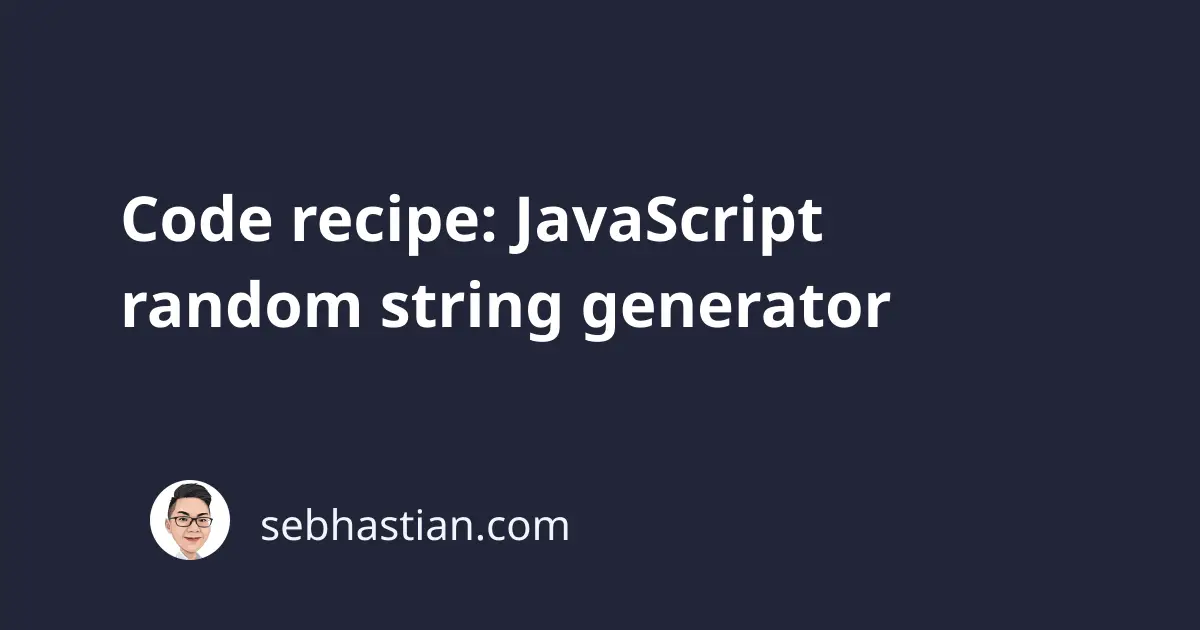
There are several ways to generate a random string with JavaScript, depending on the requirements you need to meet.
You can generate both random alphanumeric string, which is a combination of letter and numbers, and you can also generate a letter-only string. This tutorial will help you to learn both.
JavaScript random alphanumeric string
You can generate a random alphanumeric string with JavaScript by first creating a variable that contains all the characters you want to include in the random string.
For example, the following characters
variable contains the numbers 0
to 9
and letters A
to Z
both in upper and lower cases:
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
Once you settled on the characters you want to include in your random string, you need to determine the length
of the random string. For this tutorial, let’s generate a random string with 9 characters :
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const length = 9;
Now you need to create another variable that contains the random string. Just initialize the variable with an empty string like this:
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const length = 9;
let randomStr = "";
It’s time to generate the random string. Write a for
loop that will iterate as long as the value of length
variable as shown below:
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const length = 9;
let randomStr = "";
for (let i = 0; i < length; i++) {}
In each iteration, you need to generate a random number between 0
and characters.length - 1
. You will use this number to pick a character from the characters
variable that you will add to the randomStr
variable.
The code inside the for loop will be as follows:
const characters =
"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
const length = 9;
let randomStr = "";
for (let i = 0; i < length; i++) {
const randomNum = Math.floor(Math.random() * characters.length);
randomStr += characters[randomNum];
}
console.log(randomStr); // will be different with each execution
And that’s how you generate an alphanumeric random string using JavaScript. You can change the length
variable value to change how many characters you have in the random string.
JavaScript random letter-only string
To generate a letter-only string, you can use the same method as generating a random alphanumeric string above. You just need to remove the numbers 0
to 9
from the characters
variable:
const characters = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
const length = 9;
let randomStr = "";
for (let i = 0; i < length; i++) {
const randomNum = Math.floor(Math.random() * characters.length);
randomStr += characters[randomNum];
}
console.log(randomStr); // will be different with each execution
And that’s how you can generate a random letter-only string 😉