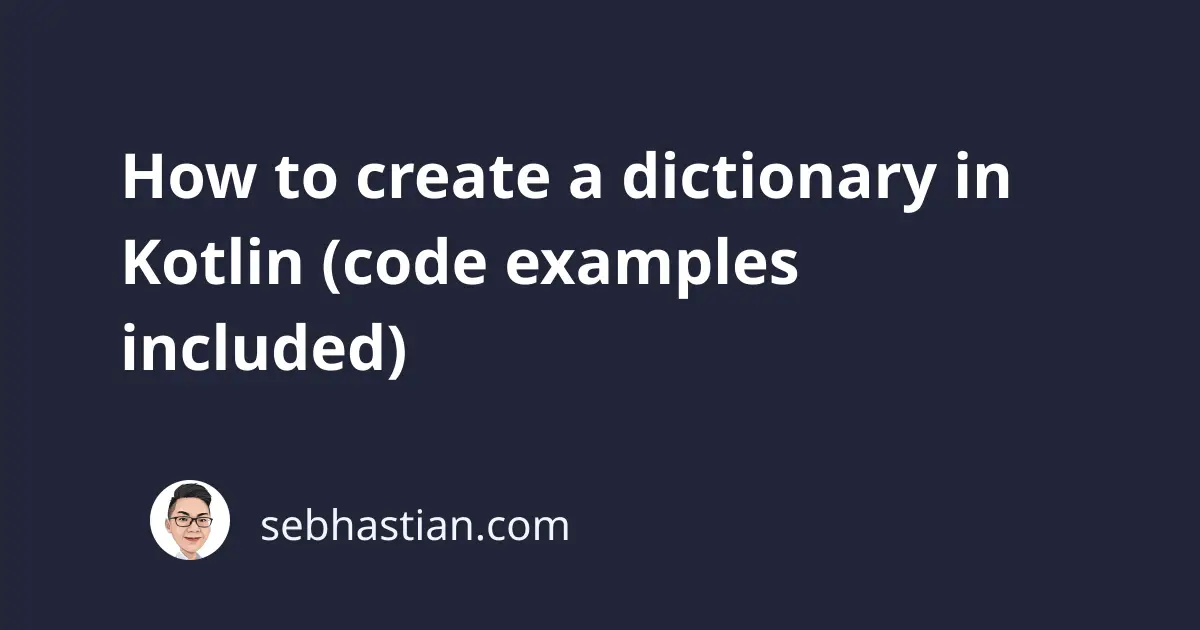
In Kotlin, you can create a dictionary by using the Map
class as the type of your variable.
To create an instance of the Map
class, you can call the mapOf()
function and pass the key-value pair as its argument.
Here’s an example of creating a dictionary with String
type for the keys and Int
type for the values:
val dictionary = mapOf("key" to 1, "anotherKey" to 2)
println(dictionary) // {key=1, anotherKey=2}
You can get the individual value of the dictionary by specifying the key in the index operator.
For example, to grab the value of key
:
print(dictionary["key"]) // 1
Creating a mutable map
The Map
class is immutable. That means you can’t modify or add more key-value pairs after the initialization.
In the following example, assigning another value to an existing key and adding a new key-value pair triggers an error:
val dictionary = mapOf("key" to 1, "anotherKey" to 2)
dictionary["key"] = 20 // Error: not set method providing array access
dictionary["max"] = 99 // Error: not set method providing array access
To create a mutable dictionary, you need to use the MutableMap
class as the blueprint of your dictionary.
The MutableMap
instance can be created by calling the mutableMapOf()
function.
It accepts the same key-value argument(s) as the mapOf()
function:
val mutableDict = mutableMapOf("key" to 1, "anotherKey" to 2)
mutableDict["key"] = 20
mutableDict["max"] = 99
println(mutableDict) // {key=20, anotherKey=2, max=99}
The MutableMap
class extends the Map
class and adds the write operation method to it.
The MutableMap
class also has two subclasses:
- The
LinkedHashMap
class preserves the insertion order of the key-value pairs - The
HashMap
class doesn’t guarantee the insertion order of the key-value pairs
The LinkedHashMap
instance can be created using the linkedMapOf()
function, while the HashMap
instance can be created using hashMapOf()
function.
Here’s an example of creating a HashMap
dictionary:
val mutableDict = hashMapOf("key" to 1, "anotherKey" to 2)
mutableDict["key"] = 20
mutableDict["max"] = 99
// 👇 The order of the pairs are random
print(mutableDict) // {anotherKey=2, max=99, key=20}
Now you’ve learned how to create a dictionary using Kotlin. Well done! 👍