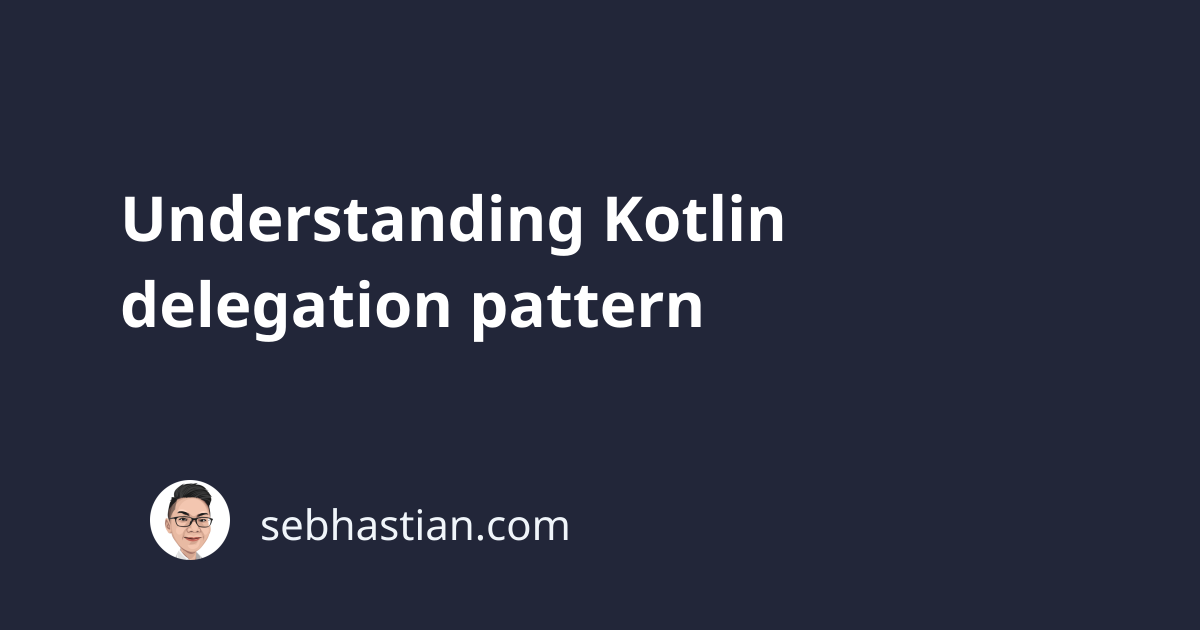
The delegation pattern is a design pattern in object-oriented programming that allows you to reuse code like the inheritance pattern.
In the delegation pattern, an object performs a specific task (running a method) by delegating it to another object, usually known as the helper object.
Below is an example of the delegation pattern implementation in Kotlin:
class Delegate(){
fun print() {
print("Hello World from Delegate!")
}
}
class Main(val d: Delegate){
fun print() = d.print()
}
In the above example, the Main
class delegates the print()
function definition into the Delegate
class implementation of the same method.
You can call the Main.print()
function with the following code:
Main(Delegate()).print()
// Hello World from Delegate!
In Kotlin, you can even delegate to an interface
instead of a class
to extend the code reuse ability of the delegation pattern.
This is done by using the by
keyword which delegates the interface
implementation to an object you pass to the class
.
Consider the following example:
interface Delegate {
fun print()
}
class Main(val d: Delegate) : Delegate by d
The Main
class above offer functions defined in the Delegate
interface that are implemented through the d
object.
Since the d
object is of type Delegate
, any implementation
of the interface
will be a valid constructor parameter.
In the example below, both DelegateImpl
class and DelegateNumber
class can be passed into the Main
class:
class DelegateImpl() : Delegate{
override fun print() {
print("DelegateImpl!")
}
}
class DelegateNum() : Delegate{
override fun print() {
print("DelegateNum!")
}
}
class Master(val d: Delegate) : Delegate by d
Master(DelegateImpl()).print() // DelegateImpl!
Master(DelegateNum()).print() // DelegateNum!
Kotlin allows you to delegate to an interface
using the by
keyword so that you don’t need to use a specific class
implementation.
The delegation pattern helps you to reuse code by passing an object to a class. It’s also useful to implement interfaces using their existing class implementations as shown above.
And that’s how the delegation pattern works in Kotlin 😉