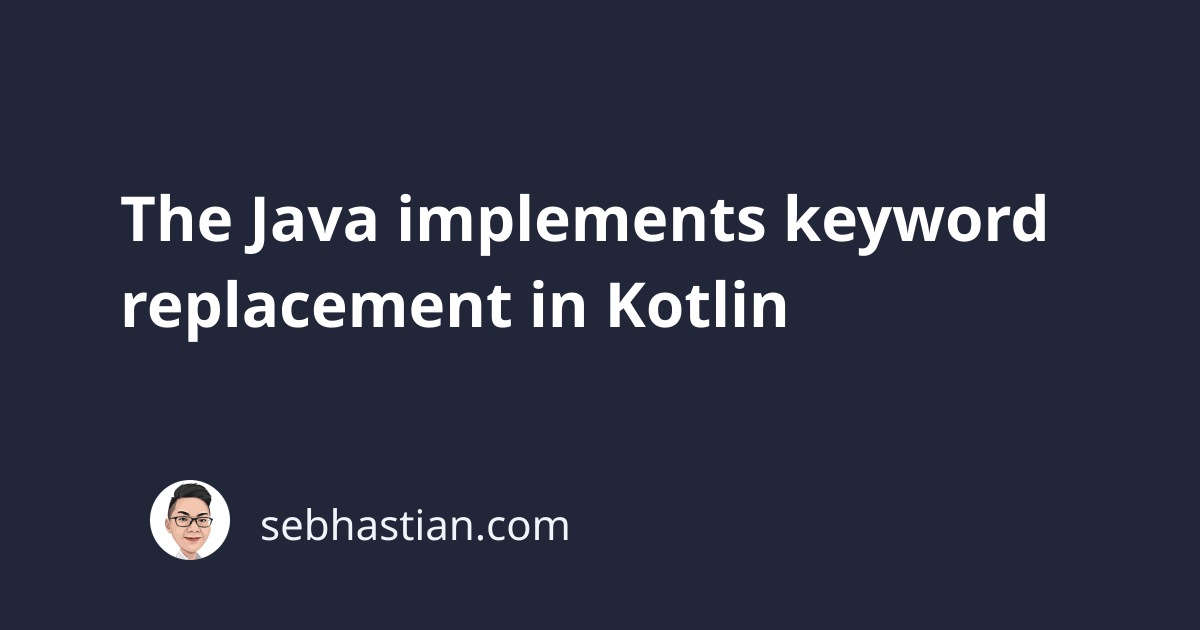
In Java, you need to use the implements
keyword when you’re implementing an interface or extends
when extending an existing class.
The following example code shows how to implement the Animal
interface to the Main
class:
interface Animal {
static void sleep(){
System.out.println("ZzzZ");
}
}
class Dog implements Animal {
public static void main(String[] args) {
Animal.sleep();
}
}
And the following code shows how to extend the Vehicle
class on the Car
class:
class Vehicle {
static void start(){
System.out.println("Igniting the engine");
}
}
class Car extends Vehicle {
public static void main(String[] args) {
start();
}
}
In Kotlin, both implements
and extends
keywords are replaced with a colon :
syntax. The symbol will mark an interface implementation or a class extension.
Here’s how you implement an interface with Kotlin:
interface Animal {
fun sleep() = println("ZzzZ")
}
class Dog: Animal
val myDog = Dog()
myDog.sleep() // ZzzZ
And here’s how you extend a class with Kotlin:
open class Vehicle {
fun start() = println("Igniting the engine")
}
class Car: Vehicle()
val myCar = Car()
myCar.start()
All classes in Kotlin are final
by default, so you need to add the open
modifier to allow class extensions in Kotlin.
But whether you need to implement an interface or extend a class, you only need to use the :
syntax after the class name to do so.
When you want to:
- implement multiple interfaces
- or an interface and a class on a single class
You can list all the interfaces and classes after the colon, separated by a comma as shown below:
open class Vehicle {
fun start() = println("Igniting the engine")
}
interface Animal {
fun sleep() = println("ZzzZ")
}
class Car : Vehicle(), Animal
val myCar = Car()
myCar.start()
myCar.sleep()
And that will be all on Java implements
replacement in Kotlin.