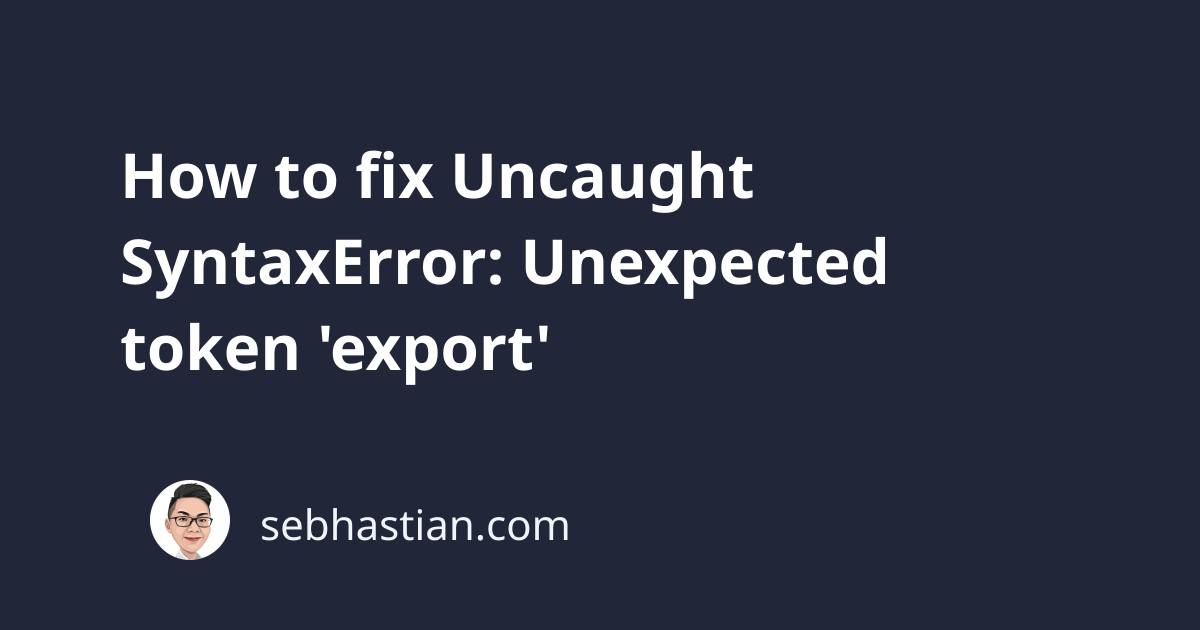
The error “Unexpected token ’export’” occurs when you run JavaScript code in an environment that doesn’t support the export
keyword.
The export
keyword is a part of JavaScript specification that allows you to export a module so that other modules can use that module using the import
keyword.
This keyword is a part of ECMAScript Modules (ESM) specification, which is the official standard way to reuse JavaScript code.
Suppose you run the following code from the browser:
function sum(x, y) {
return x + y;
}
export { sum };
The browser would respond with the following error:
Uncaught SyntaxError: Unexpected token 'export'
To use the export/import
keyword, you need to activate the ESM specification. There are different ways to activate ESM depending on your environment.
1. Activate ESM support in the browser
To activate ESM support in the browser, you need to specify the type="module"
attribute in the <script>
tag.
Here’s an example:
<script type="module" src="util.js"></script>
Then inside the util.js
file, you can write JavaScript code that uses the export
keyword:
// util.js
function sum(x, y) {
return x + y;
}
export { sum };
To import the function, you can create another <script>
tag that also has the type="module"
attribute:
<script type="module">
import { sum } from "./util.js";
console.log(sum(1, 5));
</script>
When exporting a module, it’s recommended to put the module inside its own .js
file so that you can import it without any errors.
If you put the exported module inline like this:
<script type="module">
function sum(x, y) {
return x + y;
}
export { sum };
</script>
Then importing the module would require a hack because it’s not supported natively.
To make things easy and maintainable, always export JavaScript code from an external script.
2. Activate ESM support in Node.js
To enable ESM in Node.js, you need to set the type
attribute to module
or change the file extension to .mjs
.
Open your package.json
file and add the type
attribute as follows:
{
"name": "n-app",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module"
}
If you don’t have a package.json
file in your project, you can create one using the npm init
command:
npm init -y
Once created, add the type
attribute as shown above, and you should be able to run JavaScript code using the export
keyword.
If that doesn’t work, you need to check on the Node version you have on the computer.
Node.js support for ESM is available starting from v14.13.0 and above. If you have an older Node.js version, you need to upgrade it first.
Unexpected token ’export’ when running Express framework
If you see this error when running the Express framework, check that you’re not mixing the CommonJS module.exports
syntax with ESM export
syntax.
Unless you run Babel or Webpack to build your project, a vanilla Express project commonly uses the CommonJS syntax, so the export
keyword is not supported.
Unexpected token ’export’ when running Next.js React app
When running Next.js application, you might encounter this error because a dependency from the node_modules
folder only support the ESM syntax.
When the browser executes your application, the error occurs because it’s not supported natively.
To resolve this error, you need to install the next-transpile-modules
package, which allows you to transpile code from the node_modules
folder to CommonJS syntax.
Use npm or Yarn to install the package:
npm i next-transpile-modules
# or
yarn add next-transpile-modules
Then open your next.config.js
file and add the following lines:
const transpiledModules = require('next-transpile-modules')(["react-icons"]);
Replace “react-icons” with the name of the package that’s causing the error. If you have more than one package, use a comma to separate the package names.
Once finished, run your application again. The error should now disappear.
Conclusion
The export
keyword is an additional JavaScript syntax that’s not supported by default.
When running JavaScript code that uses the export
syntax, you need to enable ESM support, regardless if you use a browser or Node.js to run the code.
Through this post, you’ve seen how to enable ESM support both for browser and Node.js. I hope you’re able to resolve this error. Happy coding! 👍