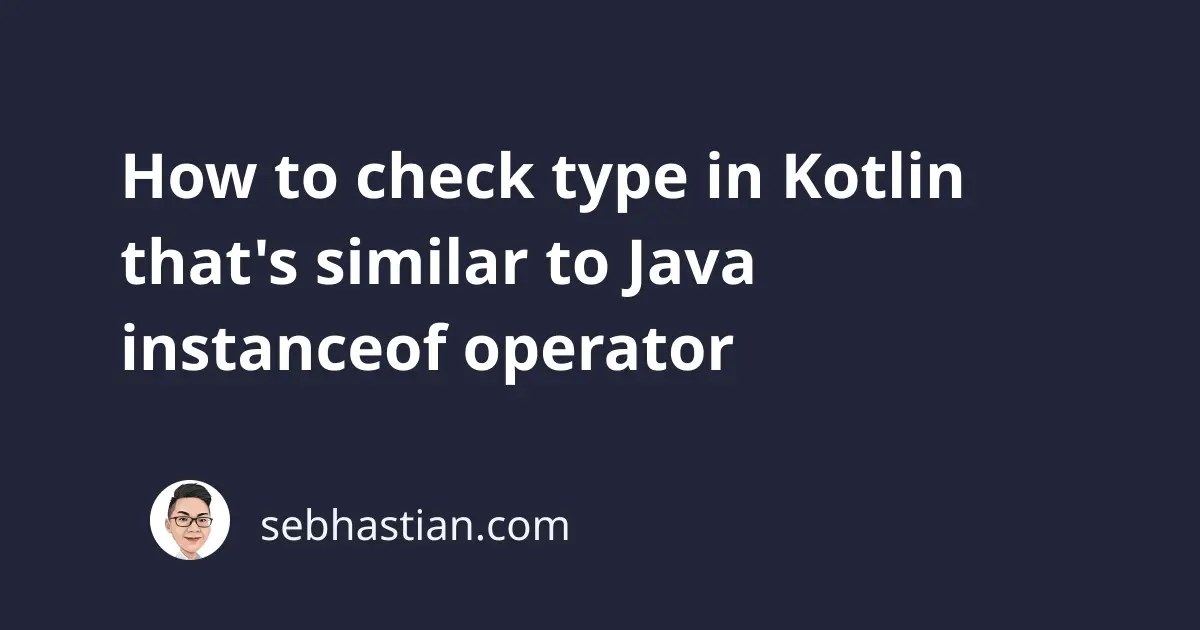
In Java, the instanceof
operator is commonly used to check if an Object
variable is of a certain type.
You may write code as follows in Java:
class Scratch {
public static void main(String[] args) {
Object myVar = "Hello World";
checkType(myVar);
}
private static void checkType(Object myVar) {
if (myVar instanceof Boolean) {
System.out.println("myVar is a Boolean object");
}
}
}
But Kotlin doesn’t have the instanceof
operator.
Instead, it allows you to check on an Object
type using the is
and !is
operators.
Kotlin is and !is operator explained
The is
operator allows you to check on a variable type just like instanceof
operator.
Here’s an example code in Kotlin:
var myVar: Any = "Hello"
if (myVar is Boolean){
print("myVar is a Boolean object")
}
Just like in Java, the is
operator can also check if the Any
object variable you have is of a custom class that you defined in your Kotlin source code.
In the example below, I can check if the variable is of a Dog
class type that I have defined previously:
class Dog {
fun bark(){
print("Woof!")
}
}
var myVar: Any = Dog()
if (myVar is Dog){
print("myVar is a Dog object")
}
Finally, the !is
operator is used to check whether an object is not of a certain type:
var myVar: Any = false
if (myVar !is String){
print("myVar is a not a String object")
}
// similar to
if !(myVar is String){
print("myVar is a not a String object")
}
The !is
operator is similar to adding an exclamation mark in an is
operator check.
You’ve just learned the Kotlin equivalent to the instanceof
operator. Well done! 👍