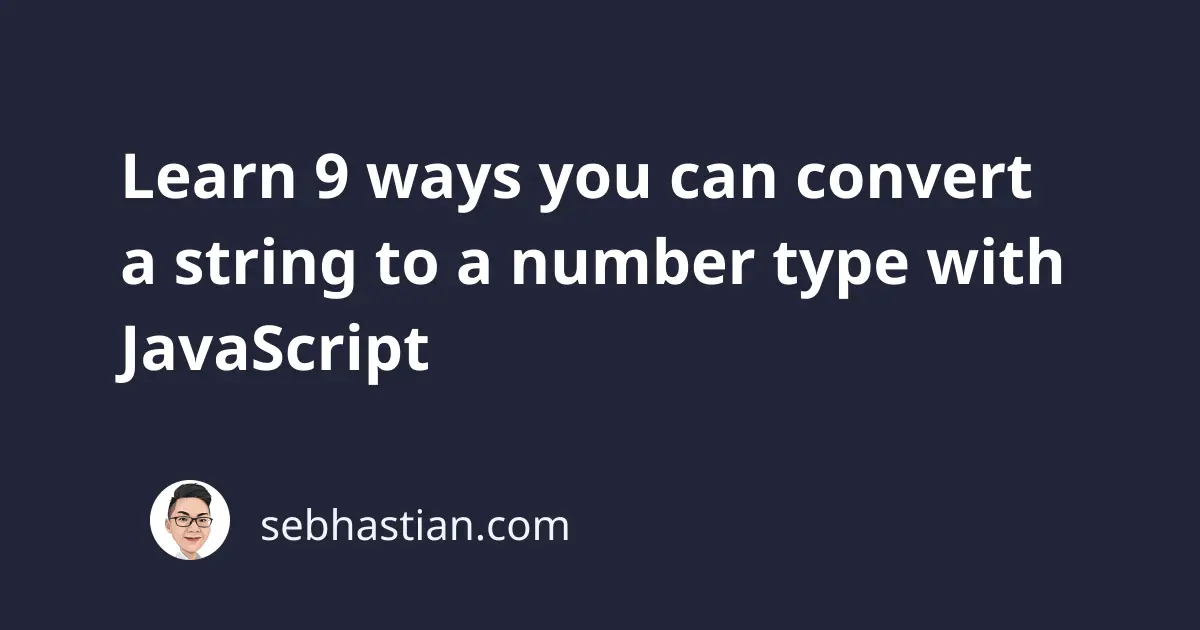
Depending on the conditions you have in your project, there are 9 ways to convert a string type into a number type in JavaScript
When you want a rounded number out of the string, then you can use these functions and methods:
parseInt()
Math.round()
Math.floor()
Math.ceil()
When you want a number with floating points, then you can use these functions and operators:
Number()
parseFloat()
- The unary
+
operator - The unary
-
operator - Implicit conversion with the minus, multiplication, division, or remainder operator
Let’s see an example of these functions in action next.
Create a rounded number from the string
The parseInt()
function parses a value you passed as its argument and tries to return a number that represents that value.
When you pass a string into the function, it will return a whole number as shown below:
parseInt("23"); // 23
// parsing a floating number string:
parseInt("7.31"); // 7
// rounded down even when the floating number > .49
parseInt("7.91"); // 7
// String with no number representative
parseInt("a"); // NaN
Note that strings with floating numbers are always rounded down, even when the floating number is greater than .49
.
You can use the Math.round()
method to round the string with floating numbers up or down depending on the floating number’s value:
Math.round("2.17"); // 2
// rounded up when floating number >= .5
Math.round("2.5"); // 3
// rounded down when floating number < .5
Math.round("2.49"); // 2
To always round the floating number down, use the Math.floor()
method:
Math.floor("2.17"); // 2
Math.floor("2.5"); // 2
Math.floor("2.49"); // 2
To always round the floating number up, use the Math.ceil()
method:
Math.ceil("2.17"); // 3
Math.ceil("2.5"); // 3
Math.ceil("2.49"); // 3
And that’s how you create a rounded number when converting a string into a number.
Next, let’s learn how to convert a string into a number and preserve the floating points.
Create a decimal number from the string
Decimal numbers are numbers with floating points. The native Number()
function in JavaScript will convert your string into its number representation without rounding the floating points:
Number("7"); // 7
Number("7.22"); // 7.22
Number("7.52"); // 7.52
Number("7.52.447"); // NaN
Next, the parseFloat()
function is a native JavaScript function to return a floating-point number from a value.
The code below shows how the parseFloat()
function works:
parseFloat("7"); // 7
parseFloat("7.52"); // 7.52
// still parse number in wrong format:
parseFloat("7.52.447"); // 7.52
parseFloat("7.52aabcs"); // 7.52
When encountering an incorrect number format, the parseFloat()
function still tries to return a number that represents the string.
On the other hand, the Number()
function immediately returns a NaN
when the number format is unrecognized.
The Number()
function is more strict than the parseFloat()
function.
Besides these two functions, you can also convert a string of decimal numbers using the unary plus (+
) and minus (-
) operator.
The +
operator evaluates the operand you added next to the operator and tries to return a number as shown below:
+"7.22"; // returns 7.22
+"777"; // returns 777
+"-52"; // -52
+"ab"; // NaN
The -
operator evaluates the given operand and negates the number, creating a negative number in the process:
The code below shows how the -
operator works:
-"7.22"; // -7.22
-"777"; // -777
// negative number string will return a positive number
-"-52"; // 52
-"-52.89"; // 52.89
-"ab"; // NaN
And that’s how the -
operator can be used to convert a string into a number.
JavaScript implicit type conversion with minus / multiplication / division / remainder operator
JavaScript automatically converts a string into a number when you use an arithmetic operator except for the unary +
operator.
Consider the following code examples:
"55" - 1; // 54
"5" * 2; // 10
"20" / 5; // 4
"100" % 6; // 4
"-7" - 3; // -10
When you use the +
operator, JavaScript will concatenate the string and the number instead of converting the string.
In the following code examples, the expressions produce a string instead of a number:
// returns a string:
"50" + 1; // "501"
"3.2" + 1; // "32.1"
// returns a number:
Number("5.7") + 3; // 8.7
+"6.1" + 3; // 9.1
You need to manually convert the string into a number before using the +
operator as shown above.
And that’s how you can convert a string into a number in JavaScript.
Depending on the requirements you have in your project, you can use one of the nine ways mentioned in this tutorial.
Thank you for reading. I hope this tutorial has been useful for you. 🙏