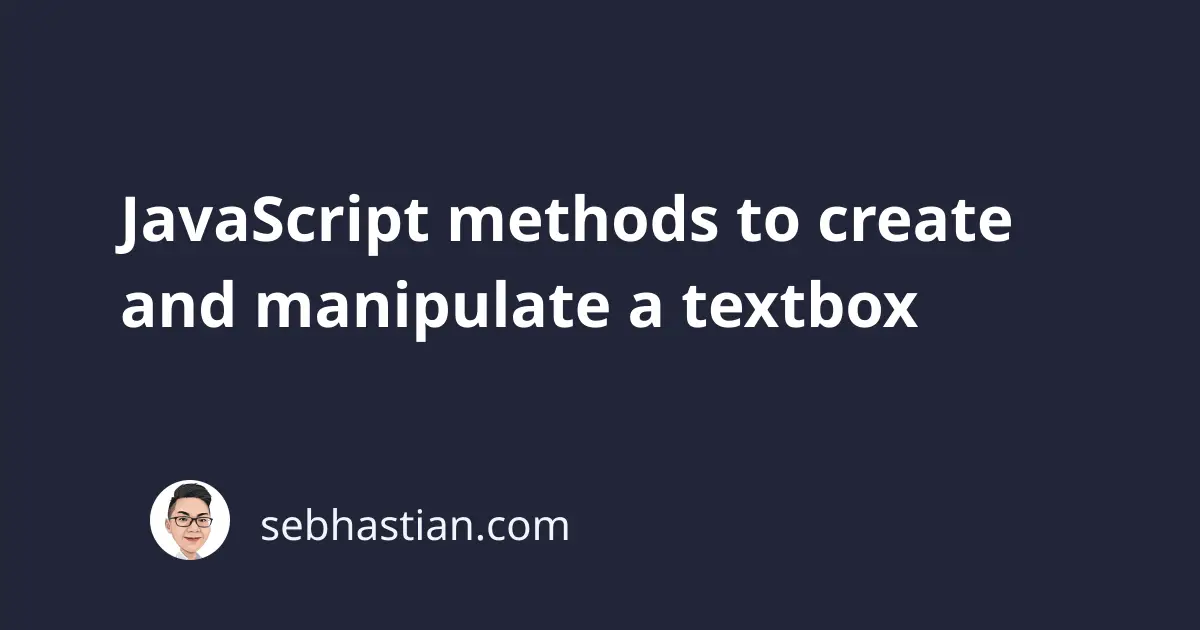
This tutorial will help you to understand how you can create a textbox element as well as how you can manipulate various attributes of the textbox using JavaScript.
Create a textbox using JavaScript
You can create a textbox using JavaScript in two simple steps:
- First, you need to use the
createElement("input")
method of thedocument
object to create an<input>
element. - Then, you need to set the
type
attribute of the<input>
element that you’ve created to"text"
using theElement.setAttribute()
method.
The code inside the <script>
tag below shows how you can create a textbox and append it to the body element:
<body>
<h1>Create Textbox element using JavaScript</h1>
<script>
const input = document.createElement("input");
input.setAttribute("type", "text");
document.body.appendChild(input);
</script>
</body>
The resulting HTML document will be as follows:
<body>
<h1>Create Textbox element using JavaScript</h1>
<input type="text">
</body>
Now that you know how you can create a textbox using JavaScript, let’s learn how you can manipulate various attributes of the textbox element.
Adding id attribute to the textbox using JavaScript
First, you may want to add an id
attribute to the element so that you can easily fetch the element and retrieve its value. You can easily add the attribute by using the same setAttribute()
method.
The code below shows how you can add the id
attribute with "username"
as its value:
input.setAttribute("id", "username");
Adding a label to the textbox using JavaScript
Next, you may want to add a <label>
tag to the textbox to make it clear to users what the textbox is there for. You can do so by using the same createElement()
method but replace the parameter with "label"
.
For example, you may want to create a textbox so that users can enter their username
. Here’s how you can create a label for that:
const label = document.createElement("label");
label.setAttribute("for", "username");
label.innerHTML = "Username: ";
The for
attribute of the <label>
element must match the id
attribute of the <input>
element so that the label will be bound to the right element. The label text will be read by the device for the user.
You also need to set the innerHTML
property of the element. The text inside will be read by screen-reader devices.
Since you need to append the label before the textbox element, you have two choices:
- Append the label before the textbox with the same
appendChild()
method - Grab the textbox element using
document.getElementById()
method, then useinsertBefore()
method to insert the label before the textbox.
For the first choice, you need to add the code for creating the label before the textbox:
// create label
const label = document.createElement("label");
label.setAttribute("for", "username");
label.innerHTML = "Username: ";
// insert label
document.body.appendChild(label);
// create textbox
const input = document.createElement("input");
input.setAttribute("id", "username");
input.setAttribute("type", "text");
// insert textbox
document.body.appendChild(input);
Now you’re going to have the following output inside the <body>
tag:
<label for="username">Username: </label>
<input id="username" type="text">
As for the second choice, You need to call the insertBefore()
method on the <input>
element’s parent, which is the <body>
element in this example.
The insertBefore()
element requires 2 parameters:
- The
newElement
to be inserted - And the
existingElement
where thenewElement
will be prepended.
The syntax for the method is as follows:
Element.insertBefore(newElement, existingElement);
Let’s see how it works step by step. First, create the textbox element and append it to the HTML body:
// create textbox
const input = document.createElement("input");
input.setAttribute("id", "username");
input.setAttribute("type", "text");
// insert textbox
document.body.appendChild(input);
Then, create the label element:
const label = document.createElement("label");
label.setAttribute("for", "username");
label.innerHTML = "Username: ";
Finally, how you can grab the textbox element and call body.insertBefore()
method to insert the <label>
element above the textbox:
// grab the username textbox
const usernameText = document.getElementById("username");
// use insertBefore() method
document.body.insertBefore(label, usernameText);
The full code will be as shown below:
const input = document.createElement("input");
input.setAttribute("id", "username");
input.setAttribute("type", "text");
document.body.appendChild(input);
const label = document.createElement("label");
label.setAttribute("for", "username");
label.innerHTML = "Username: ";
const usernameText = document.getElementById("username");
document.body.insertBefore(label, usernameText);
Now you’ve learned how to insert a label for the textbox element.
Manipulate textbox placeholder attribute using JavaScript
You may also want to set the placeholder
attribute for the textbox to give your users a hint about the expected value for the textbox.
Since your textbox already has an id
attribute, you can use the document.getElementById()
method to fetch the element and use the setAttribute()
method to set the placeholder:
const usernameText = document.getElementById("username");
usernameText.setAttribute("placeholder", "Input username here...");
And with that, your textbox will have the placeholder
attribute set.
Manipulate the value attribute of the textbox using JavaScript
Finally, the input element also has the value
property which you can use the get or set the value
stored inside the textbox.
For example, type any text you want into the textbox and then fetch its value:
const usernameText = document.getElementById("username");
const value = usernameText.value;
console.log(value); // your textbox value
You can also set the value
as follows:
const usernameText = document.getElementById("username");
usernameText.value = "Nathan";
And that’s how you can get or set the value
property of a textbox 😉