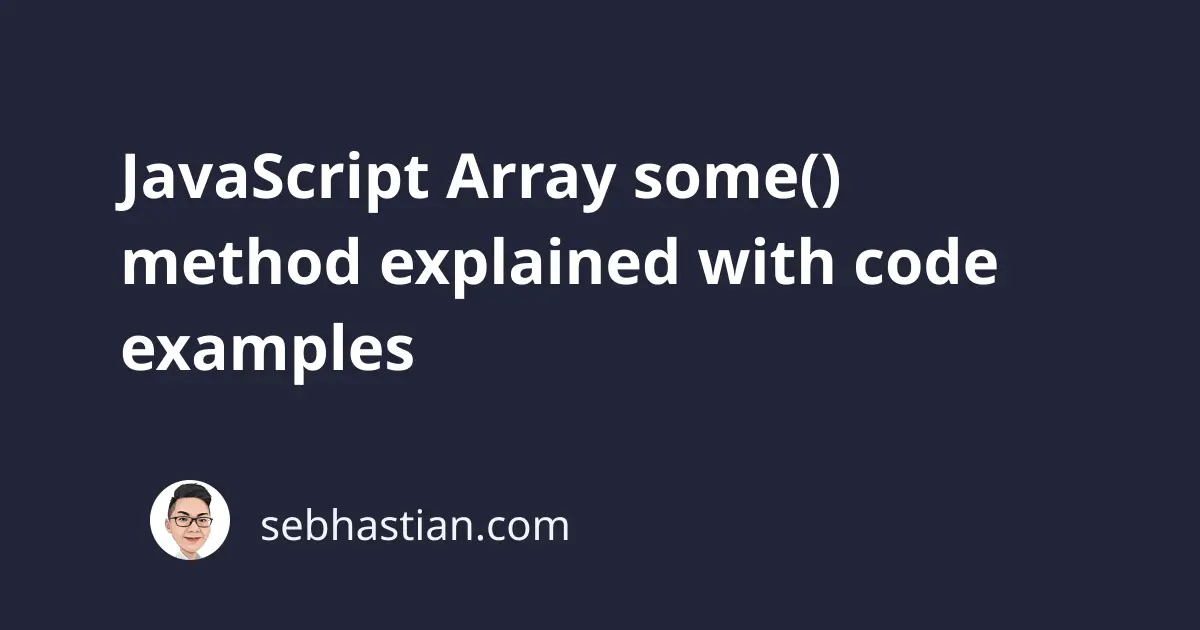
The JavaScript Array some()
method is used to test if an array has at least one element that meets a specific condition.
To use the some()
method, you need to pass the test as a function that will be run against each element in the array.
For example, suppose you want to test if an array has an element that’s greater than 4
. Here’s how you do it:
const numbers = [1, 2, 3, 4, 5];
const testResult = numbers.some(function (element) {
return element > 4;
});
console.log(testResult); // true
The some()
method will pass each element in the array into the function
passed as its argument.
When one of the tested elements returns true
, then some()
will return true
; otherwise, the method returns false
.
You can read the some()
method as check if some elements in this array meet a specific condition, with the condition passed as its argument.
The syntax of the some()
method is as follows:
Array.some(function (element, index, array) {
// function body
}, thisArg);
The function
you passed as an argument to the some()
method will be executed with three arguments:
- The
element
from the array - The
index
of that element - And the
array
object where the method is called from
The thisArg
argument passed after the function
is used to change the context of the this
keyword inside the function body.
When defining the some()
method, only the element
argument is required. The index
, array
, and thisArg
arguments are optional.
Code examples of some() method in action
The following example tests if any element in the array equals a specific value:
const animals = ["dog", "cat", "horse"];
const result = animals.some(function (element) {
return element === "horse";
});
console.log(result); // true
So far, the examples used an anonymous function as the argument for the some()
method, but you can also use an [arrow function( https://sebhastian.com/arrow-function-javascript/ )] or named function as shown below:
[7, 57, 83, 101].some(element => element < 5); // false
[7, 57, 83, 101].some((element, index, array) => {
return element > 5;
}); // true
// Using a named function
function lessThanTen(element, index, array) {
return element < 10;
}
[7, 57, 83, 101].some(lessThanTen); // true
// Using a named arrow function
const greaterThanHundred = element => element > 100;
[7, 57, 83, 101].some(greaterThanHundred); // true
When you execute the some()
method against an empty array, the method will always return false
regardless of the condition you specified.
Consider the following code examples:
[].some(el => el === null); // false
[].some(el => el === undefined); // false
[].some(el => el > 2); // false
Finally, you can also check if a number in your array is within a specific range with some()
method.
The code below tests if any element in the array is between 30
to 50
:
const scores = [25, 55, 72, 85];
const result = scores.some(function (el) {
return el >= 30 && el <= 50;
});
console.log(result); // false
The function
provided as the argument to the some()
method can be as creative as you need it to be.
The only rule is that the function
must return a boolean value (true
or false
)
Now you’ve learned how the some()
method of the JavaScript Array
object works. Nice work! 😉