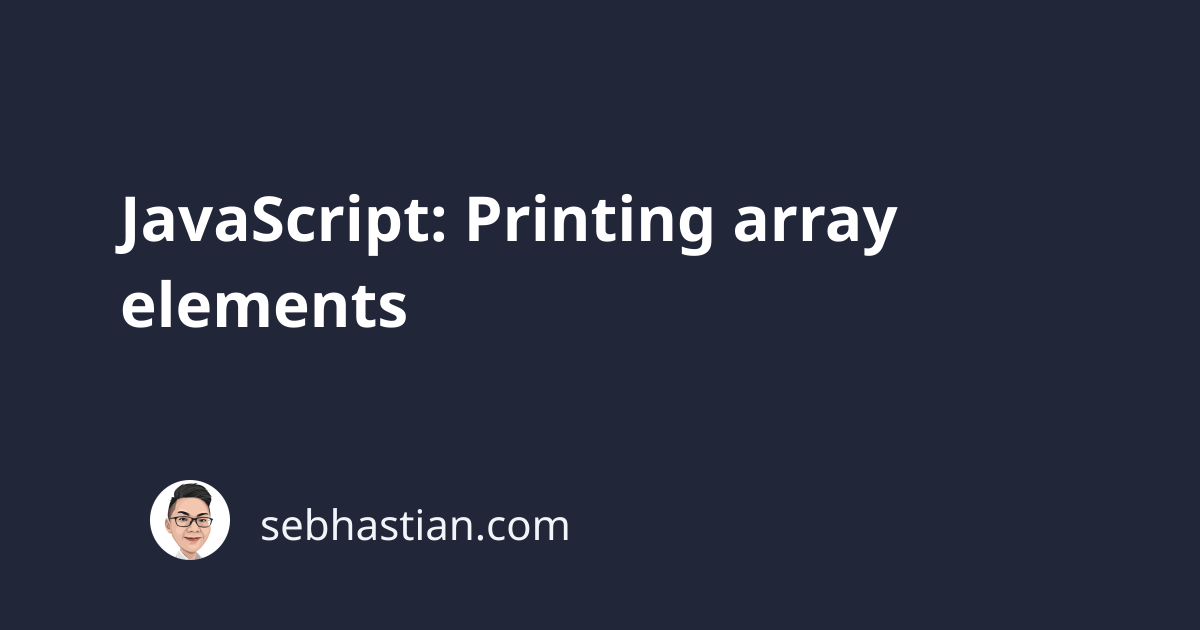
When you need to print array elements, you can loop over the array and print each element to the console as follows:
let arr = ["Jack", "John", "James"];
for(let i = 0; i < arr.length; i++){
console.log(arr[i]);
}
The array elements will be printed to the console as follows:
> "Jack"
> "John"
> "James"
Now sometimes you want the elements to be printed in a nice format to a web page. You can do so by assigning the array as the innerHTML
property of an element.
Here’s an example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript print array</title>
</head>
<body>
<pre id="arrPrint"></pre>
<script>
let arr = ["Jack", "John", "James"];
document.getElementById("arrPrint").innerHTML = arr;
</script>
</body>
</html>
But when you have an array of objects, the innerHTML
of the element will be set as [object Object]
instead of the array elements. To print an array of objects properly, you need to format the array as a JSON string using JSON.stringify()
method and attach the string to a <pre>
tag in your HTML page.
Try the following example:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>JavaScript print array</title>
</head>
<body>
<pre id="arrPrint"></pre>
<script>
let arr = [{name: "Jack"}, {name: "John"}, {name: "James"}];
document.getElementById("arrPrint").innerHTML = JSON.stringify(arr, null, 2);
</script>
</body>
</html>
Your array will be printed as follows:
[
{
"name": "Jack"
},
{
"name": "John"
},
{
"name": "James"
}
]
And that’s how you can print JavaScript array elements to the web page.