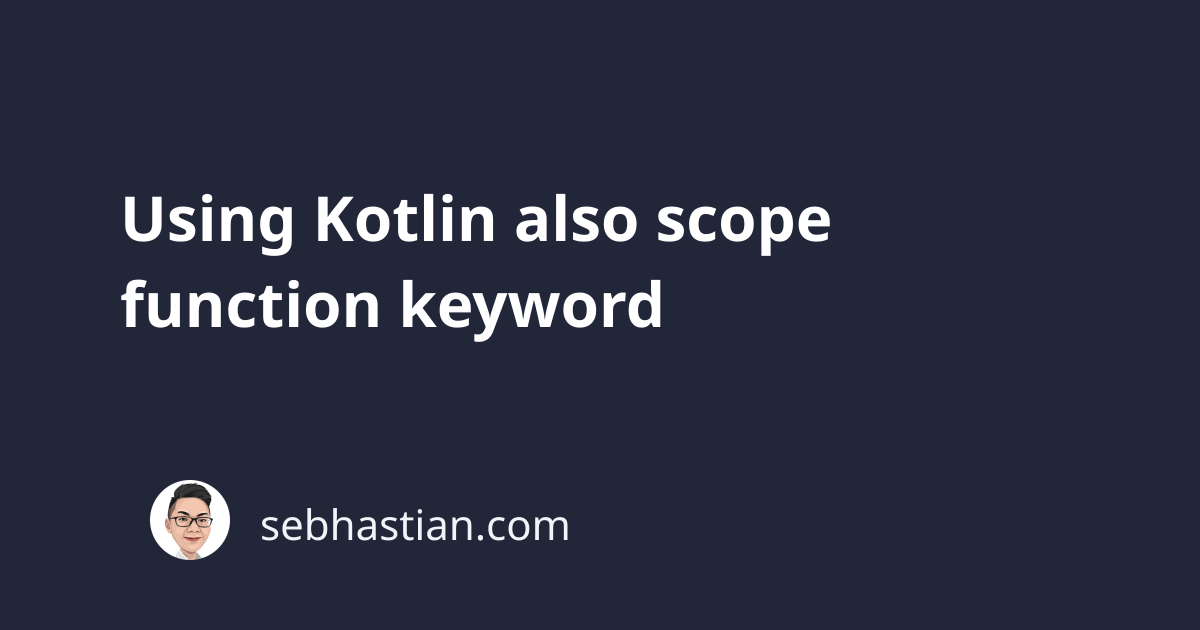
The Kotlin also
keyword is one of the keywords that allows you to create a scope function.
A scope function in Kotlin is used to execute a block of code under the context of the object from which you call the function.
Using a scope function allows you to write more concise and readable code.
Let’s see the also
scope function in action.
Suppose you have an array of String
as follows:
val myArray = arrayOf("One", "Two", "Three")
To change the values of the array in a certain index, you would use the indexing operator assignment [index]
as shown below:
myArray[0] = "Four"
myArray[1] = "Five"
println(myArray.joinToString()) // Four, Five, Three
Using the also
operator, you can change the assignment as follows:
myArray.also {
it[0] = "Four"
it[1] = "Five"
println(it.joinToString()) // Four, Five, Three
}
The also
keyword is called using the Kotlin lambda expression as its body.
Inside the scoped function body, you can refer to the receiver object using the it
keyword.
As you see in the example above, you can do things with the object inside the also
function body.
The also
keyword returns the receiving object, which you can save under a variable:
val newArr = myArray.also {
it[0] = "Four"
it[1] = "Five"
}
newArr.joinToString() // Four, Five, Three
But most of the time the returned object will be identical to the one you use to call the also
function, so you can omit saving it as a variable.
You can use the also
function in any valid Kotlin object. The example below calls the also
function from a String
instance:
val str = "Hello"
str.also {
if (it.length > 3) {
println("String length greater than 3")
} else {
println("String length less than 3")
}
}
And that’s how you use the also
scoped function in Kotlin 😉