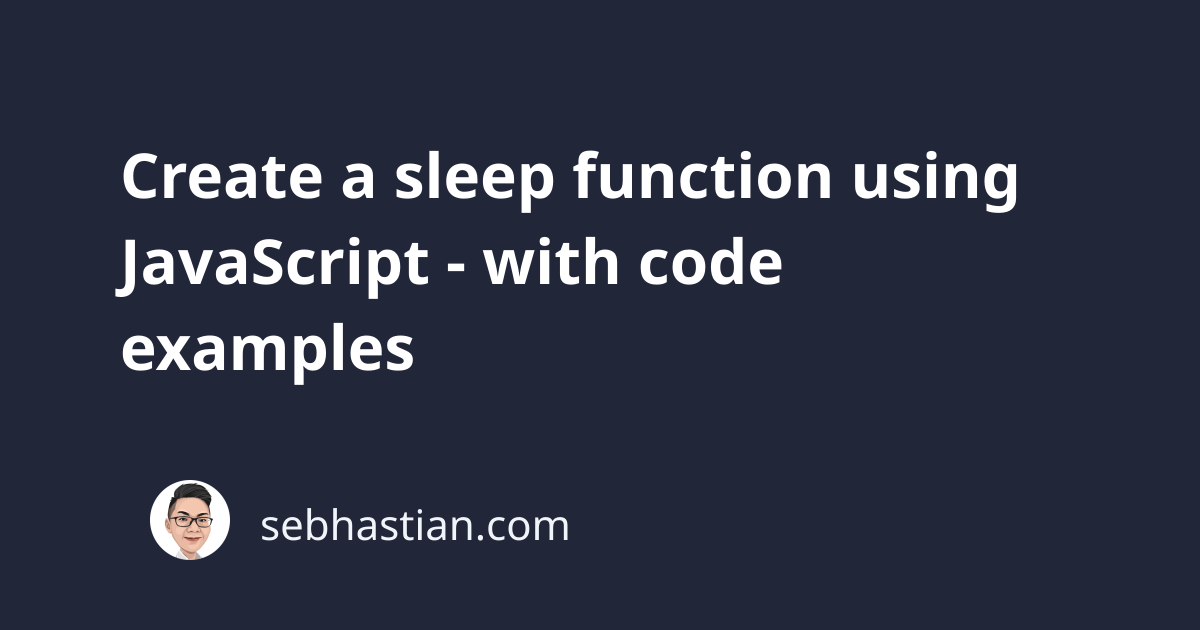
In computer programming, sleep()
is a common function that allows you to delay execution between one piece of operation and another.
For example, the Python programming language allows you to delay code execution using time.sleep()
method like this:
import time
print("Let me sleep for three seconds...")
time.sleep(3)
print("Hello! I just woke up")
When you run the code above, there will be a 3 seconds delay between the first print()
function and the second one.
The output is as follows:
Unfortunately, there’s no native sleep()
function that you can use to delay code execution in JavaScript.
Instead, you have the setTimeout()
function that executes a function after a delay.
The setTimeout()
function works with two parameters:
- The first parameter is the function to execute after a timeout occurs
- The second parameter is the timeout counter measured in milliseconds
The following code example shows how you can recreate the Python script above with JavaScript:
console.log("Let me sleep for three seconds...");
setTimeout(function () {
console.log("Hello! I just woke up");
}, 3000);
The setTimeout()
function is different from a sleep()
function. While sleep()
will cause the machine running the code to stop for a while, setTimeout()
just delays the execution of the function defined as its parameter.
This means JavaScript will continue to execute the code you write below the setTimeout()
function.
The code example below:
console.log("Let me sleep for three seconds...");
setTimeout(function () {
console.log("Hello! I just woke up");
}, 3000);
console.log("Going to the office!");
Will produce the following output:
Let me sleep for three seconds...
Going to the office!
Hello! I just woke up
With the setTimeout()
function, you need to put the code you want to run after the delay inside the callback function.
For some people (including me) the setTimeout()
function looks a bit messy.
The more readable and clean way to delay execution in JavaScript is to create your own sleep()
function.
By using promises and async
/await
features of JavaScript, you can define a sleep()
function as follows:
function sleep(delay) {
return new Promise(resolve => setTimeout(resolve, delay));
}
The sleep()
function above will return a Promise
object that will resolve when the timeout occurs.
You can pass the time in milliseconds as the argument to the function:
console.log("I'm going to sleep...");
sleep(2000).then(function () {
console.log("Been sleeping for two seconds. Any news?");
});
You can also use the async
/await
operator to make the code even cleaner.
JavaScript async
operator is used to mark a function as asynchronous.
This means the function always returns a promise, and you can use the await
operator inside the function body.
The await
operator is added before a function call to make JavaScript delay code execution until the promise is resolved.
Here’s an example code of using the async
/await
operators:
async function sleepTwoSeconds() {
console.log("I'm going to sleep...");
await sleep(2000);
console.log("Been sleeping for two seconds. Any news?");
}
// call the function
sleepTwoSeconds();
Combining the sleep()
function with the async
/await
operator is the cleanest way to delay code execution.
Promises are supported by Node.js since version 0.12 and modern browsers (except Internet Explorer)
The async
/await
operators are available in Node 7 and modern browsers since around 2017.
If you need to support older JavaScript environments, then you need to use Babel to transpile your source code.
Now you’ve learned how to write a sleep()
function using JavaScript. Well done! 😉