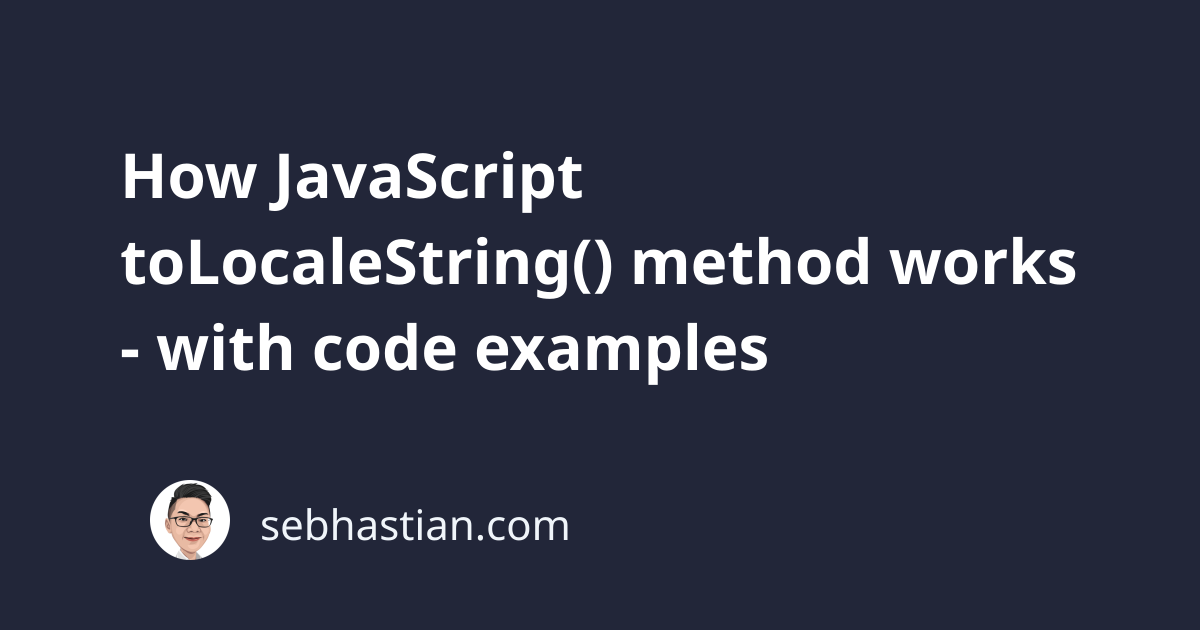
The JavaScript toLocaleString()
method is available from JavaScript Date
and Number
objects.
The purpose of the toLocaleString()
method is to create a locale-sensitive value of your date or number value.
This tutorial will show you how the method works both for Date
and Number
type values.
Let’s start with the Date
type value first.
JavaScript Date toLocaleString() method explained
The JavaScript Date.prototype.toLocaleString()
method is available to call from all Date
type values you might define in your source code.
For example, suppose you want a Date
to be written in the Month-Day-Year format like in the United States. Here’s how you do it:
let date = new Date();
console.log(date);
console.log(date.toLocaleString("en-US"));
The JavaScript console will produce the following output:
Mon Mar 14 2022 10:10:35 GMT+0800 (Singapore Standard Time)
3/14/2022, 10:10:35 AM
Keep in mind that the Date.toLocaleString()
method returns a string
, so you can’t call Date
object methods on the returned value.
A TypeError
will be thrown when you call any Date
methods from the returned value as shown below:
let date = new Date(); //
let localeDate = date.toLocaleString("en-US");
console.log(date.getMonth()); // 2 for March
console.log(localeDate.getMonth()); // TypeError
The full syntax of the Date.toLocaleString()
method is as follows:
toLocaleString(locales, options);
The method has two optional parameters:
- The
locales
parameter accepts astring
value that represents the locale language and date format you want to use - The
options
parameter accepts anobject
of options that customize the returned value
The locales
parameter affects both the date format and the language used in the returned value.
For example, you can create a date in German format and language with the following code:
let date = new Date();
let localeDate = date.toLocaleString("de-DE", {
weekday: "long",
day: "numeric",
year: "numeric",
month: "long",
});
console.log(localeDate); // Montag, 14. März 2022
The options
parameter allows you to customize the returned value as shown above.
Instead of using numbers to represent the month, you can use the full name of the month with the month: "long"
option.
When you don’t pass any valid locales
parameter, JavaScript will use the default locale from your computer. This is particularly useful if you only want to customize the returned value using the options
parameter.
In the example below, the value undefined
is passed to the locales
parameter. This causes JavaScript to transform the returned date value using the default format:
let date = new Date(); // Singapore locale
let localeDate = date.toLocaleString(undefined, {
weekday: "long",
day: "numeric",
year: "numeric",
month: "long",
});
console.log(localeDate); // Monday, March 14, 2022
You can see the full list of possible values for the parameters from MDN International Date and Time format here.
And that’s how the toLocaleString()
method works for Date
type values.
Let’s see how the method works for Number
type values next.
JavaScript Number toLocaleString() method explained
The Number.prototype.toLocaleString()
method is used to transform any given Number
value into its locale-sensitive representation.
The method is particularly useful to add thousand separators to your number:
let num = 123456789;
// default locale digits
console.log(num.toLocaleString()); // 123,456,789
// Germany uses comma as decimal separator and period for thousands
console.log(num.toLocaleString("de-DE")); // 123.456.789
The Number.toLocaleString
method accepts two parameters:
locales
string for the locale number format you want to useoptions
object to customize the returned number value
For example, you can return a monetary currency using the style
and currency
options:
let num = 123456789;
let jpnYen = num.toLocaleString("ja-JP", {
style: "currency",
currency: "JPY",
});
console.log(jpnYen); // ¥123,456,789
And that’s how the Number.toLocaleString()
method works.
You can see the full list of possible parameters for Number format here.
Conclusion
The toLocaleString()
method is a JavaScript method available for Number
and Date
type values.
This method allows you to create a representation of the values in a locale-sensitive format.
The locale values can be customized using the locales
and options
arguments to fit your requirements.