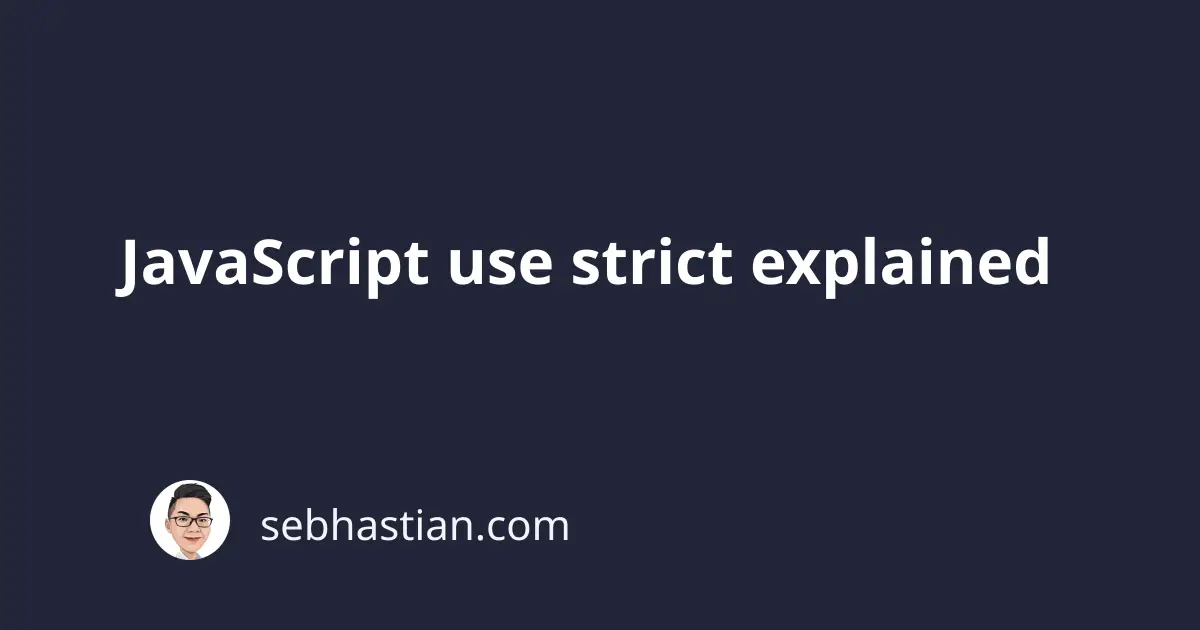
The "use strict"
directive is added since ES5 in order to help you write JavaScript code that is less prone to error. For example, you can’t initialize a variable that’s not yet defined with the strict mode active:
"use strict";
name = "Jack";
To active the strict mode you need to add the "use strict"
string expression right at the first line of your code. Adding the directive may be ignored by JavaScript:
console.log("Hello");
("use strict"); // ignored
name = "Jack"; // doesn't throw an error
Is use strict necessary?
The strict mode is no longer required since the release of ES2015, which fixes most of JavaScript’s confusing behavior with a more robust syntax. It’s just good to know because you might find it in legacy projects that still used it.
Strict mode rules
The strict mode will prohibit you from doing the following things:
You can’t use a variable before declaration:
"use strict";
name = "Jack"; // ReferenceError: name is not defined
Deleting declarations and expressions including variables, functions, and classes:
"use strict";
function greeting() {
console.log("Hello");
}
delete greeting; // Delete of an unqualified identifier in strict mode
Deleting an undeletable property:
"use strict";
delete Object.prototype; // Cannot delete property 'prototype' of function Object()
Using the same parameter name twice, like arg
name above:
"use strict";
function x(arg, arg) {} // Duplicate parameter name not allowed in this context
Removed the with
statement because it causes performance and ambiguity problems.
"use strict";
with ({}) { // Strict mode code may not include a with statement
var name = "Jack";
}
Octal literals are not allowed:
"use strict";
var num = 010; // Octal literals are not allowed in strict mode.
You can’t use some names for variables:
"use strict";
var eval = 7; // Unexpected eval or arguments in strict mode
The list of restricted names are:
eval
arguments
implements
interface
let
package
private
protected
public
static
yield
And it will include any names that will be used as keywords for future JavaScript versions.