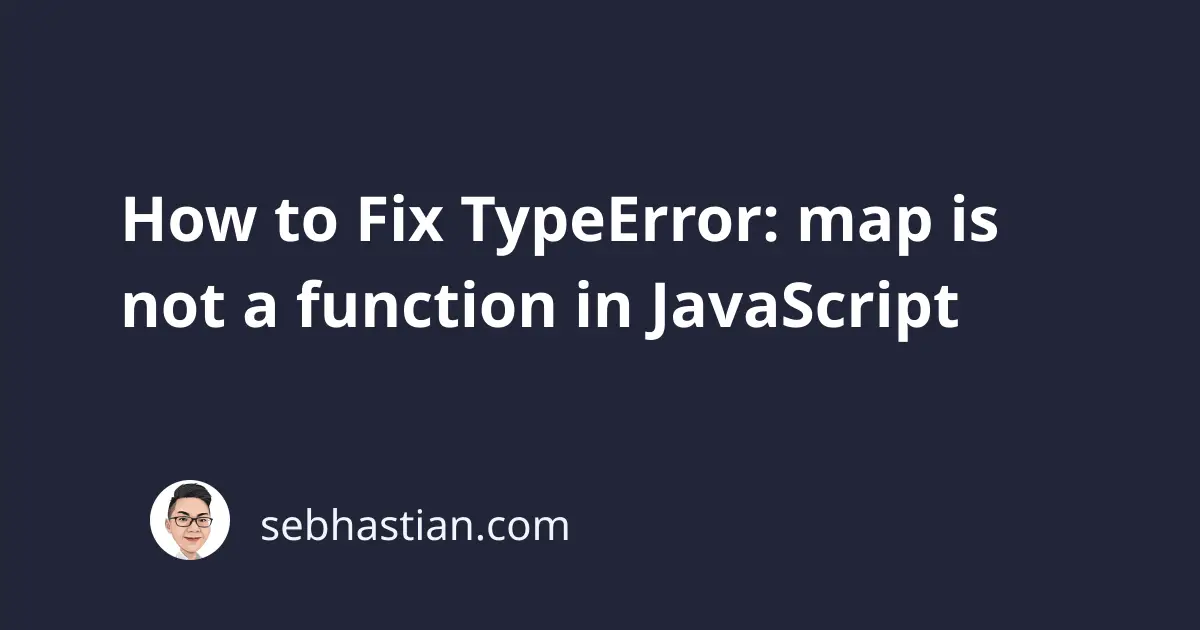
The error message ‘TypeError: map is not a function’ occurs when you call the map()
function from a non-Array object.
The map()
function is a method available only on Array prototype object, so this error might appear when you call the function from a String, an Object, or any other data type in JavaScript.
For example, suppose you call the method from an Object as shown below:
let user = { name: 'Nathan' };
user.map(item => console.log(item));
Because the calling object is not an array, the error is triggered:
TypeError: user.map is not a function
To fix this error, you need to avoid calling the function from a non-Array object. One way to make sure that you have an array is to call the Array.isArray()
method.
The isArray()
method returns a boolean value indicating that the object you passed to the method is an array. This method returns true
only if you pass an array to it, so you can use an if
statement as follows:
let user = { name: 'Nathan' };
if (Array.isArray(user)) {
user.map(item => item + '101');
}
If isArray()
returns false
, the map()
function inside the if
statement won’t be executed, so you prevent the error.
Now if you want to map over an object, you need to turn the object into an array first. If you only need the keys, use the Object.keys()
method. To turn the object values into an array, use the Object.values()
method.
When you need both keys and values, use the Object.entries()
method which converts both into a two-dimensional array.
The following example shows how you can use the Object.keys()
method before mapping the array keys:
let user = { name: 'Nathan', age: 30 };
let keys = Object.keys(user); // [ 'name', 'age' ]
let mapResult = keys.map(item => item + '101');
console.log(mapResult); // [ 'name101', 'age101' ]
To map over the array values, change the function called from Object.keys()
to Object.values()
:
let user = { name: 'Nathan', age: 30 };
let values = Object.values(user); // [ 'Nathan', 30 ]
let mapResult = values.map(item => item + '101');
console.log(mapResult); // [ 'Nathan', '30101' ]
The same applies when you want to create an array from both keys and values. However, note that since you will have a two dimensional array, you need to specify value you wish to manipulate with the index notation.
The array keys will be at index 0, while the values at index 1. In the following example, the keys will have ‘101’ added to it, while the values will have ‘102’ added:
let user = { name: 'Nathan', age: 30 };
let entries = Object.entries(user);
// [ [ 'name', 'Nathan' ], [ 'age', 30 ] ]
let mapResult = entries.map(item => [item[0] + '101', item[1] + '102']);
console.log(mapResult);
// [ [ 'name101', 'Nathan102' ], [ 'age101', '30102' ] ]
Here you can see that ’name’ becomes ’name101’ while ‘Nathan’ becomes ‘Nathan102’, and so on.
And that’s how you convert an object into an array to use it with the map()
function.
I hope this tutorial is useful and happy coding! 👋