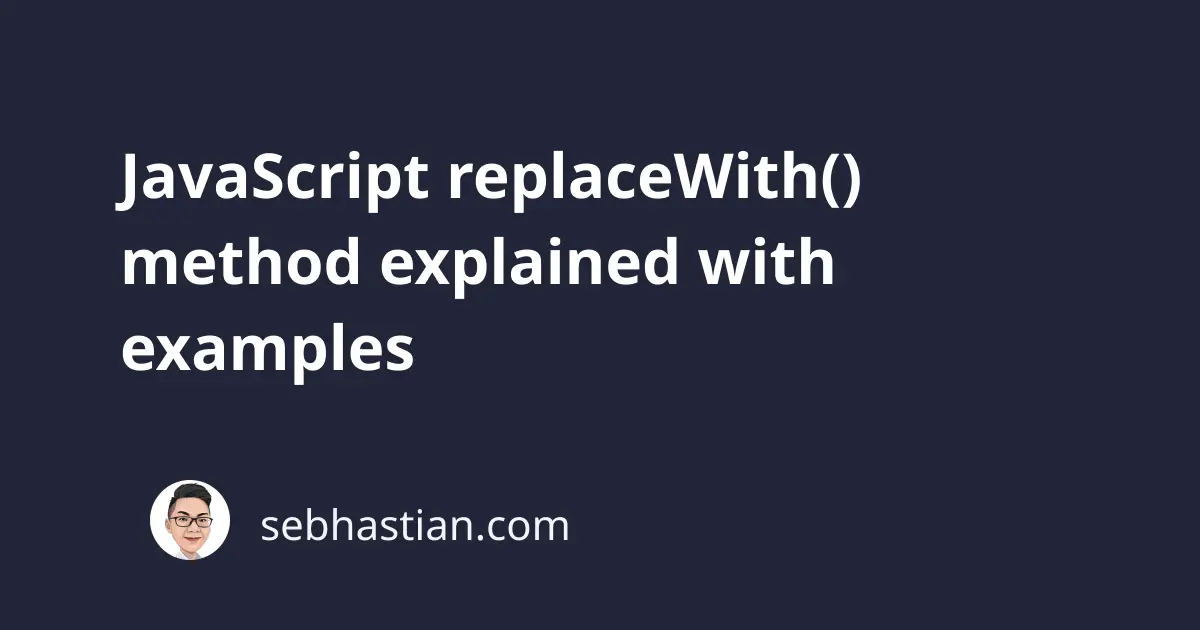
The JavaScript replaceWith()
method is a method of the Element
object that allows you to replace the Element
with the argument passed into the method.
This method accepts either a set of Node
element(s) or a Text
string.
When you pass a Node
into the method, you will replace the Element
where you call this method with that Node
.
In the following example, the <p>
element is replaced with a <span>
element:
const div = document.createElement("div");
const p = document.createElement("p");
const header = document.createElement("h1");
div.appendChild(p);
p.appendChild(header);
console.log(div);
/* <div>
<p>
<h1></h1>
</p>
</div>
*/
const span = document.createElement("span");
p.replaceWith(span);
console.log(div);
/* <div>
<span></span>
</div>
*/
On the other hand, JavaScript will add a string
you pass into the method as a Text
node.
In the example below, the <p>
element is replaced with Hello World!
text:
const div = document.createElement("div");
const p = document.createElement("p");
div.appendChild(p);
console.log(div);
/* <div>
<p></p>
</div>
*/
p.replaceWith("Hello World!");
console.log(div);
/* <div>
Hello World!
</div>
*/
Even when you pass a string
of HTML tags, the replaceWith()
method will consider it as a Text
node instead of a valid HTML tag node.
Consider the following example:
const div = document.createElement("div");
const p = document.createElement("p");
div.appendChild(p);
console.log(div);
/* <div>
<p></p>
</div>
*/
p.replaceWith("<h1></h1>");
console.log(div);
/* <div>
<h1></h1>
</div>
*/
As you can see from the result of the second console log above, the <>
symbol used to create HTML tags are transformed into <
and >
entities.
They are visible on the HTML page from the browser and are not considered valid HTML tags.
To actually pass a Node
object, you need to use the JavaScript document.createElement()
method to create one.
Or if you already have the HTML tags present on the page, you can fetch them and put them in the desired location.
For example, suppose you have an HTML page structured as follows:
<div id="wrapper">
<p id="paragraph">
<span id="span"></span>
</p>
<ul id="list">
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
</ul>
</div>
You can replace the <p>
tag with the <ul>
tag by fetching the elements using their id
attribute first:
const p = document.getElementById("paragraph");
const list = document.getElementById("list");
p.replaceWith(list);
The new HTML structure will be as shown below:
<div id="wrapper">
<ul id="list">
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
</ul>
</div>
The replaceWith()
method can replace the Element
with a Text
node or a set of Node
element objects.
You can create the Node
using document.createElement()
or fetch one from the existing DOM node tree.
And that’s how the JavaScript replaceWith()
method works.
Keep in mind that the native document
object replaceWith()
method is a bit different from the jQuery replaceWith()
method.
The jQuery replaceWith()
method returns the original Element
where you call the method from. Aside from that, both method works the same way.
Good work on learning about JavaScript replaceWith()
method. 👍