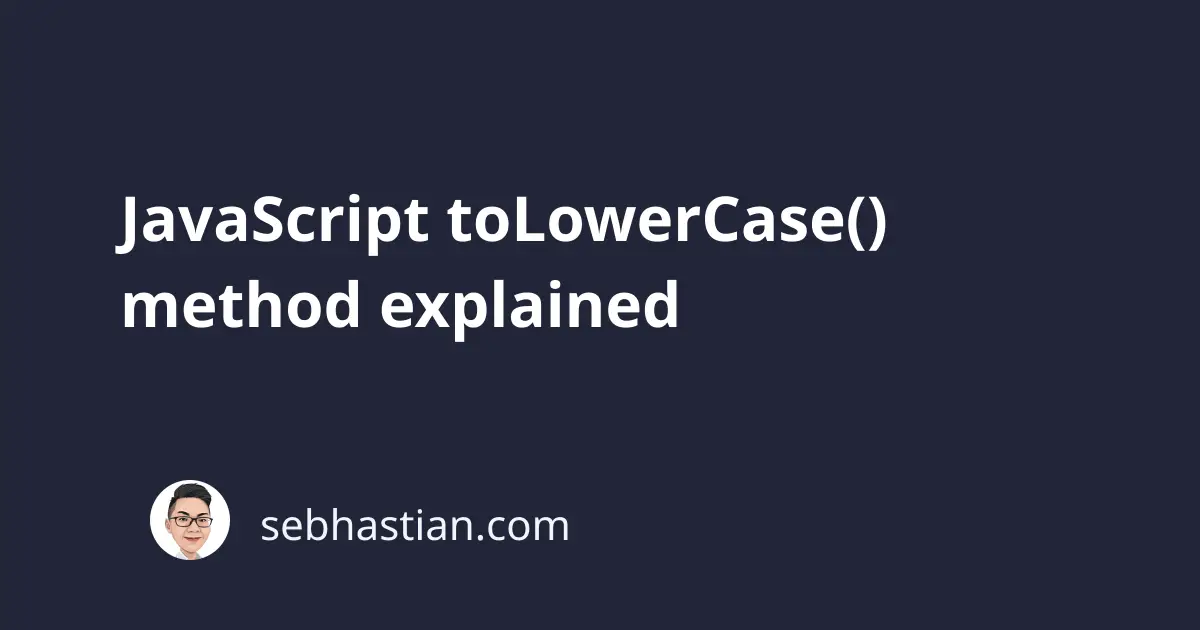
The JavaScript toLowerCase()
method is used to convert a string into its lowercase version.
The method can be called from any valid string
value you may have in your source code.
Here’s an example of using the toLowerCase()
method:
let str = "GOOD MORNING FROM MARS!";
console.log(str.toLowerCase());
// good morning from mars!
The toLowerCase()
method returns a new value, so the original value is not affected.
You can use the toLowerCase()
method to make a lowercase string except for the first letter with the help of the charAt()
method:
function lowerCaseExceptFirstLetter(str) {
return str.charAt(0) + str.substring(1).toLowerCase();
}
let str = "GOOD MORNING FROM MARS!";
console.log(lowerCaseExceptFirstLetter(str));
// Good morning from mars!
The method above only leaves the first letter of the sentence with an uppercase letter.
If you want to capitalize the first letter of every word, you need to create a function that uses split()
to split the string into an array first.
Let’s name this function as lowerCaseWords()
function:
function lowerCaseWords(str) {
// 1. Create an array out of the string
let strArray = str.split(" ");
}
Next, you use the map()
method to iterate over the array.
Inside the map, use the same charAt()
and toLowerCase()
methods to convert the string to lowercase except for the first letter. Assign the returned values from the mapping operation back to the strArray
:
function lowerCaseWords(str) {
// 1. Create an array out of the string
let strArray = str.split(" ");
// 2. Lowercase the words except the first letter
strArray = strArray.map((str) => {
return lowerCaseExceptFirstLetter(str);
});
}
Finally, call the join()
method to put the array of words back into a single sentence string and return it from the function.
Here’s the full function code and its test result:
function lowerCaseExceptFirstLetter(str) {
return str.charAt(0) + str.substring(1).toLowerCase();
}
function lowerCaseWords(str) {
// 1. Create an array out of the string
let strArray = str.split(" ");
// 2. Lowercase the words except the first letter
strArray = strArray.map((str) => {
return lowerCaseExceptFirstLetter(str);
});
// 3. Put the array back into a string and return it
return strArray.join(" ");
}
// Test the function
let str = "GOOD MORNING FROM MARS!";
console.log(lowerCaseWords(str));
// Good Morning From Mars!
Now you’ve learned how the JavaScript toLowerCase()
method works and how it can be useful to convert strings into lowercase letters.
The toLowerCase()
method is available from JavaScript ES1 version. It’s fully supported by all browsers today.
Feel free to use the code in this tutorial for your project. 😉