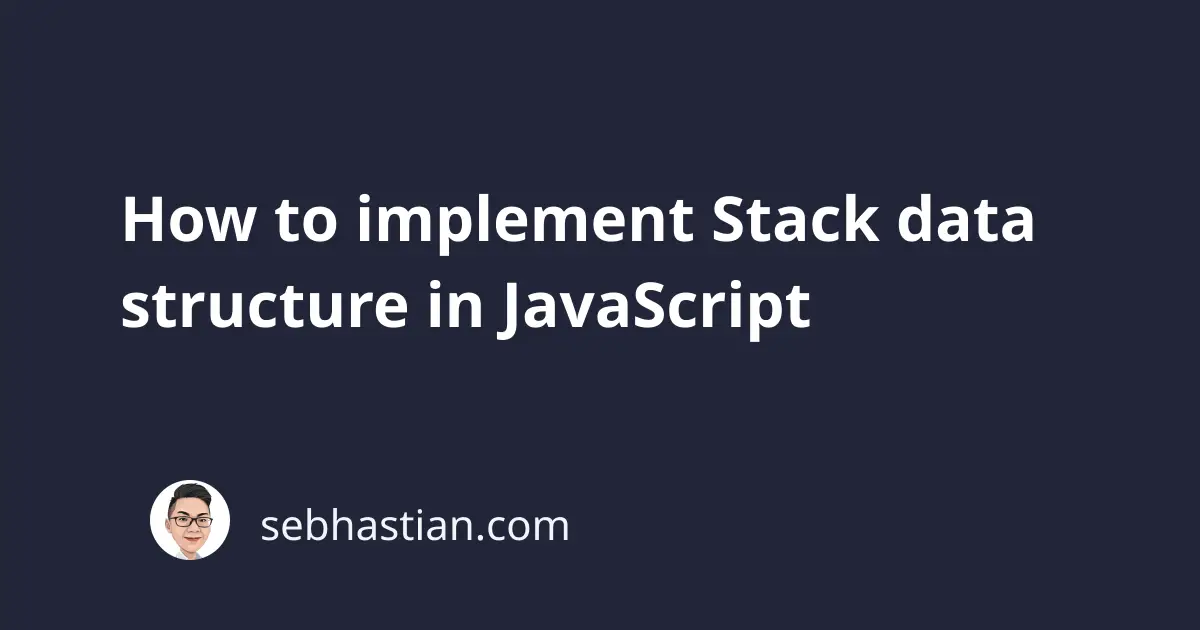
A stack is a linear data structure that follows a last-in-first-out (LIFO) principle.
This data structure resembles a collection of items that are arranged one on top of the other, like a stack of plates or books. Hence the name stack.
There are two main operations that can be done in a stack: push
and pop
.
The push
operation adds an element to the top of the stack, and the pop
operation removes an element from the top of the stack.
In this article, you will see how to implement a stack in JavaScript using an array. We’ll also discuss some common applications of stacks and the various ways in which you can use them in your code.
Stack implementation with Javascript array
To implement a stack in JavaScript using an array, you can create a class called Stack
that has an array property called items
to store the stack elements.
Here’s an example of how you can define the Stack
class:
class Stack {
constructor() {
this.items = [];
}
}
Next, you need to define the push
, pop
, peek
, isEmpty
, clear
, and size
methods. These methods will be used to perform the various stack operations.
The push
method adds an element to the top of the stack. You can implement it by using the push()
method from the array object:
// adds an element to the top of the stack
push(element) {
this.items.push(element);
}
The pop
method removes and returns the top element of the stack. You can implement it by using the pop
method of the items
array object:
// removes and returns the top element of the stack
pop() {
return this.items.pop();
}
The peek
method returns the top element of the stack without removing it. You can implement it by returning the last element of the items
array:
// returns the top element of the stack
peek() {
return this.items[this.items.length - 1];
}
The isEmpty
method returns a boolean value indicating whether the stack is empty or not.
You can implement it by checking if the length
of the items
array is equal to zero:
// returns true if the stack is empty, false otherwise
isEmpty() {
return this.items.length === 0;
}
The clear
method removes all elements from the stack. You can implement it by setting the items
array to an empty array:
// removes all elements from the stack
clear() {
this.items = [];
}
The size
method returns the size of the stack, which is the number of elements in the stack.
You can implement it by returning the length
of the items
array:
// returns the size of the stack
size() {
return this.items.length;
}
Now you have all the required methods implemented in the class.
Here’s the complete JavaScript code for the Stack
class:
class Stack {
constructor() {
this.items = [];
}
// adds an element to the top of the stack
push(element) {
this.items.push(element);
}
// removes and returns the top element of the stack
pop() {
return this.items.pop();
}
// returns the top element of the stack
peek() {
return this.items[this.items.length - 1];
}
// returns true if the stack is empty, false otherwise
isEmpty() {
return this.items.length === 0;
}
// removes all elements from the stack
clear() {
this.items = [];
}
// returns the size of the stack
size() {
return this.items.length;
}
}
Now that you have implemented the Stack
class, you can use it to perform stack operations in your code.
To use the stack, you need to create an instance of the Stack
class and call its methods.
Here’s an example of how you can use the Stack
class to perform some common stack operations:
const stack = new Stack();
// add a stack element
stack.push(10);
stack.push(20);
stack.push(30);
// perform operations
console.log(stack.size()); // 3
console.log(stack.peek()); // 30
console.log(stack.pop()); // 30
console.log(stack.peek()); // 20
stack.clear();
console.log(stack.isEmpty()); // true
As you can see, the Stack
data structure has been implemented successfully in JavaScript.
Applications of stacks
The stack data structure has many applications. Here are some common applications of a stack:
- Reversing a string: You can use a stack to reverse a string by pushing each character of the string onto a stack and then popping them off the stack to create a reversed string.
- Undo operation: You can use a stack to implement undo operation in applications such as text editors. You can push the previous state of the document onto a stack every time the user makes a change. The user can then undo the changes by popping the top element from the stack and restoring the previous state.
Conclusion
You’ve seen how to implement the stack data structure in JavaScript using an array in this article.
You’ve also learned some common applications of stacks. We also demonstrated how to use the Stack
class to perform various stack operations.
The stack data structure can be used to perform various operations, such as reversing a string or implementing undo operation.
I hope this article helps you in understanding how to implement and use a stack data structure in JavaScript.