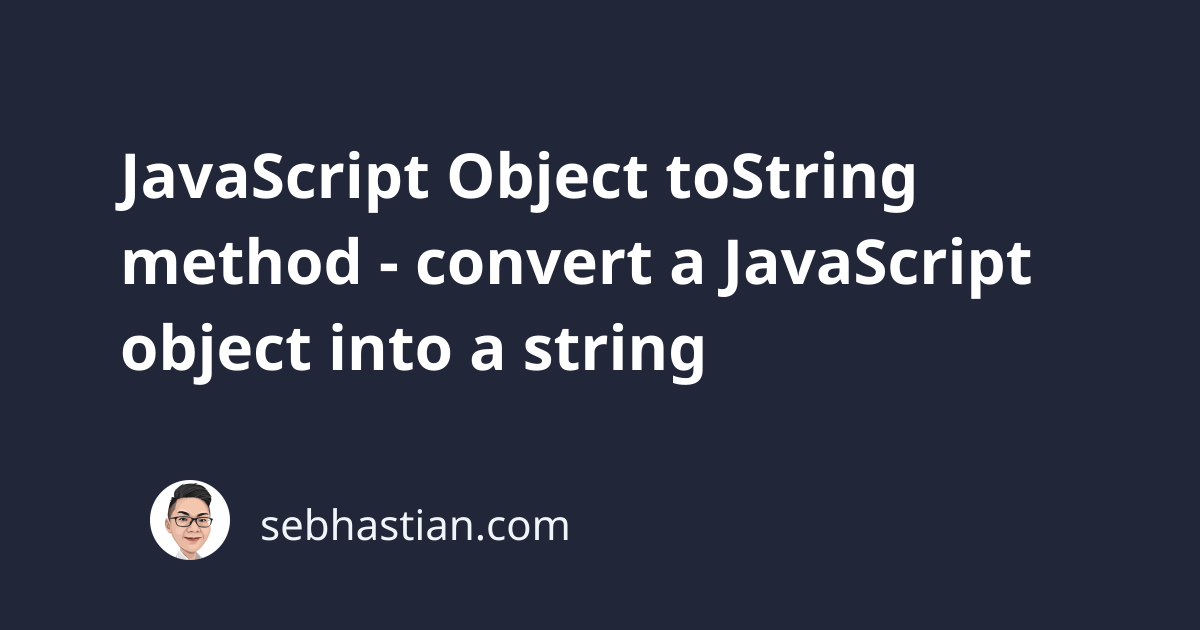
JavaScript Object
class has the toString()
method that returns a string
representing the object where you call the method.
But because an object can have many properties, JavaScript will return an "[object Type]"
string instead of the object keys and properties.
Consider the following code:
const person = {
name: "Nathan",
};
console.log(person.toString());
// [object Object]
Logically, you might expect that the output of the toString()
method above as {"name":"Nathan"}
.
Instead, JavaScript returns a string in the format of "[object Type]"
because it considers the object Type
better represents what the object is.
I do think it’s strange, but this is based on the ECMAScript 15.2.4.2 specification for the toString()
method that says:
Return the String value that is the result of concatenating the three Strings “[object “, class, and “]”.
The same [object Object]
will be returned for objects generated from functions and classes:
class Car {
constructor(name, year) {
this.name = name;
this.year = year;
}
}
const car = new Car("Tesla", 2018);
console.log(car.toString());
// [object Object]
function User(username) {
this.username = username;
}
const user = new User("JohnDoe78");
console.log(user.toString());
// [object Object]
Fortunately, you can override the toString()
method to return unique information about the object:
const person = {
name: "Nathan",
};
person.toString = function () {
return this.name;
};
console.log(person.toString());
// Nathan
The same can be done for JavaScript objects created from functions and classes:
class Car {
constructor(name, year) {
this.name = name;
this.year = year;
}
toString() {
return this.name;
}
}
const car = new Car("Tesla", 2018);
console.log(car.toString());
// Tesla
function User(username) {
this.username = username;
}
User.prototype.toString = function () {
return this.username;
};
const user = new User("JohnDoe78");
console.log(user.toString());
// JohnDoe78
For a class-based object, you add the toString()
method in the class
body.
For a function-based object, you override the function’s prototype.toString
method.
But overriding the toString()
method of each object you define in your source code is very tedious.
Your code will become more complex when you need to make the toString()
method return a dynamic value based on the property key
passed into the method.
Instead of overriding the toString()
method, it’s better to use the JSON.stringify()
method to return a string
representing your object.
Here’s how the JSON.stringify()
method works:
class Car {
constructor(name, year) {
this.name = name;
this.year = year;
}
}
const car = new Car("Tesla", 2018);
console.log(JSON.stringify(car, null, 2));
// {"name":"Tesla","year":2018}
The JSON.stringify()
function converts the object you pass as its argument into a JSON text format.
You can even control the white space used to format the string:
console.log(JSON.stringify(car, null, 2));
// {
// "name": "Tesla",
// "year": 2018
// }
console.log(JSON.stringify(car));
// {"name":"Tesla","year":2018}
The second parameter of the JSON.stringify()
method is used to add a function or an array to manipulate the object before turning it into a string.
For example, you can convert the object name
value to uppercase:
console.log(JSON.stringify(car, function (key, value) {
if (key == "name") {
return value.toUpperCase();
}
return value;
}, 2));
// {
// "name": "TESLA",
// "year": 2018
// }
If you only need to control the white space for the returned string, you can pass null
as the second argument of the stringify()
method.
Now you’ve learned how the JavaScript Object.toString()
method works and how you can represent your objects with more informative strings.
Feel free to use the code in this tutorial for your projects. 😉