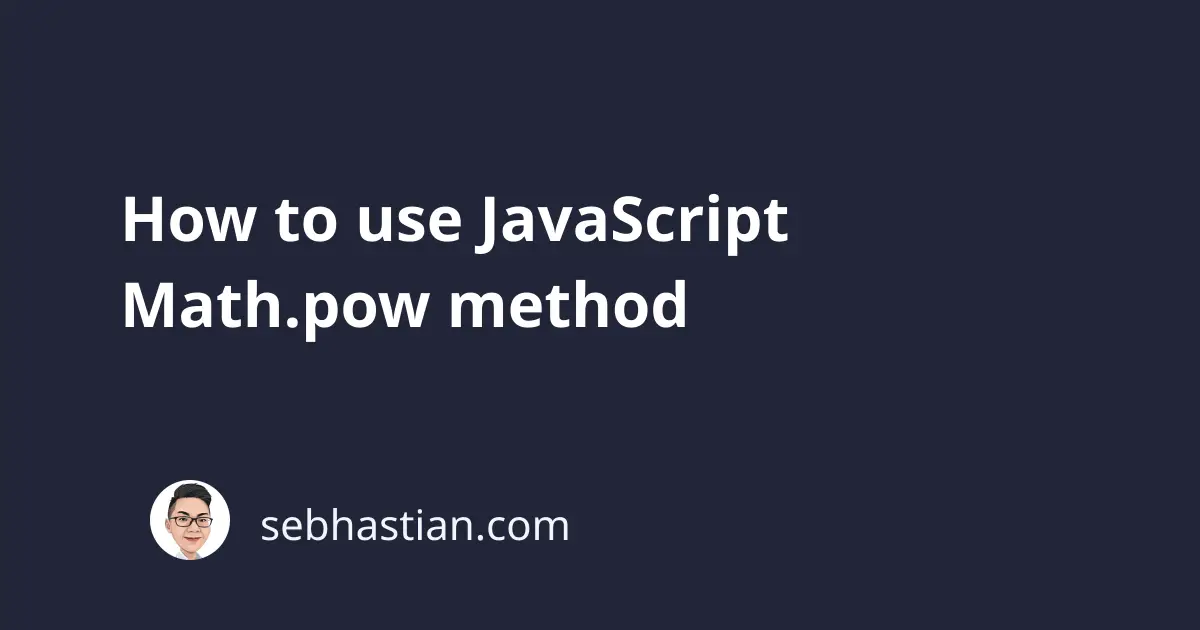
In JavaScript, you can find the exponent value of a number by using the Math.pow()
method, which accepts two parameters:
- The first parameter is the
base
number - The second parameter is the
exponent
number to raise thebase
Here’s an example of 4
to power 3
or 4*4*4
:
let baseNumber = 4;
let exponentNumber = 3;
Math.pow(base, exponent);
// returns 64
When you pass only one parameter, the method will return NaN
:
Math.pow(3);
// returns NaN
You can calculate negative exponents, which will divide the base number instead of multiply it:
Math.pow(8, -2);
// returns 0.015625
When you pass 0
as the exponent
number, the method will always return 1 as value:
Math.pow(7, 0);
Math.pow(0, 0);
Math.pow(0.7, 0);
Math.pow(-23, 0);
Math.pow(-3.2, 0);
// all returns 1
The method will return NaN for any negative base
number to the power of fractional exponent
:
Math.pow(-23, 0.7);
// returns NaN
When you pass any other data types, the method will return NaN
as value:
Math.pow("hello", "world");
// returns NaN