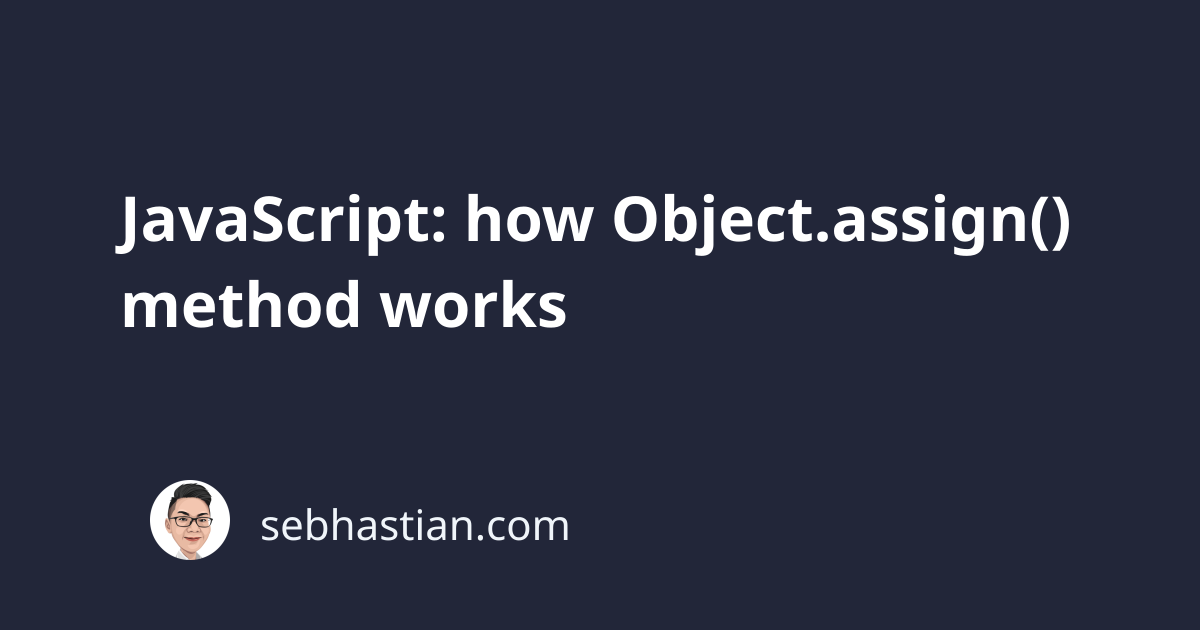
The Object.assign()
method is used to copy all enumerable properties of one or more sources
objects to the target
object. Enumerable properties are properties defined inside the object. They are not properties inherited from other objects.
The following example copies the object user
properties to object person
:
let person = { name: "Nathan" };
let user = { role: "Admin" };
Object.assign(person, user);
console.log(person); // { name: "Nathan", role: "Admin" }
As you can see in the example above, the property role
from object user
is copied to the person
object.
The Object.assign()
method allows you to use as many source
object as you want, but only one target
object. The first object passed as an argument to the assign()
method will be the target
object, while the rest will be the sources
object:
let person = { name: "Nathan" };
let user = { role: "Admin" };
Object.assign(person, user, { age: 28 }, { job: "Web Developer" });
console.log(person); // { name: "Nathan", role: "Admin", age: 28, job: "Web Developer" }
Finally, if the target
object and the source
object has the same property, the property in target
object will be overwritten by the property in source
object. The following example shows how the name
property value in person
object is getting replaced by the source object:
let person = { name: "Nathan" };
let user = { name: "Jack" };
Object.assign(person, user);
console.log(person); // { name: "Jack" }
And that’s how you can use Object.assign()
method to combine two or more JavaScript objects.