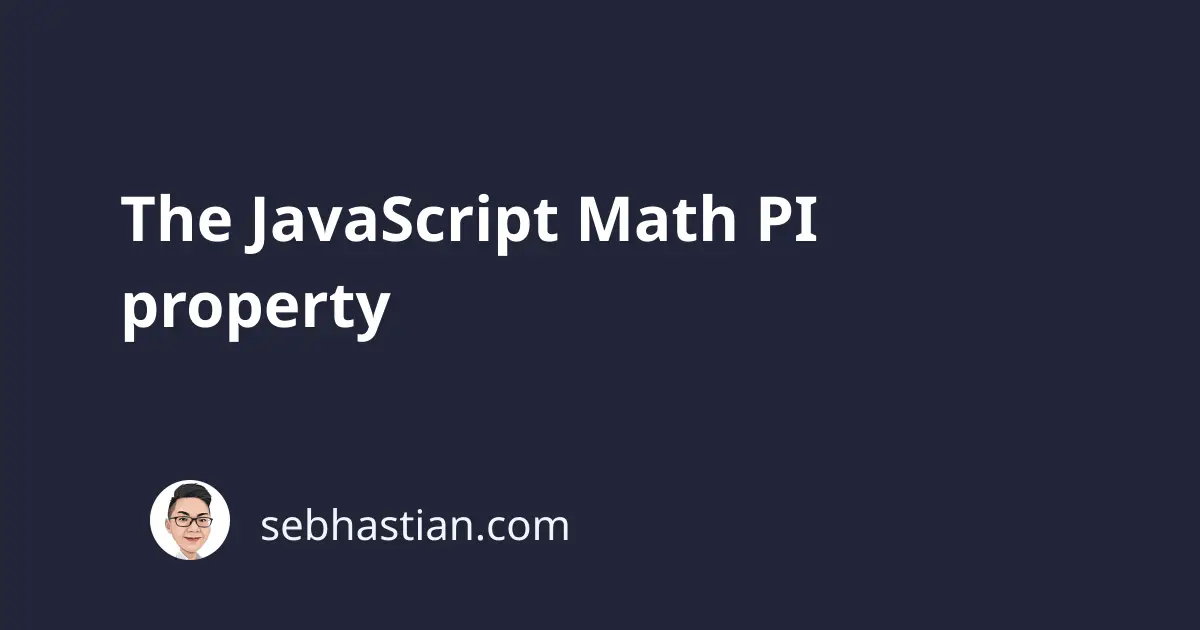
In JavaScript, the mathematical constant PI
is stored in the Math object’s static property:
console.log(Math.PI);
// returns 3.141592653589793
You can use the value of Math.PI
to find the Diameter when you know the Circumference:
// Diameter = Circumference / π
let circumference = 20
let diameter = circumference / Math.PI
You can find the Circumference by multiplying the PI with the Diameter:
// Circumference = π X Diameter
let diameter = 9
let circumference = Math.PI * diameter
Finally, you can calculate the Radius of a circle if you know the Circumference:
// Radius = Circumference X 2π
let circumference = 17;
let radius = circumference * (2 * Math.PI)