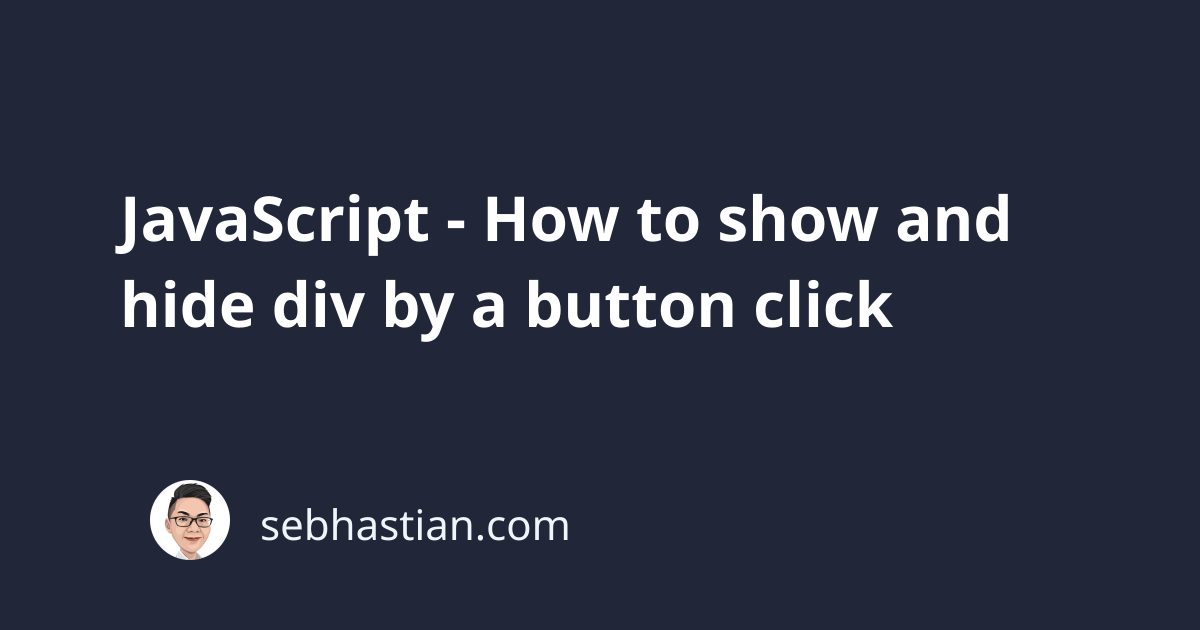
To display or hide a <div>
by a <button>
click, you can add the onclick
event listener to the <button>
element.
The onclick
listener for the button will have a function
that will change the display
attribute of the <div>
from the default value (which is block
) to none
.
For example, suppose you have an HTML <body>
element as follows:
<body>
<div id="first">This is the FIRST div</div>
<div id="second">This is the SECOND div</div>
<div id="third">This is the THIRD div</div>
<button id="toggle">Hide THIRD div</button>
</body>
The <button>
element above is created to hide or show the <div id="third">
element on click.
You need to add the onclick
event listener to the <button>
element like this:
const targetDiv = document.getElementById("third");
const btn = document.getElementById("toggle");
btn.onclick = function () {
if (targetDiv.style.display !== "none") {
targetDiv.style.display = "none";
} else {
targetDiv.style.display = "block";
}
};
The HTML will be rendered as if the <div>
element never existed by setting the display
attribute to none
.
When you click the <button>
element again, the display
attribute will be set back to block
, so the <div>
will be rendered back in the HTML page.
Since this solution is using JavaScript API native to the browser, you don’t need to install any JavaScript libraries like jQuery.
You can add the JavaScript code to your HTML <body>
tag using the <script>
tag as follows:
<body>
<div id="first">This is the FIRST div</div>
<div id="second">This is the SECOND div</div>
<div id="third">This is the THIRD div</div>
<button id="toggle">Hide THIRD div</button>
<script>
const targetDiv = document.getElementById("third");
const btn = document.getElementById("toggle");
btn.onclick = function () {
if (targetDiv.style.display !== "none") {
targetDiv.style.display = "none";
} else {
targetDiv.style.display = "block";
}
};
</script>
</body>
Feel free to use and modify the code above in your project.
I hope this tutorial has been useful for you. 👍