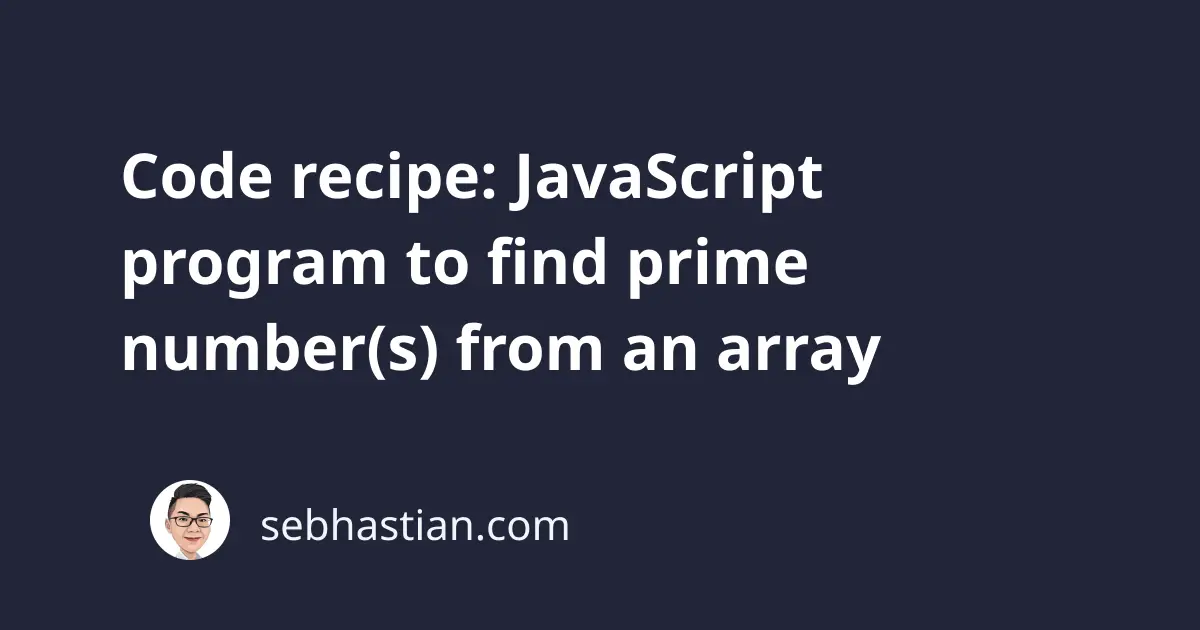
A prime number is a number that’s only divisible by two numbers: one and itself. Some examples of prime numbers are 2, 3, 5, 7, 11, and 13.
This tutorial will help you to write a JavaScript program that can find prime number(s) from an array. But first, let’s write a function to find out if a number is a prime number.
Writing a function to check for a prime number
To find prime numbers using a JavaScript program, you can use a combination of the for
loop and the conditional if..else
statement.
First, create a function that accepts a number
to check whether it’s a prime number or not. Let’s call this function checkPrime()
:
function checkPrime(number) {}
Then, create an if
block to check if the number value equals to 1
or lower. Return false
if it is because 0, 1, and negative numbers are not prime numbers.
function checkPrime(number) {
if (number <= 1) {
return false;
}
}
Next, create an else
block and initiate the for
loop inside it. The for loop will be initialized from the number 2
and will continue to iterate until it’s equal to the number
value:
function checkPrime(number) {
if (number <= 1) {
return false;
} else {
for (let i = 2; i < number; i++) {
// TODO: write the code to check for prime numbers
}
}
}
Inside the for
loop, create another if
block that checks whether the number
value is divisible by the value of i
and return false
if it is. This is because the number
must not be divisible by any other number except one and itself:
function checkPrime(number) {
if (number <= 1) {
return false;
} else {
for (let i = 2; i < number; i++) {
if (number % i == 0) {
return false;
}
}
}
}
The return
keyword will stop the function
execution and send the value false
to the caller. You can store this value in a variable as follows:
const isPrime = checkPrime(9);
console.log(isPrime); // false
Finally, return true
just below the for
block that you’ve created to let the caller know that the number
is a prime number:
for (let i = 2; i < number; i++) {
if (number % i == 0) {
return false;
}
}
return true;
This is because if the for
loop finished without returning false
, it means the number
value can’t be divided by any number between 1 and itself. In other words, it’s a prime number.
This is why you should return true
to let the function caller know that the number is a prime number.
Here’s the complete source code for you to inspect:
function checkPrime(number) {
if (number <= 1) {
return false;
} else {
for (let i = 2; i < number; i++) {
if (number % i == 0) {
return false;
}
}
return true;
}
}
Your checkPrime()
function is now done. You can test the code by calling the function multiple times with different numbers:
let isPrime;
isPrime = checkPrime(3);
console.log(isPrime); // true
isPrime = checkPrime(12);
console.log(isPrime); // false
isPrime = checkPrime(50);
console.log(isPrime); // false
isPrime = checkPrime(23);
console.log(isPrime); // true
isPrime = checkPrime(0);
console.log(isPrime); // false
Finding prime number(s) from an array of numbers
Now that you have a working checkPrime()
function, you can check if a number is a prime or not from an array of numbers as follows:
let arr = [3, 12, 50, 23, 0];
To check the numbers inside the arr
array above, you need to iterate through the array using the forEach()
method, then call the checkPrime()
function for each element
passed from the callback
function as in the code below:
arr.forEach(function (element) {
const isPrime = checkPrime(element);
if (isPrime) {
console.log(`${element} is a prime number`);
} else {
console.log(`${element} is NOT a prime number`);
}
});
And that’s how you can find prime numbers from an array of numbers. Feel free to use the code as you require it 😉