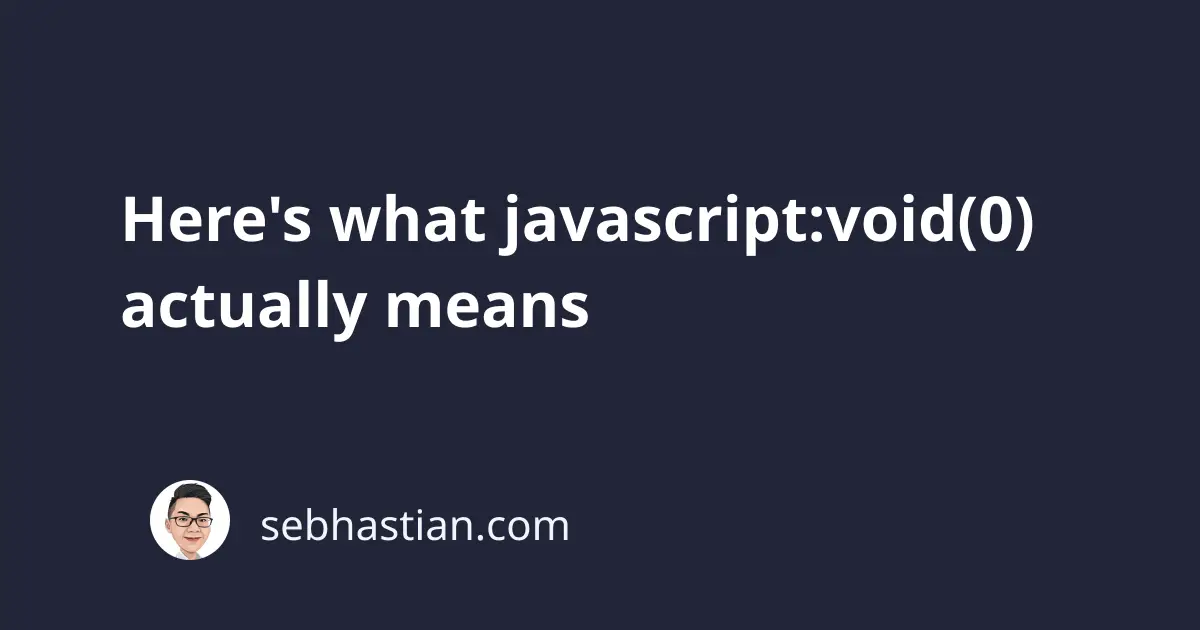
The JavaScript void
keyword is an operator that tells JavaScript NOT to return the evaluation result of an expression.
For example, the following expression between 2 + 2
will return 4
;
let num = 2 + 2;
console.log(num); // 4
When you evaluate the expression using the void
keyword, the expression will return undefined
instead of the actual result:
let num = void (2 + 2);
console.log(num); // undefined
Even though the num
keyword is undefined
, the 2 + 2
expression above is still executed by JavaScript:
let num = void console.log(2 + 2); // 4
console.log(num); // undefined
In short, the code that follows the void
operator will still be executed as normal JavaScript code, but it will always return undefined
.
What javascript:void(0); actually means
As a web developer, you may sometimes find javascript:void(0)
written as the value of href
attribute for some <a>
tag.
For example, you might find a button to print the web page written as follows:
<a href="javascript:void(0)" onclick="printPage()" id="printButton">
Print
</a>
The javascript:
part is a URI scheme that tells your browser to treat the following text as a JavaScript code. There are other URI for href
attribute like tel:
for phone numbers and sms:
to launch the SMS application of your phone.
The 0
inside the void
operator is simply used as a placeholder. By using javascript:void(0)
, the <a>
tag won’t send you to other web address. It also won’t refresh the page as links usually do when you put /
as the value of href
attribute.
You may wonder then why not just remove the href
attribute? Well, removing the href
attribute will cause the pointing finger mouse icon to turn into a caret for text.
In short, putting javascript:void(0)
as the value of href
attribute will create a link that does nothing.
The example link above will run the printPage()
function when clicked without refreshing the page or sending you anywhere.
When do you need to use void?
The void
operator is rarely used today because of the improvements made in JavaScript ES versions.
For example, you can re-assign the undefined
keyword as another value:
undefined = 9;
console.log(9 === undefined); // false
The example above will return true
before the ES5 update in 2009, so you want to check for undefined with void 0
instead:
console.log(void 0); // undefined
console.log(9 === void 0); // false
But undefined
is read-only after ES5, so you don’t need void 0
anymore.
As for preventing the default behavior of <a>
tag, it’s generally encouraged for you to use <button>
for buttons and <a>
for links.
When you need to change the cursor, it’s recommended to use CSS instead of using the <a>
tag.