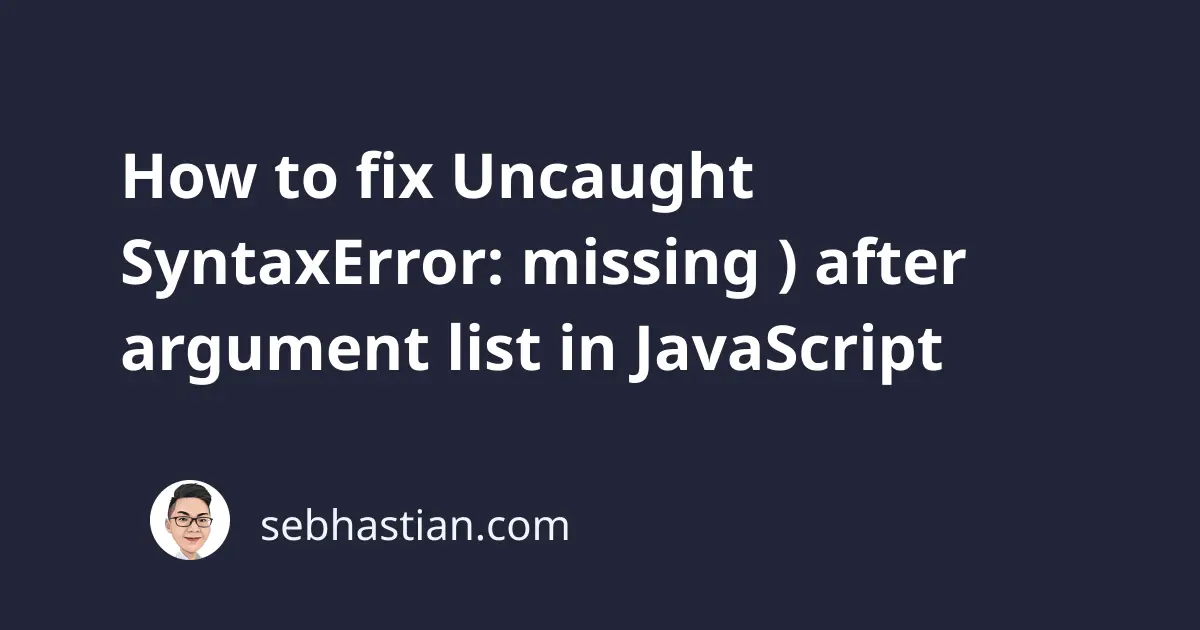
One error you might encounter when running JavaScript code is:
Uncaught SyntaxError: missing ) after argument list
This error means you have a syntax error when calling a JavaScript function. You might forget to separate arguments with a comma, or add a plus +
operator to concatenate 2 or more strings.
To fix this error, you need to ensure that you’re using the correct syntax when calling a function.
Let me show you an example that causes this error and how I fix it.
Examples that cause the error and how to resolve them
Suppose you try to call the console.log()
method to print a string as follows:
console.log("a" "b"); // ❌
You passed two strings a
and b
to the console.log()
method, but there’s no comma to separate the arguments. An error will be raised:
Uncaught SyntaxError: missing ) after argument list
To resolve this error, add a comma to separate the arguments as shown below:
console.log("a", "b"); // ✅
Now the code runs without any errors.
Another example that can trigger this error is when you forget to add a +
operator between 2 or more strings.
In the example below, you want to print the string and a variable:
const name = "Nathan"
console.log("Hello, " name); // ❌
The error occurs because there’s no +
sign between the string and the variable.
To resolve this error, add a +
operator between the string and the variable to concatenate them:
const name = "Nathan"
console.log("Hello, " + name); // ✅
Or you can also use a template literal as follows:
const name = "Nathan"
console.log(`Hello, ${name}`); // ✅
Now JavaScript knows you want to concatenate the variable in the string.
The third example that causes this error is when you have an unterminated string in your argument list. Consider the example below:
console.log('It's a great day'); // ❌
Here, the single quote symbol is used three times. JavaScript thinks the closing bracket )
is a part of the string.
When you need to use the same symbol as the string marker, you need to escape it using the backslash \
operator. Here’s an example:
console.log('It\'s a great day'); // ✅
console.log("You said, \"Good morning!\""); // ✅
You can also use a template literal to make the string wrapped in backticks. This way, you can use single and double quotes without escaping them:
console.log(`It's a great day`); // ✅
console.log(`You said, "Good morning!"`); // ✅
When the string is wrapped correctly, the error should disappear.
Conclusion
The JavaScript SyntaxError: missing ) after argument list
occurs when you have a syntax error when calling a function.
To resolve this error, make sure that you’ve stated the arguments for the function call correctly.
The examples in this tutorial should help you solve the error. Happy coding! 👍