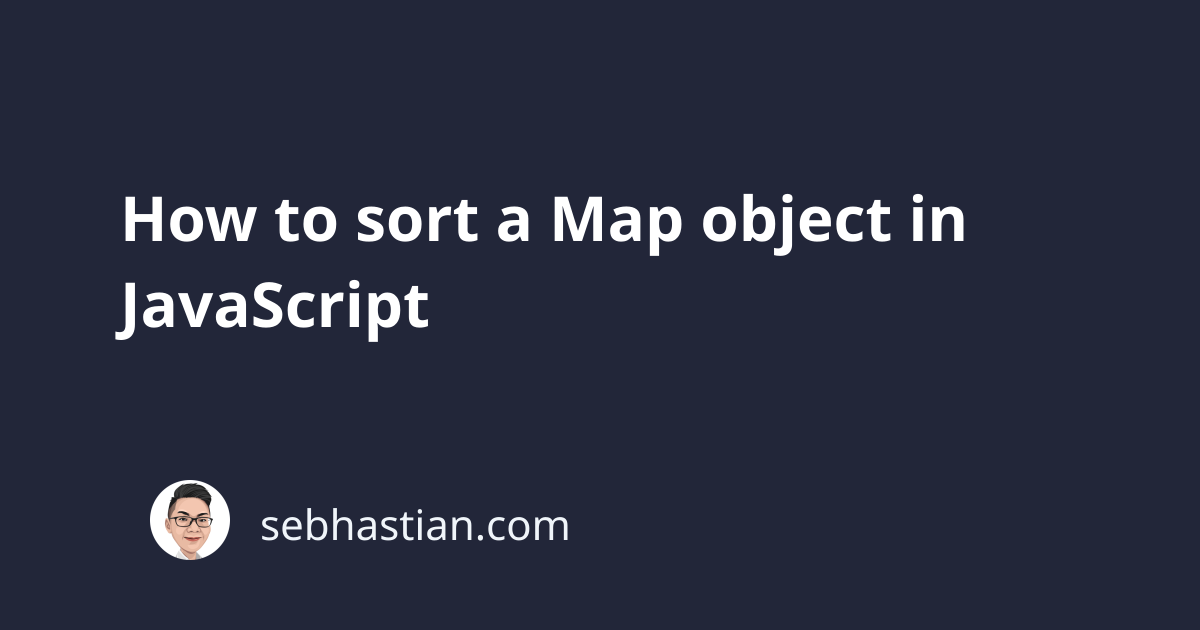
A JavaScript Map
object is a special data type designed to hold key-value pairs. It also preserves the insertion order of the elements you assigned to it.
The order of elements in a Map
object can’t be sorted directly. If you need to sort a Map
object elements, you need to convert the Map
object into an array, sort the values, then convert it back to a Map
.
There are two easy ways you can do this:
- Using the spread operator and
Array.sort()
method - Using the
Array.from()
and theArray.sort()
method - Bonus: Sort by values instead of keys
In this tutorial, I will show you how to sort a Map
object using the solutions mentioned above. Don’t worry, this will be quick and easy!
1. Using the spread operator and the sort() method
The JavaScript spread ...
operator can be used on a Map
object to get the key-value pairs as an array. See the example below:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
console.log(...myMap);
Output:
["z", 2] ["x", 1] ["c", 3]
Knowing this, you can turn a Map
object into an array by wrapping the ...myMap
syntax in square brackets like this:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
console.log([...myMap]);
Output:
[["z", 2], ["x", 1], ["c", 3]]
Now you have a two-dimensional array, where an array of key-value pairs are grouped in one parent array.
You can add the call to the Array.sort()
method right after the square brackets as follows:
[...myMap].sort();
The sort()
method will sort the child arrays in ascending order using their key values.
The last thing you need to do is to convert the array back to a Map
object. You need to call the Map()
constructor and put the array as its argument. Here’s the complete code:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map([...myMap].sort());
console.log(...sortedMap);
The result will be:
["c", 3] ["x", 1] ["z", 2]
Here, you can see that the new sortedMap
object has its key-value pairs sorted ascendingly based on the key values.
If you want to sort the key-value pair in descending order, you need to call the reverse()
method after the sort()
method as follows:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map([...myMap].sort().reverse());
console.log(...sortedMap);
Output:
["z", 2] ["x", 1] ["c", 3]
And that’s how you sort a Map
object in JavaScript using the spread operator and the array sort()
method.
2. Using the Array from() and sort() methods
The Array.from()
and the Array.sort()
methods can be used to sort a Map
object values.
You need to create an array from the map object, then call the sort()
method as follows:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map(Array.from(myMap).sort());
console.log(...sortedMap);
Output:
["c", 3] ["x", 1] ["z", 2]
To sort the key-value pairs in descending order, call the reverse()
method next to the sort()
method:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map(Array.from(myMap).sort().reverse());
console.log(...sortedMap);
Output:
["z", 2] ["x", 1] ["c", 3]
As you can see, the Array.from()
method is used in a similar manner as the spread operator in the previous solution.
Next, let’s see how you can sort a Map
object by values instead of keys.
3. Bonus: Sort by values instead of keys
To sort a Map
object by values, you need to add arguments to the sort()
method and pass a comparison function that compares the array values instead of keys.
See the example code below:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map([...myMap].sort((a, b) => a[1] - b[1]));
console.log(...sortedMap);
Output:
["x", 1] ["z", 2] ["c", 3]
Here, you can see that a comparison function is passed to the sort()
method. The values of the Map
object are stored in each array at index 1, so here we compare the value of a[1]
and b[1]
To compare by keys, you need to pass the index 0 instead of 1. But this is not needed as the sort()
method will sort using the value at index 0 by default.
And that’s it. If you want to sort by values in descending order, just swap a[1] - b[1]
with b[1] - a[1]
as follows:
const myMap = new Map();
myMap.set("z", 2);
myMap.set("x", 1);
myMap.set("c", 3);
const sortedMap = new Map([...myMap].sort((a, b) => b[1] - a[1]));
console.log(...sortedMap);
Output:
["c", 3] ["z", 2] ["x", 1]
I hope this tutorial is useful. Happy coding and see you next time! 👍