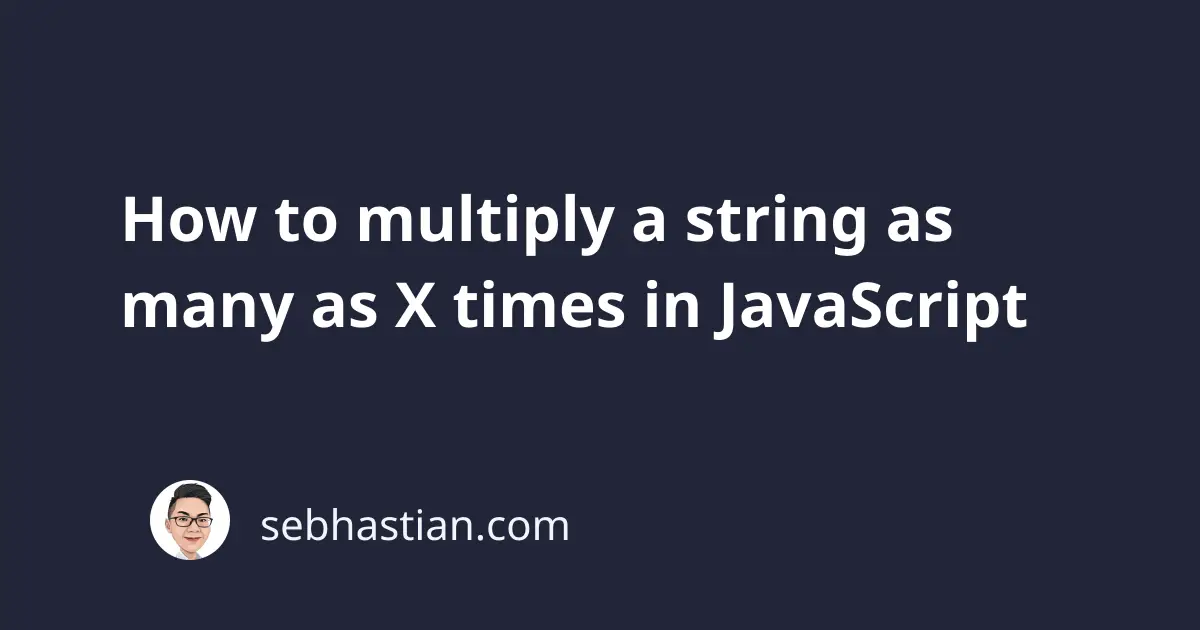
Sometimes, you want to multiply a string using JavaScript as many times as your project requires. For example, you may want to multiply the following string as many as the value stored in multi
variable:
let multi = 3;
let str = "Mary had a little lamb";
There are three ways you can multiply the string above:
- Using the
String.repeat()
method - Using a
for
loop - Using a
while
loop
This article will help you to learn how to use all three methods to multiply the string, starting from using the String.repeat()
method.
Multiply string using repeat() method
The String.repeat()
method will return a new string
value that contains the number of copies of the string concatenated together. You need to pass a number
value to the method that specifies how many copies of the string are required.
For example, to repeat a string two times, pass the number 2
into the repeat()
method:
let str = "abc ";
let multiStr = str.repeat(2);
console.log(str); // "abc "
console.log(multiStr); // "abc abc "
Knowing this, you can easily multiply any string you want:
let multi = 3;
let str = "Mary had a little lamb";
console.log(str.repeat(multi));
// "Mary had a little lambMary had a little lambMary had a little lamb"
But as you can see, the repeat()
method requires you to add a white space at the end of the string, or the words will be joined together without a space. But if you add a white space, then it will also be added to the end of your multiplied string as in the code below:
let multi = 2;
let str = "Little lamb ";
console.log(str.repeat(multi)); // "Little lamb Little lamb "
To remove the white space at the end of the string, you need to use the trimEnd()
method:
let multi = 2;
let str = "Little lamb ";
let multiStr = str.repeat(multi).trimEnd();
console.log(multiStr); // "Little lamb Little lamb"
And with that, the white space is trimmed from the end of the string. Next, let’s look at how to multiply a string using the for
loop.
Multiply string using a for loop
The for
loop allows you to perform a task repeatedly, so you can use it to multiply your string. First, you need to declare an empty string, which will receive the original string inside the loop. You need to pass how many times to iterate and concatenate the string into the conditional expression (the middle one) as in the following example:
let multi = 2;
let str = "Little lamb";
let multiStr = "";
for(let i = 0; i < multi; i++){
multiStr += str
}
console.log(multiStr); // "Little lambLittle lamb"
Still, the for
loop will multiply the string without a white space, so if you need one, you need to add it inside the loop, then use the trimEnd()
method to remove the white space at the end of the string:
let multi = 2;
let str = "Little lamb";
let multiStr = "";
for(let i = 0; i < multi; i++){
multiStr += str
multiStr += " ";
}
multiStr = multiStr.trimEnd();
console.log(multiStr); // "Little lamb Little lamb"
And that’s how you can multiply a string using the for
loop. Let’s look at the final method to multiply a string: the while
loop method.
Multiply a string using a while
loop
You need to concatenate the string inside the while
loop and make sure that the loop is repeated as many times as you require. Take a look at the code below and notice how the while
loop will repeat as many as multi
value times:
let multi = 2;
let str = "Little lamb";
let multiStr = "";
while(multi > 0){
multiStr += str
multiStr += " ";
multi--;
}
multiStr = multiStr.trimEnd();
console.log(multiStr); // "Little lamb Little lamb"
And just like when using the for
loop, you need to add white spaces between the string and remove it from the end of the string using the trimEnd()
method.
And those are the three ways you can multiply a string in JavaScript. The easiest way is to use the repeat()
method, but you may have some programming exercise that requires you to do it manually. If that’s the case, then you can choose between using a for
loop or a while
loop to multiply the string.
Feel free to choose the method you like best.