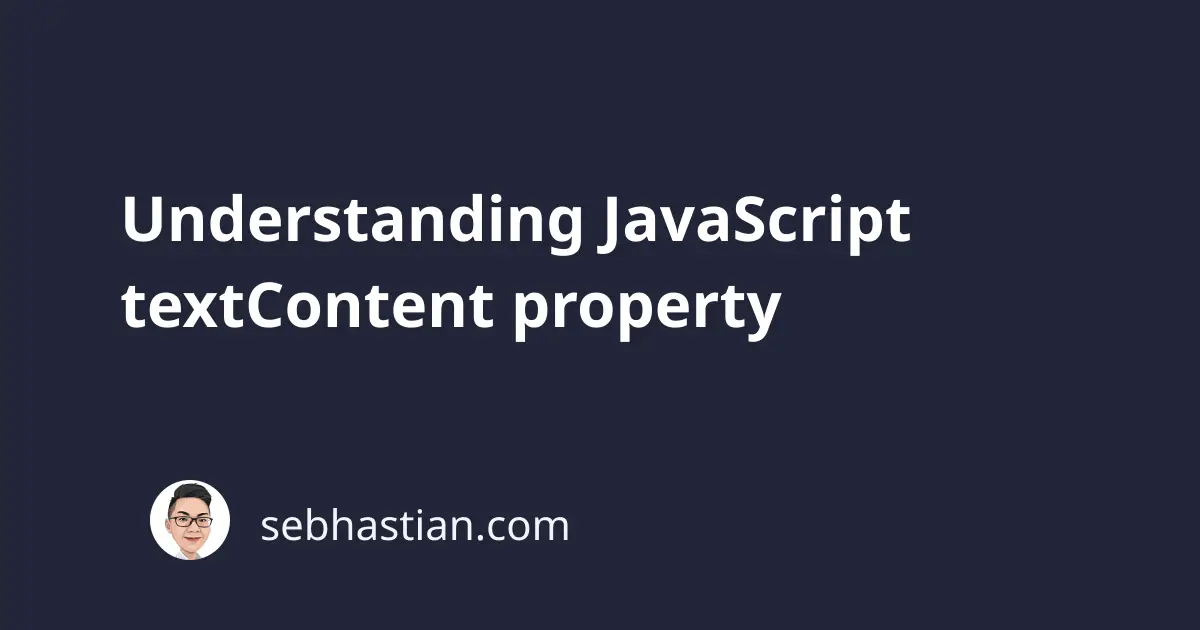
When manipulating DOM elements in the browser, you can use the textContent
property to retrieve the text content of a certain element and all its descendants.
For example, let’s say you have the following HTML <body>
tag:
<body>
<div id="partOne">
<p>Roses are red</p>
<p>Violets are blue</p>
<p>Sunflowers are yellow</p>
</div>
</body>
You can use textContent
property to grab the text content of the <div>
tag as show below:
let str = document.getElementById("partOne").textContent;
console.log(str);
The output of textContent
property will be as follows:
Roses are red
Violets are blue
Sunflowers are yellow
As you can see from the output above, The textContent
property only get the text inside your element, but not the HTML tags. When you need to get the HTML tag as well, you need to use the innerHTML
property instead of textContent
property:
let str = document.getElementById("partOne").innerHTML;
console.log(str);
The output of innerHTML
property will be as follows:
<p>Roses are red</p>
<p>Violets are blue</p>
<p>Sunflowers are yellow</p>
You can also change the textContent
property, which will remove all existing child elements, replacing it with a single text as follows:
document.getElementById("partOne").textContent = "Hello World!";
The HTML <body>
tag will be modified as shown below:
<body>
<div id="partOne">Hello World!</div>
</body>
Please keep in mind that the textContent
property is not supported by Internet Explorer 8 or below.