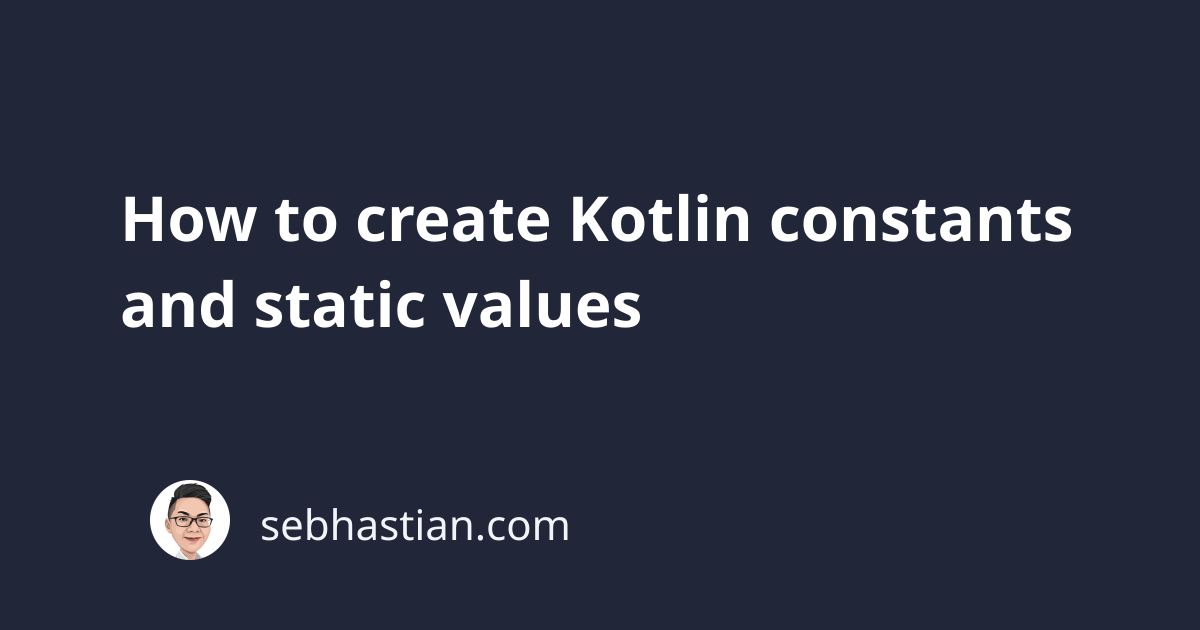
In Kotlin, you can use the var
and val
keyword to declare and initialize variables.
Variables created using the var
keyword can be changed, which means they are mutable.
To create constant (immutable) values in Kotlin, you need to use the val
keyword when declaring the variable:
val statusGreen = "green"
statusGreen = "red" // Error: Val cannot be reassigned
To create a static variable that’s known at compile-time, you can add the const
keyword before the val
keyword.
The const
keyword is equivalent to the use of static
keyword in Java:
const val STATUS_GREEN = "green"
When initializing a const val
variable, you are encouraged to define the variable name using all uppercase with underscores to separate the words. This is to follow the Java convention when declaring a static constant variable.
But you can still use the camelCase naming if you want to.
Finally, you can only create a const val
variable on top-level or in objects:
package com.example.myapplication
const val STATUS_GREEN = "green" // OK
class Helper {
const val STATUS_RED = "red" // Error
}
object Constants {
const val STATUS_YELLOW = "yellow" // OK
}
To declare a const val
variable inside a class
, you need to add a companion object
to your Kotlin class
.
Take a look at the following example:
class Helper {
companion object {
const val STATUS_RED = "red" // OK
}
}
The companion object
keyword creates an object with the same name as the class
. It’s the Kotlin way of accessing members of a class
without instantiating the class
itself.
Since you can create a single object using the object
keyword in Kotlin, it’s recommended to create an object
instead of a class
when you want to group constant variables.
Consider the following example:
object Status {
const val GREEN = "green"
const val YELLOW = "yellow"
const val RED = "red"
}
You can then call the constants inside the object
like how you call the Math.PI
constant:
println(Status.GREEN) // green
println(Status.YELLOW) // yellow
println(Status.RED) // red
A Kotlin object
represents a static instance that can’t be instantiated (a singleton) which is perfect for storing static constant values.
When you need to create an object that can’t be instantiated, use the object
keyword instead of class
.
If you must use a class
for a specific reason, then you need to add a companion object
to the class for declaring constant members.
And that’s how you define constant values in Kotlin 😉