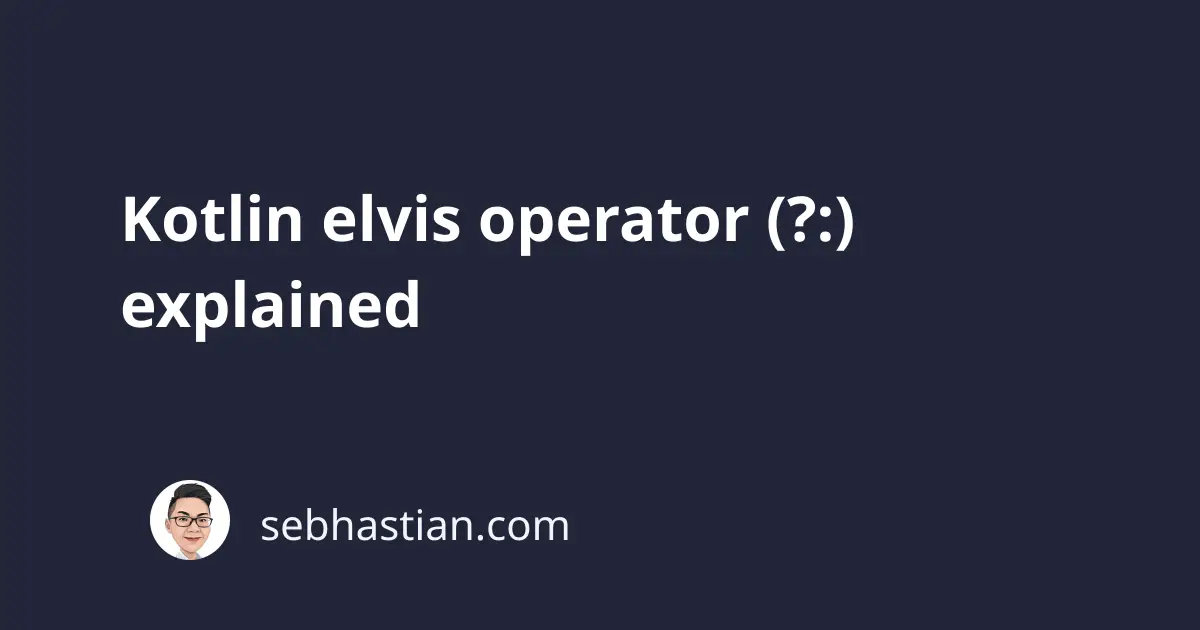
The Kotlin elvis operator ?:
is commonly used to write a shorter conditional assignment when you try to assign the value of a nullable variable into a non-nullable variable.
For example, suppose you have a nullable string called myStr
that you wish to assign to another variable that’s a non-nullable type:
var myStr: String? = null
var myVar: String = myStr // Type mismatch error
Kotlin considers the String?
and String
as different types, so you will get a type mismatch error during static checking of the code.
To be able to assign myStr
value to myVar
, you need to let Kotlin know what alternative value will be assigned to the myVar
variable if the myStr
variable value is null
.
To do so, you need to make use of the elvis operator as follows:
var myStr: String? = null
var myVar: String = myStr ?: "Hello"
The elvis operator above will cause Kotlin to check on the value of myStr
variable during myVar
initialization.
If myStr
value is not null
, then Kotlin will assign the value of myStr
to myVar
as normal.
If the myStr
value is null
, then the value on the right side of the operator will be returned to myVar
instead.
And that’s how the elvis operator works in Kotlin. It allows you to provide an alternative value to use when the left side operand value is null
.
Additionally, you can also combine the elvis operator with the null checking operator ?.
to write a short and concise code.
For example, suppose you want to print the length of myStr
. You can check if the variable is null
and provide an alternative string to print when it is:
var myStr: String? = null
print(myStr?.length ?: "The string is null")
Without the null check and the elvis operator, you need to write the code above using the if..else
conditional as follows:
var myStr: String? = null
print(if (myStr != null) myStr!!.length else "The string is null")
I don’t know about you, but the if..else
statement seems confusing to read for me 😵💫
Furthermore, you also need to add the double bang operator !!
to tell Kotlin that you are sure myStr
value is not null
when accessing the .length
property.
By using the elvis operator, the code you write will be easier to read and understood by other developers.