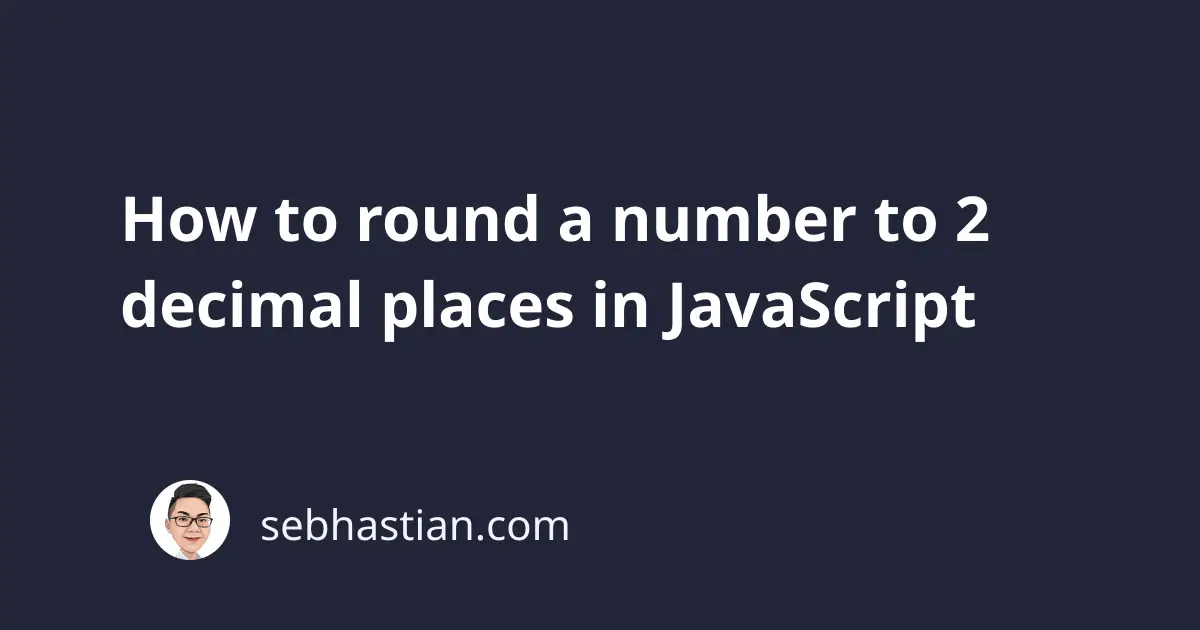
To round a number to 2 decimal places, you need to call the toFixed(2)
method on the value you wish to round.
For example, here you can see me rounding a variable named myNum
:
let myNum = 23.9999999;
// round to 2 decimal places
let newNum = myNum.toFixed(2);
console.log(newNum); // 24.00
The toFixed(2)
method will parse the number and return a string representing the number. The argument you passed to the method will be the number of decimal places presented on the number.
Keep in mind that the method returns a string. If you want to further operate on the number, you need to convert the returned value to a float using the parseFloat()
function:
let myNum = 23.2519999;
// round to 2 decimal places
let newNum = parseFloat(myNum.toFixed(2));
console.log(newNum); // 23.25
// perform additional
console.log(newNum + 1.92); // 25.17
If you don’t call the parseFloat()
function and you try to perform an addition, JavaScript will concatenate the numbers instead of adding them together.
Now you know how to round a number to 2 decimal places in JavaScript. See you in other tutorials!