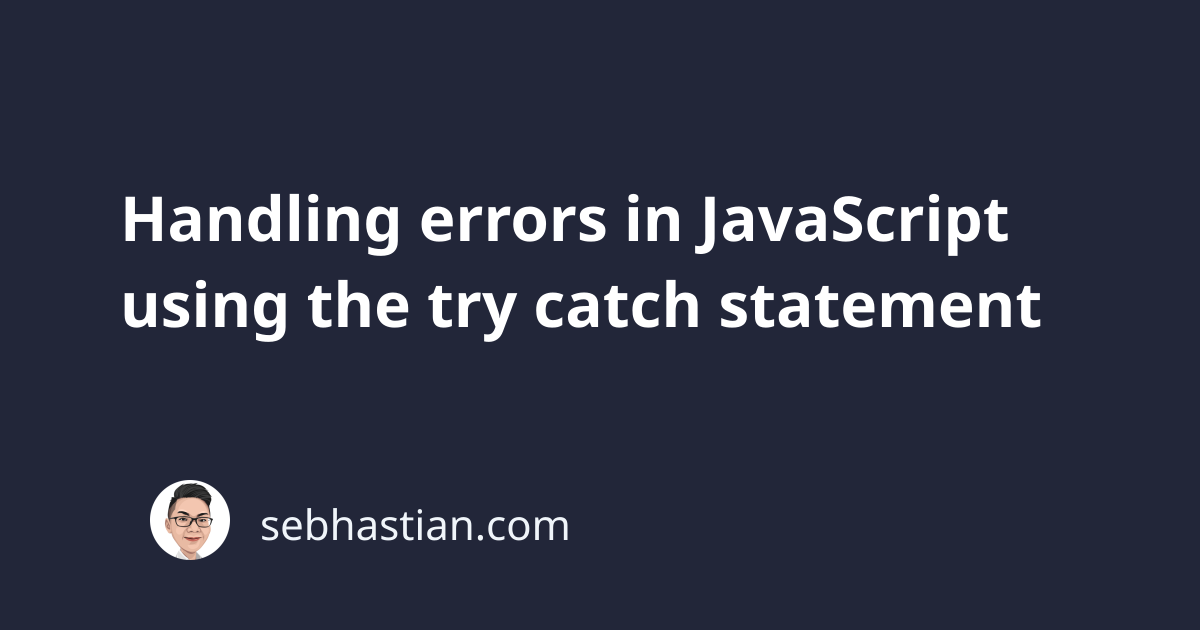
As you write your JavaScript application, there will be times when you will unexpectedly hit an error. The error might be caused by unexpected user input, a fault in the code, or a server response timeout.
For example, you can cause an error by calling a function you haven’t defined in your code:
printFn();
// Error: printFn is not defined
The kind of error above will cause JavaScript to crash and stop further code execution.
printFn(); // code below this line won't get executed
console.log("Hello there");
The try
and catch
statements are a group of statement that allows you to control what happens when JavaScript encounter an error just like the one above. Here’s how the code recipe looks like:
try {
// code...
} catch (err) {
// error handling
}
Both try
and catch
statements are followed by a block of code that you can write as the body of those statements. They work as follows:
- The code you write inside the
try
block will be executed as normal - If there is no error until the end of the
try
code, thecatch
block will be skipped - When the
try
code hits an error, JavaScript will run thecatch
block - JavaScript will pass the error details into the
err
variable. You’re free to name it anything
Now let’s try to run the code below to see try..catch
in action:
try {
printFn();
} catch (err) {
console.error(err);
}
console.log("Hello there");
The output will be as follows:
> ReferenceError: printFn is not defined
> "Hello there"
While the call to printFn()
still cause an error, JavaScript will handle that error nicely using the catch
block, then continued to execute the rest of the code you write. If the code inside try
block doesn’t cause any error, the catch
block will be skipped:
try {
let name = "John";
} catch (err) {
console.error(err);
}
console.log("Hello there");
But what if you still want to stop the execution of your code when the catch
block is executed? Then you can use the return
statement, which will stop the current script execution:
try {
printFn();
} catch (err) {
console.error(err);
return;
}
console.log("Hello there");
JavaScript also has the finally
statement, which allows you to write a block of code that will be executed regardless of the result of both try
and catch
block. With the finally
statement, you can write three forms of error controller statements:
try..catch
statementtry..catch..finally
statementtry..finally
statement
The code inside finally
block will be executed even when you have a return
statement inside the catch
block:
try {
printFn();
} catch (err) {
console.error(err);
return;
} finally {
console.log("Hello there");
}
But please be advised: JavaScript won’t execute the finally
block in a try..finally
statement if your try
block actually hits an error. This is because when the try
block hits an error, JavaScript will move the control into the catch
block. But in this case you have no catch
block:
try {
printFn();
} finally {
// code here won't be executed
console.log("Hello there");
}
Because there is no catch
block, JavaScript will use its default error handler and call it a day, ignoring the finally
block.
The try..catch
statement would be the most common form you will find in real application projects.