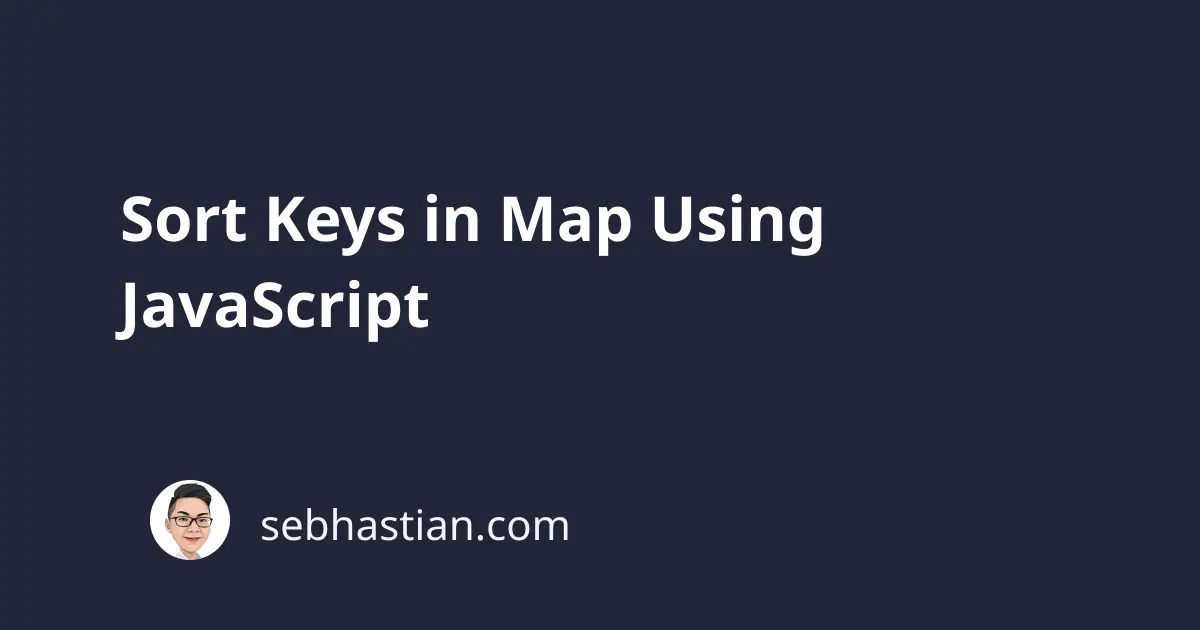
Hello friends! In this article, I’m going to show you how to sort keys in a Map
object using JavaScript.
As you probably know, the Map
object is an unordered iterable object that doesn’t have a sort method.
The object use the order of the key-value insertions to maintain the order of the keys.
If you want to sort a Map object, there are three things you need do to:
- Convert the Map object into an Array
- Call the
sort()
method to sort the array in an ascending or descending order - Convert the Array back into a Map object
Using ES6 syntax, here’s how you can sort a Map object using the shortest amount of code:
const myMap = new Map()
// Set the key-value pair of the Map object
myMap.set('c', "Jack");
myMap.set('a', "Jill");
myMap.set('b', "Drew");
// Order the Map object in ascending order
const ascMap = new Map([...myMap].sort());
// { 'a' => 'Jill', 'b' => 'Drew', 'c' => 'Jack' }
// Order the Map object in descending order
const descMap = new Map([...myMap].sort().reverse());
// { 'c' => 'Jack', 'b' => 'Drew', 'a' => 'Jill' }
In the code above, we create a new Map
object named myMap
and insert some key-value pairs into the object.
To sort the Map
object in ascending order, we use the spread operator to copy the values of Map object into an Array.
Next, we call the sort()
method on the Array
to sort it in ascending order. The entire code is wrapped in a new Map()
construct call to convert the Array object back into Map.
When you want to sort the Map
object in descending order, just add the call to the reverse()
method after you call the sort()
method.
And that’s how you sort a Map
object based on its key values.
I hope this article is useful. Happy Coding! 👋