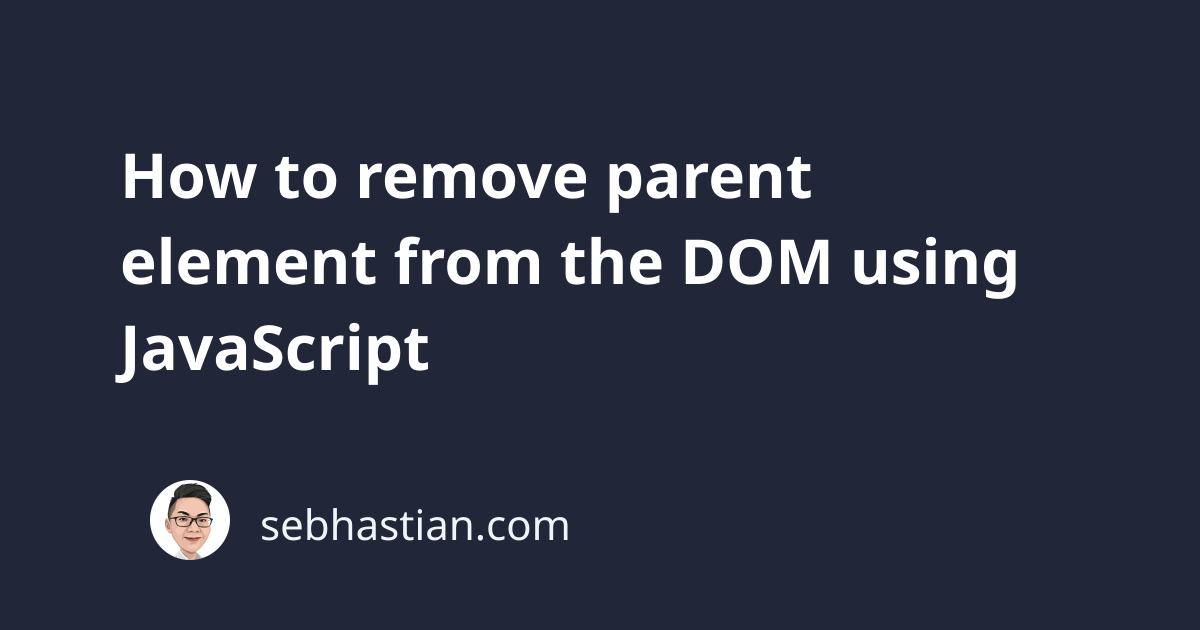
In this post, I will help you learn how to remove parent element from the DOM tree using JavaScript.
Each Element
object has the parentElement
property that you can use to access the parent element of that object.
To remove the parent element of a child element, you can call the remove()
method on the parentElement
object.
Here are the steps to remove a parent element:
- Use
document.getElementById()
or any other selector methods to get the child element. - Get the parent element by accessing the
parentElement
property - Call the
remove()
method on the parent element
Let’s see an example. Suppose you have an HTML document as follows:
<!DOCTYPE html>
<html lang="en">
<head>
<title>sebhastian.com</title>
<meta charset="UTF-8" />
</head>
<body>
<div>
<div id="child">Child 1</div>
</div>
</body>
</html>
Next, you want to remove the <div>
parent from the div that has the id
of child
.
Here’s the JavaScript code you need to run:
document.getElementById("child").parentElement.remove();
And that’s enough to remove the parent element, leaving the <body>
tag empty:
<body></body>
Remove parent element on click
If you want to remove the parent element on click, you need to put the code to remove the element inside a function, then call that function when the button is clicked.
In the following example, a button with an onclick
attribute is added to the HTML document:
<!DOCTYPE html>
<html lang="en">
<head>
<title>sebhastian.com</title>
<meta charset="UTF-8" />
</head>
<body>
<div>
<div id="child">Child 1</div>
</div>
<button onclick="removeParent()">Remove parent element</button>
<script></script>
</body>
</html>
The button will call the removeParent()
function when clicked. You need to define the function inside the <script>
tag as follows:
<script>
function removeParent() {
document.getElementById("child").parentElement.remove();
}
</script>
Open the HTML document in the browser, and click the button to remove the parent element.
The HTML output should be as shown below:
<!DOCTYPE html>
<html lang="en">
<head>
<title>sebhastian.com</title>
<meta charset="UTF-8" />
</head>
<body>
<button onclick="removeParent()">Remove parent element</button>
<script>
...
</script>
</body>
</html>
As you can see the parent <div>
element is removed from the document.
Remove parent element without removing the child
If you want to remove the parent element without removing the child element, then you need to use the replaceWith()
method instead of the remove()
method.
The replaceWith()
method is used to replace the Element
object from which you called this method with another element.
To preserve the child element, use replaceWith()
to replace the parent element with the child:
let childEl = document.getElementById("child");
childEl.parentElement.replaceWith(childElement);
The <div>
parent element should be replaced with the child element, so the HTML document looks like this:
<body>
<div id="child">Child 1</div>
</body>
Here, the child element is not removed from the DOM tree.
Remove class from parent element javascript
If you want to remove the class
attribute from a parent element, then you can use the classList.remove()
method on the parent element.
Suppose you have an HTML <body>
tag with the following contents:
<body>
<div class="text-red bold">
<div id="child">Child 1</div>
</div>
</body>
To remove the class text-red
from the parent element, run the following code:
let childEl = document.getElementById("child");
childEl.parentElement.classList.remove("text-red");
If you want to remove the whole class
attribute instead of a specific entry, use the removeAttribute()
method instead:
let childEl = document.getElementById("child");
childEl.parentElement.removeAttribute("class");
When calling classList.remove()
method, you need to specify the specific class you want to remove.
When calling the removeAttribute()
method, specify the class
attribute to remove it from the element.
And that’s how you remove a parent element or its class from the HTML document.
I hope this tutorial is useful. Happy coding and I’ll see you next time! 👋