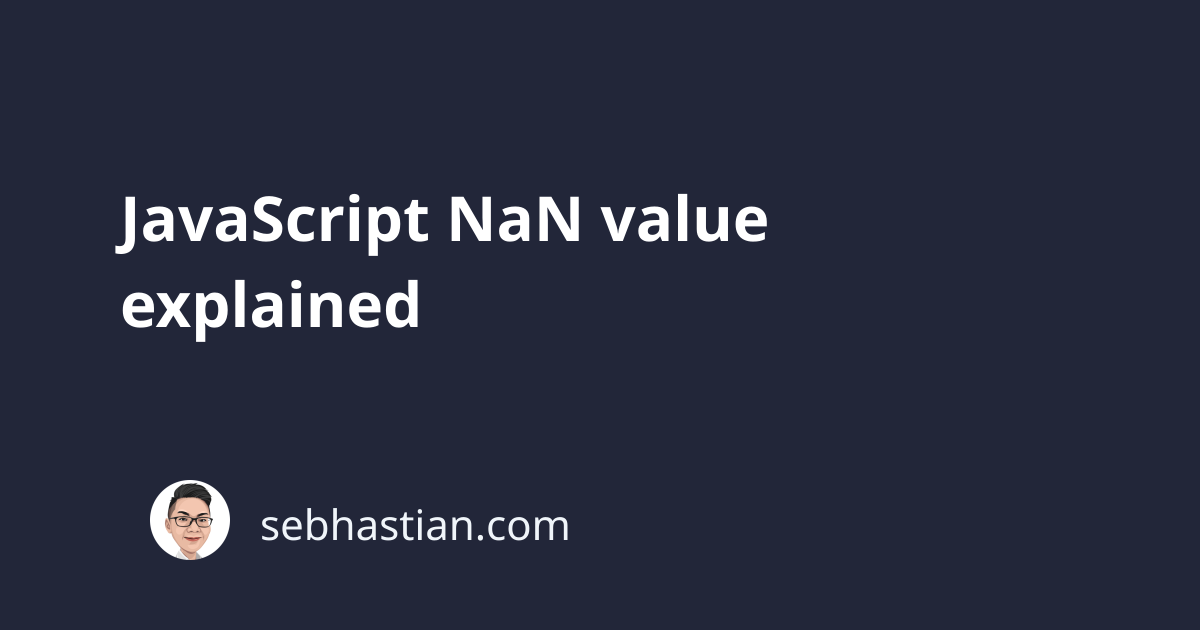
The global property NaN
is a special value that represents an invalid number in JavaScript.
NaN
stands for Not-a-Number, and it can be generated by JavaScript when you perform an operation that can’t produce a valid number.
For example, when you try to create a number from a string
that has no number
representation:
console.log(Number("Z"));
// NaN
console.log(Number("55"));
// 55
The same occurs when you try to parseInt()
or parseFloat()
values that can’t be parsed into a number:
parseInt(undefined); // NaN
parseFloat(); // NaN
In all, there are 5 conditions that will cause JavaScript to return a NaN
value:
- When you parse a value with no number representation like
Number("Z")
- Run a
Math
method that returns an invalid number likeMath.floor()
- Run an arithmetic operation with invalid operands like
7 + undefined
Any arithmetic operation will result in NaN
when you use an invalid value as its operand.
Except for additions between a number and a string, the following operations all return NaN
values:
7 - "asd"; // NaN
3 - [1, 3, 4]; // NaN
9 + undefined; // NaN
5 * "five"; // NaN
7 + "seven"; // 7seven
To check for a NaN
value, you can use the global isNaN()
function provided by JavaScript.
The isNaN
function returns true
when you have a NaN
value:
isNaN(NaN); // true
isNaN(9); // false
isNaN("zxc"); // true
The isNaN()
function is required each time you want to check for a NaN
value.
Using a comparison operator ==
or ===
for NaN
values will always return false
:
NaN == NaN; // false
NaN === NaN; // false
isNaN(1 + undefined); // true
Now you’ve learned how the NaN
value works in JavaScript. Nice work! 👍