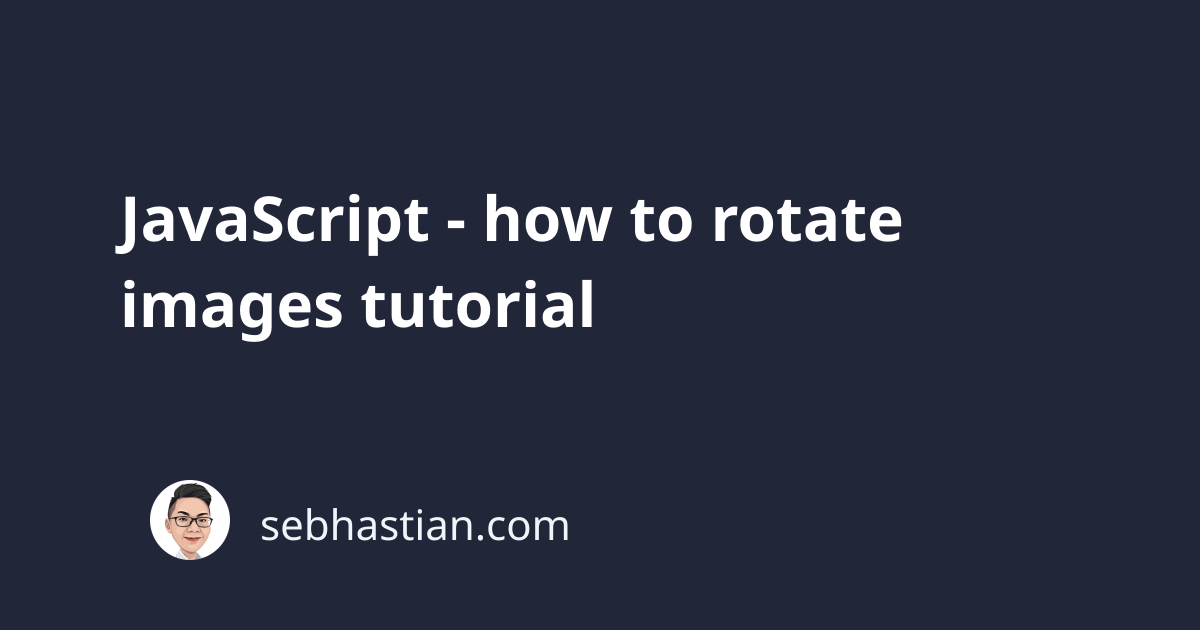
When you need to rotate images using JavaScript, you need to add the transform: rotate(x)
CSS style to the image element you want to rotate.
For example, let’s say you have the following <img>
tag in your project:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript rotate images</title>
</head>
<body>
<img id="img" src="https://via.placeholder.com/350x150.png" />
</body>
</html>
To rotate the image, you can select the element using document.querySelector('#img')
and then append the .style.transform
property to the element. The rotate
property accepts the circular angle parameter measured in 360 degrees.
The following JavaScript code will rotate the image by 90 degrees:
document.querySelector("#img").style.transform = "rotate(90deg)";
The transform: rotate(x)
style will be added to your HTML <img>
tag as follows:
<body>
<img
id="img"
src="https://via.placeholder.com/350x150.png"
style="transform: rotate(90deg);"
/>
</body>
It’s up to you how many degrees you want to rotate the <img>
. To turn the image upside down, you can use rotate(360deg)
property:
document.querySelector("#img").style.transform = "rotate(360deg)";
Rotate an image with a button click
If you need to rotate the image when a button is clicked, you can create a JavaScript function to rotate the image. Then, you need to assign that function to the onclick
attribute of the button:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript rotate images</title>
</head>
<body>
<img id="img" src="https://via.placeholder.com/350x150.png" />
<button onClick="rotateImg()">Rotate Image</button>
<script>
function rotateImg() {
document.querySelector("#img").style.transform = "rotate(90deg)";
}
</script>
</body>
</html>
You can also further rotate the image by 90 degrees each time the button is clicked by first creating a variable that holds the rotation angle.
Take a look at the following example:
<body>
<img id="img" src="https://via.placeholder.com/350x150.png" />
<button onClick="rotateImg()">Rotate Image</button>
<script>
let rotation = 0;
function rotateImg() {
rotation += 90; // add 90 degrees, you can change this as you want
if (rotation === 360) {
// 360 means rotate back to 0
rotation = 0;
}
document.querySelector("#img").style.transform = `rotate(${rotation}deg)`;
}
</script>
</body>
Finally, you can rotate the image counter clockwise by adding a negative number instead of a positive. The following code shows how you can rotate the image -45 degrees:
document.querySelector("#img").style.transform = "rotate(-45deg)";
And that’s how you can rotate images using JavaScript. Feel free to modify the code as you see fit.