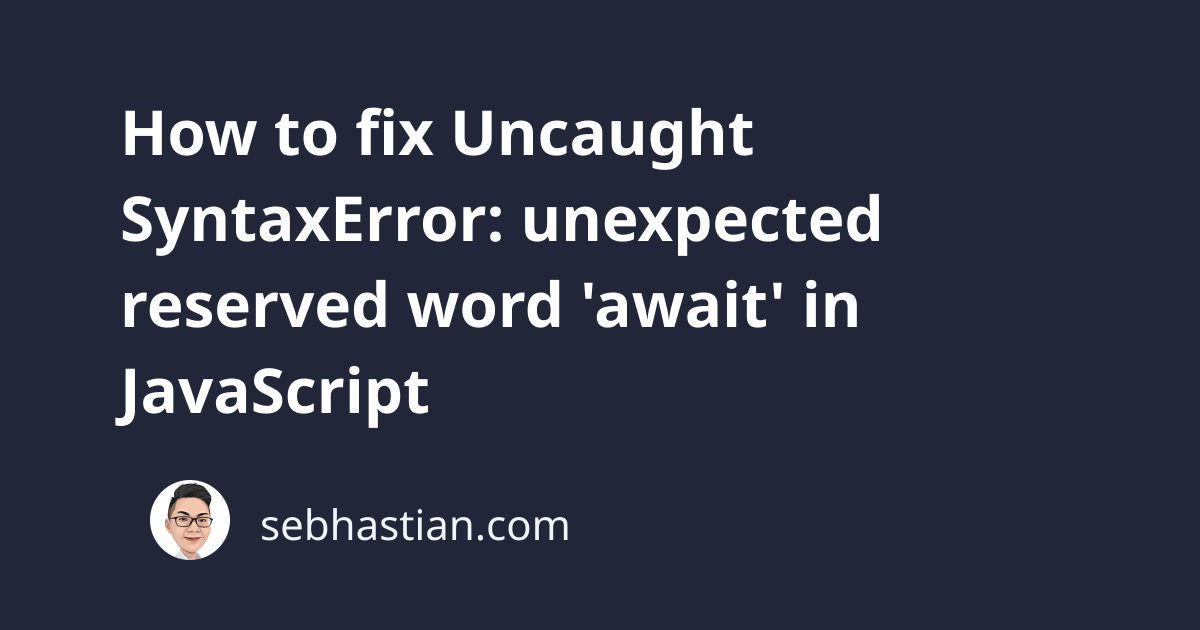
When running JavaScript code, one common error you might encounter is:
SyntaxError: Unexpected reserved word ('await')
This error occurs when you use the await
keyword inside a function that’s not specified as an async
function.
To resolve this error, you need to add the async
modifier to the function.
In this tutorial, let me show you an example that causes this error and how I fix it.
How to reproduce the error
Suppose you want to perform an asynchronous call using the Promise
object.
You created a function and added an await
call as follows:
function getUser() {
const result = await Promise.resolve("Nathan");
return result;
}
You don’t even need to call the function. When you run the code above, JavaScript will throw an error:
Uncaught SyntaxError: Unexpected reserved word
While the error doesn’t mention the word await
, this error is definitely caused by that word.
How to fix this error
When you use the await
modifier, you need to mark a function as an asynchronous function.
To resolve this error, add the async
keyword before the function
keyword as shown below:
async function getUser() {
const result = await Promise.resolve("Nathan");
return result;
}
const response = await getUser();
console.log(response); // Nathan
By adding the async
keyword, the error is now resolved.
Note that the code above still triggers the same error when you run it using Node.js.
In Node.js, you need to set the type
attribute to "module"
in your package.json
file:
// package.json
{
"name": "example-js",
"type": "module",
"version": "1.0.0",
}
Now the await
keyword will work at the top-level body (in the global scope)
If you see the same error from the browser, then add the type="module"
attribute to your <script>
tag as follows:
<script type="module">
async function getUser() {
const result = await Promise.resolve("Nathan");
return result;
}
const response = await getUser();
console.log(response); // Nathan
</script>
You need to add the src
attribute if the script is an external script.
Fix async in nested function
Keep in mind that you need to add the async
modifier to all nested functions that use the await
keyword.
If you don’t add the async
keyword to the nested function, then the error will be triggered.
In the example below, note how the second arrow function doesn’t have the async
modifier:
const createUser = async(username) => {
result = () => {
await fetch('https://api-example.com/user', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ username }),
});
}
}
This will cause the error because the function that has the await
modifier is not an async
function.
You need to add the async
modifier in the highlighted line as follows:
const createUser = async(username) => {
result = async() => {
await fetch('https://api-example.com/user', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ username }),
});
}
}
Now JavaScript will run the code without any errors.
And that’s how you solve the SyntaxError: Unexpected reserved word ('await')
in JavaScript. I hope this tutorial helps. See you later! 👋