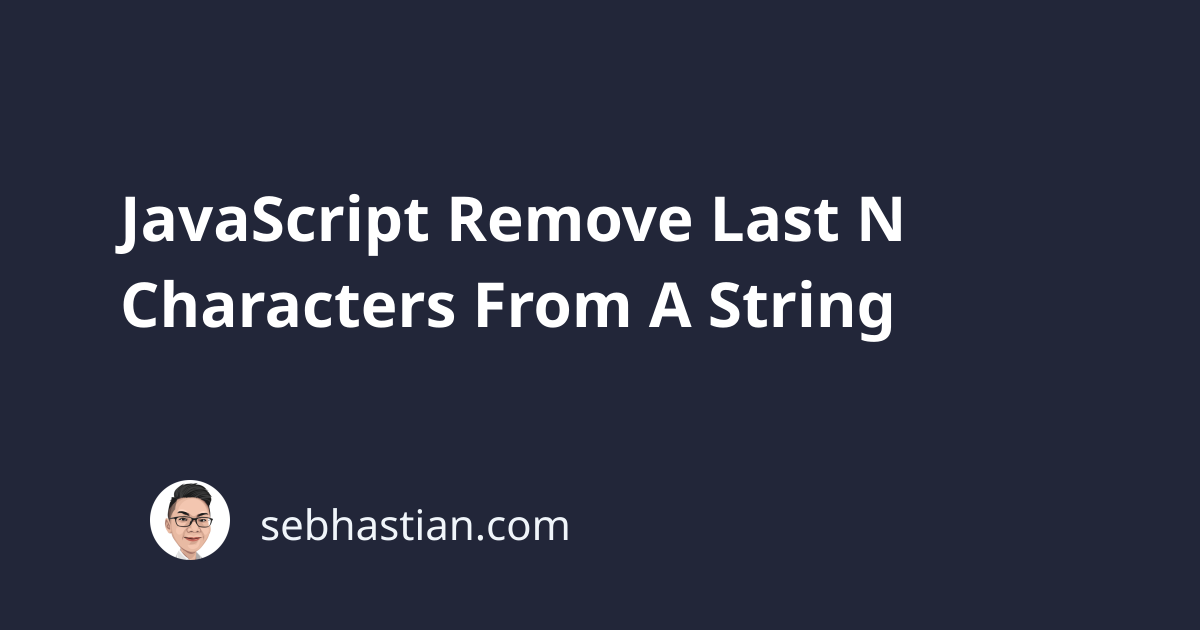
There are two easy ways you can remove the last N characters from a string:
- Using the
slice()
method - Using the
substring()
method - Using the
substr()
method
This article will show you how to use both methods in practice. Let’s see how these work.
1. Using the slice() method
The slice()
method can be used to remove the last N characters from a string. You need to pass the number 0
as the start index and -N
as the end index.
When you specify a negative number as the end index, the slice()
method will chop the string from the end instead of the beginning.
For example, here’s how I remove the last 3 characters from the name
string:
const name = 'Nathan';
// Remove the last 3 characters from a string
const remove3 = name.slice(0, -3);
console.log(remove3); // Nat
Here, you can see that the last 3 characters ‘han’ was removed from the string ‘Nathan’. You can adjust the second argument to fit your needs. If you need to remove 1 character, then pass -1
as the second argument.
2. Using the substring()
method
The substring()
method is another method you can use to remove the last N characters from a string.
Like the slice()
method, the substring()
method accepts start and end indices as its parameters. But instead of passing a negative number, you need to subtract the N characters from the length
property of the string:
const name = 'Nathan';
// Remove the last 3 characters from a string
const remove3 = name.substring(0, name.length-3);
console.log(remove3); // Nat
The reason you need to pass the length
subtracted by N characters is because substring()
treats a negative number as zero.
If you pass zero as both the start and end indices, then you’ll get an empty string returned by the method.
3. Using the substr()
method
Another method you can use to remove last N characters from a string is the substr()
method, which works in a similar manner to the substring()
method:
const name = 'Nathan';
const remove3 = name.substr(0, name.length-3);
console.log(remove3); // Nat
However, note that this method is deprecated because it’s not part of the ECMAScript standard. Some browsers might have removed this function completely. To prevent breaking your web application, never use this method again.
Conclusion
To remove the last N characters from a JavaScript string, use either the slice()
or substring()
method.
I hope this article helps. Happy coding and see you in other articles! 👍