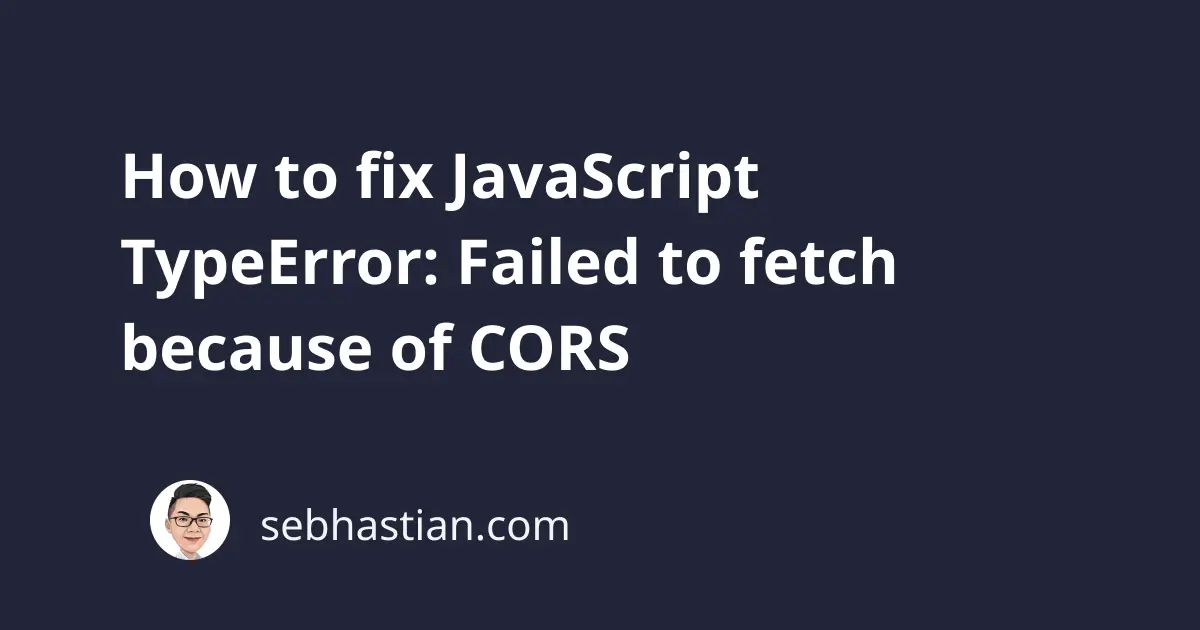
When using JavaScript to fetch data from an API, you might get an error saying ‘TypeError: Failed to fetch’. This error mostly occurs because of a CORS or Cross-Origin Resource Sharing issue, where your Fetch request is rejected because of no resource sharing policy.
For example, I run code to fetch data from an API as follows:
fetch('https://example.com/api/not-exists')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
And here’s the response I got:
Access to fetch at 'https://example.com/api/not-exists' from origin 'x' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
If an opaque response serves your needs, set the request's mode to 'no-cors' to fetch the resource with CORS disabled.
TypeError: Failed to fetch
As you can see from the response, the request to fetch has been blocked by CORS because there’s no ‘Access-Control-Allow-Origin’ header set on the origin.
How to fix this error
To fix the CORS policy, you need to access your API server and specifically add a CORS policy to enable fetch requests. Depending on the programming language used by your API, there are different ways to do this.
1. CORS for Node.js + Express framework
For API developed using Node.js and Express, you can use the cors
package to open CORS access as shown below:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
app.get('/api/users', (req, res) => {
const data = { message: 'Success' };
res.json(data);
});
app.listen(8000, () => {
console.log('Server listening on port 8000');
});
The above example enables CORS from all origin. If you only want CORS enabled for a specific website, you can add the origin
option when calling the cors()
function inside app.use()
like this:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors({origin: 'http://example.com'}));
app.get('/api/users', (req, res) => {
const data = { message: 'Success' };
res.json(data);
});
app.listen(8000, () => {
console.log('Server listening on port 8000');
});
This way, only requests from ’example.com’ will be allowed. Everything else will be rejected.
2. Python Flask
If you use Python Flask framework to develop your API, you can add the CORS policy to the response headers as shown below:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/users')
def get_user_data():
data = {'key': 'value'}
response = jsonify(data)
response.headers.add('Access-Control-Allow-Origin', '*')
response.headers.add('Access-Control-Allow-Methods', 'GET,PUT,POST,DELETE')
response.headers.add('Access-Control-Allow-Headers', 'Content-Type')
response.headers.add('Access-Control-Allow-Credentials', 'true')
return response
if __name__ == '__main__':
app.run()
Note that you need to add all the required headers for CORS policy.
3. Java Spring Boot
If you use Java Spring Boot, then add the @CrossOrigin
annotation to enable CORS for your endpoints:
@RestController
@RequestMapping("/user/1")
@CrossOrigin(origins = "*")
public class UserController {
@GetMapping("/user")
public String get_user_data() {
return "Success!";
}
}
You can specify *
as the origins
parameter to enable request from all origins.
Don’t Use The no-cors Option
Reading the error message, you can see that the console recommends adding the no-cors
option to your fetch()
call.
You can add this option next to the URL you passed in the request like this:
fetch('https://example.com/api/not-exists', {
mode: 'no-cors',
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
But this option usually doesn’t fix the issue because no-cors
prevent JavaScript from reading the contents of the response body and headers. Not sure why that would be recommended by the console.
Conclusion
The ‘TypeError: Failed to fetch’ usually occurs when you didn’t specify a CORS policy in your API server. By default, a web server would block requests from other origins to prevent unauthorized access.
To resolve this error, you can enable CORS policy from your API server. How to enable the policy depends on what framework you’re using to create that API.
Once the CORS policy is added, the fetch error should be resolved. Happy coding!