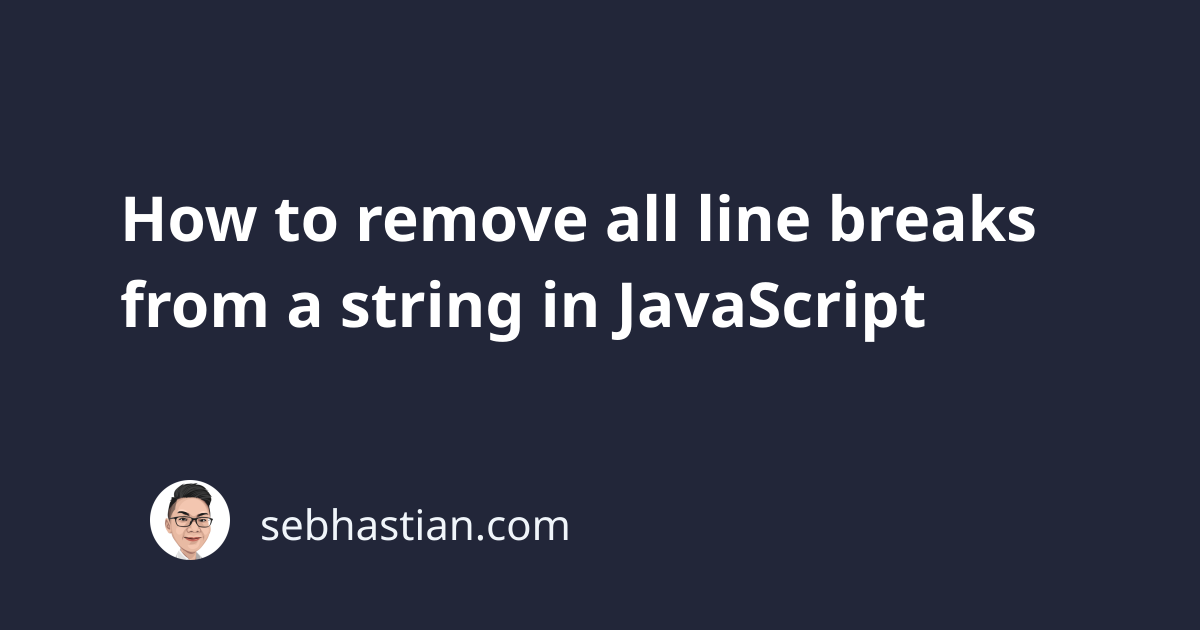
To remove all line breaks from a string in JavaScript, you need to use the String.replace()
method and pass a regular expression pattern to catch all line breaks.
You can then remove the line breaks by passing an empty string "" to replace any matches. See the example below:
let myText = "\rHello \nWorld!\r\n";
myText = myText.replace(/(\r\n|\n|\r)/gm, "");
console.log(myText) // Hello World!
There are three different line break characters depending on the Operating System used on your computer. A Windows computer uses \r\n
, Linux just uses \n
and macOS uses \r
.
The regular expression (\r\n|\n|\r)
will catch all 3 line break characters from your string.
The gm
option means global
and multiline
, and they are added to catch all matching patterns in your string.
The regular expression can also be optimized as /\r?\n|\r/
:
let myText = "\rHello \nWorld!\r\n";
myText = myText.replace(/\r?\n|\r/gm, "");
console.log(myText) // Hello World!
Here, the question mark ?
is used to check if the /r
token is matched before looking for the \n
token. This is used to look for /r/n
and /n
tokens.
The second alternative |/r
is added to find the /r
token. This regular expression is faster than the first one, which tests three different alternatives.
Remove line breaks from the beginning and end of the string
If you want to remove line breaks only from the beginning and end of the string, you can use the String.trim()
method as a faster alternative.
You can call the method without passing any argument as shown below:
let myText = "\rHello \nWorld!\r\n";
myText = myText.trim();
console.log(myText) // Hello \nWorld!
Here, you can see that the \n
in the middle of the string is ignored. The trim()
method only looks for white spaces and line breaks at the beginning and the end of a string.
If you want to use the replace()
method instead of trim()
, then you need to specify a regular expression /^\s+|\s+$/
like this:
let myText = "\rHello \nWorld!\r\n";
myText = myText.replace(/^\s+|\s+$/gm, "");
console.log(myText) // Hello World!
The caret ^
symbol matches the beginning of the string, and the $
symbol for the end of the string.
The \s+
pattern matches all new line, space, and tab characters. You then replace them with an empty string.
Conclusion
To remove all line breaks from a string in JavaScript, you can use the String replace()
method and pass a regular expression to replace all new lines with an empty string.
If you want to remove line breaks only from the beginning and the end of a string, use the trim()
method to make it easier and faster.
I hope this tutorial is useful. Happy coding! 🙌