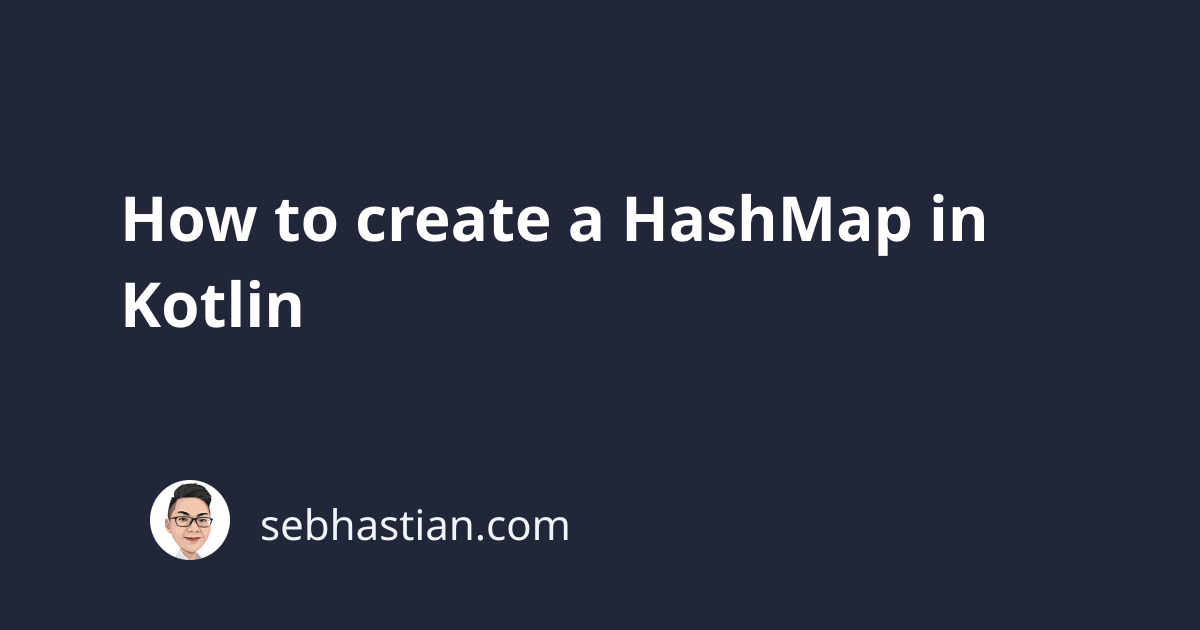
A HashMap
is a data structure that contains a collection of key-value pairs.
Each value you put into a HashMap
can be retrieved from the key you define for that value.
To create a HashMap
in Kotlin, you can call the HashMap<>()
or hashMapOf<>()
functions.
Here’s the call syntax for the functions:
HashMap<K, V>()
hashMapOf<K, V>()
The generic K
is the type for the key, while V
is the generic type for the value in your HashMap
Both functions will create a new empty HashMap
that you can put key-value pairs into.
For example, here’s how you create a HashMap
with a String
key and Int
value:
val firstMap = HashMap<String, Int>()
val secondMap = hashMapOf<String, Int>()
The code above creates two HashMap
of K = String
and V = Int
.
To insert new data into the HashMap
above, you can use the assignment syntax or the put()
method as shown below:
firstMap["A"] = 1
firstMap["B"] = 2
firstMap["C"] = 3
// or
firstMap.put("A", 1)
firstMap.put("B", 2)
firstMap.put("C", 3)
The above syntax produces the same result. You can use all the properties and methods of the HashMap
class to help you manipulate the HashMap
instance that you’ve created.
HashMap
properties like entries
, keys
, and values
help you to grab the content of your hashmap instance:
println(firstMap.entries) // [A=1, B=2, C=3]
println(firstMap.keys) // [A, B, C]
println(firstMap.values) // [1, 2, 3]
You can use the HashMap
method clear()
to empty the instance:
firstMap.clear()
println(firstMap.entries) // []
Finally, you can loop over the HashMap
to and print each key-value pair as follows:
for (key in firstMap.keys) {
println("Element at key $key : ${firstMap[key]}")
}
The result of the for
statement above is as shown below:
Element at key A : 1
Element at key B : 2
Element at key C : 3
And that’s how you can create a HashMap
instance in Kotlin.
For more information on the methods available for Kotlin HashMap
, please refer to the Kotlin documentation.
Now you’ve learned how Kotlin HashMap
works. Great work! 😉