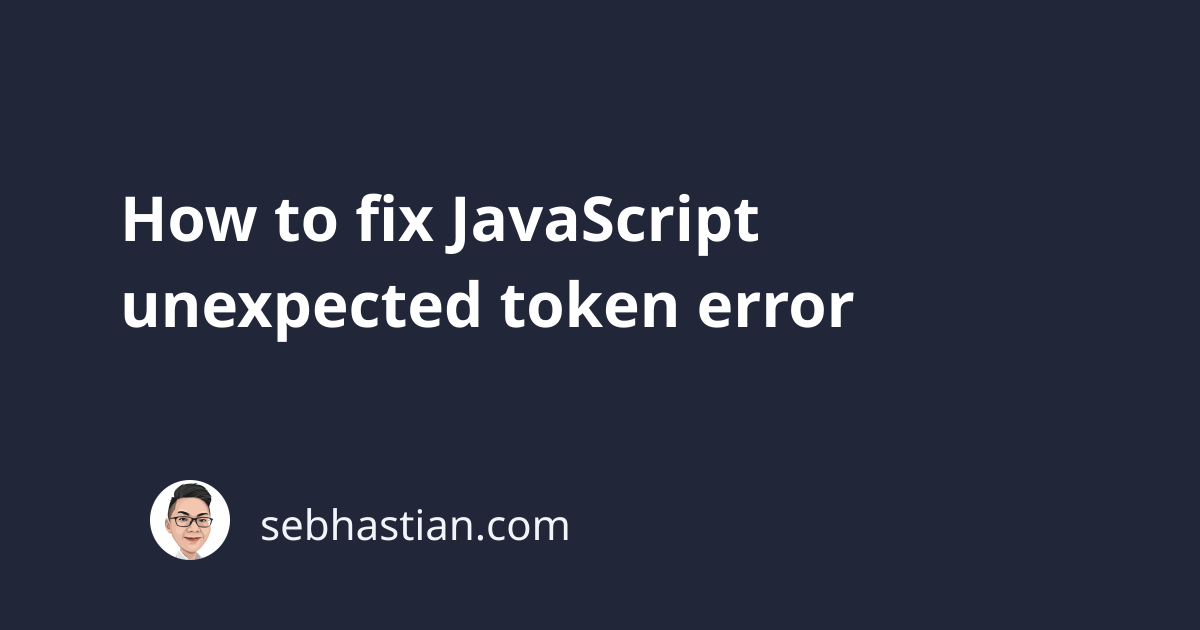
Sometimes you will encounter an unexpected token error in JavaScript as follows:
let val+ = "Hello";
// Uncaught SyntaxError: Unexpected token '+'
In the code above, the unexpected token error happens because the plus symbol +
is reserved in JavaScript for concatenation and adding two numbers together. It can’t be used for naming variables, so JavaScript throws the unexpected token error.
As you write your JavaScript application, the unexpected token error always occurs because JavaScript expected a specific syntax that’s not fulfilled by your current code. You can generally fix the error by removing or adding a specific JavaScript language symbol to your code.
In another example, the following function
code lacks the closing round bracket )
after the parameter num
:
function square(num {
return num * num;
}
But when you run the code, it says Error: Unexpected token '{'
because JavaScript expects the parenthesis to be closed.
function square(num) {
return num * num;
}
When you’re using a code editor like VSCode, you may notice the syntax color difference between the correct and the wrong code as follows:
As you can see from the screenshot above, the correct code will color the return
letter purple, but the wrong code simply doesn’t do that.
The cause for unexpected token error for your code may be different, so you need to understand basic JavaScript rules and figure out the right solution yourself.
You could have extra brackets somewhere, like in the following code:
// Unexpected token ')'
for (i = 0; i < 10; i++))
{
console.log(i);
}
Or you may miss a semicolon symbol ;
somewhere as in the code below:
// Unexpected identifier
for (i = 0 i < 10; i++)
{
console.log(i);
}
Whatever the case is, you need to trace the error message and see which line causes you the problem, and you have to apply the right fix yourself.